JavaScript: Create a new string with the results of calling a provided function on every character in the calling string
JavaScript fundamental (ES6 Syntax): Exercise-83 with Solution
Map Characters of String to New String
Write a JavaScript program to create an updated string with the results of calling a provided function on every character in the called string.
- Use String.prototype.split('') and Array.prototype.map() to call the provided function, fn, for each character in str.
- Use Array.prototype.join('') to recombine the array of characters into a string.
- The callback function, fn, takes three arguments (the current character, the index of the current character and the string mapString was called upon).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'mapString' to map each character of a string to a new character using a given function.
const mapString = (str, fn) =>
// Split the string into an array of characters, apply the function to each character along with its index, and join the resulting characters back into a string.
str.split('').map((c, i) => fn(c, i, str)).join('');
// Example usage:
console.log(mapString('Javascript exercises', c => c.toUpperCase())); // Outputs: "JAVASCRIPT EXERCISES"
Output:
JAVASCRIPT EXERCISES
Visual Presentation:
Flowchart:
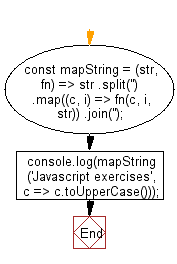
Live Demo:
See the Pen javascript-basic-exercise-83-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to map the values of an array to an object using a function, where the key-value pairs consist of the original value as the key and the mapped value.
Next: Write a JavaScript program to create an object with the same keys as the provided object and values generated by running the provided function for each value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-83.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics