JavaScript: Create an object with the same keys as the provided object and values generated by running the provided function for each value
JavaScript fundamental (ES6 Syntax): Exercise-84 with Solution
Generate Object Values by Function
Write a JavaScript program to create an object with the same keys as the provided object. It will also generate values generated by running the provided function for each value.
Maps the values of an object using the provided function, generating a new object with the same keys.
- Use Object.keys() to iterate over the object's keys.
- Use Array.prototype.reduce() to create a new object with the same keys and mapped values using fn.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'mapValues' to map each value of an object to a new value using a given function.
const mapValues = (obj, fn) =>
// Reduce the object's keys, apply the function to each value along with its key, and store the resulting values in a new object.
Object.keys(obj).reduce((acc, k) => {
acc[k] = fn(obj[k], k, obj);
return acc;
}, {});
// Example usage:
const users = {
fred: { user: 'fred', age: 40 },
pebbles: { user: 'pebbles', age: 1 }
};
console.log(mapValues(users, u => u.age)); // Outputs: { fred: 40, pebbles: 1 }
Output:
{"fred":40,"pebbles":1}
Flowchart:
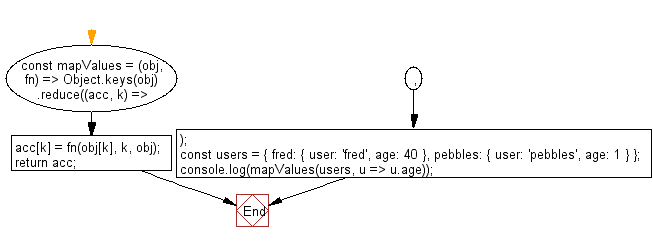
Live Demo:
See the Pen javascript-basic-exercise-84-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a new object with the same keys as an existing object, but with values generated by a function.
- Write a JavaScript function that iterates over an object’s keys and replaces each value with the result of a callback function.
- Write a JavaScript program that transforms an object’s values by applying a provided function and returns the updated object.
Go to:
PREV : Map Characters of String to New String.
NEXT : Mask Characters Except Last Few.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.