JavaScript: Replace all but the last number of characters with the specified mask character
JavaScript fundamental (ES6 Syntax): Exercise-85 with Solution
Mask Characters Except Last Few
Write a JavaScript program to replace all but the last number of characters with the specified mask character.
- Use String.prototype.slice() to grab the portion of the characters that will remain unmasked.
- Use String.padStart() to fill the beginning of the string with the mask character up to the original length.
- If num is negative, the unmasked characters will be at the start of the string.
- Omit the second argument, num, to keep a default of 4 characters unmasked.
- Omit the third argument, mask, to use a default character of '*' for the mask.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'mask' to mask part of a credit card number.
const mask = (cc, num = 4, mask = '*') =>
// Convert the credit card number to a string, mask all but the last 'num' digits, and return the masked number.
('' + cc).slice(0, -num).replace(/./g, mask) + ('' + cc).slice(-num);
// Example usage:
console.log(mask(1234567890));
console.log(mask(1234567890, 3));
console.log(mask(1234567890, -4, '$'));
Output:
******7890 *******890 $$$$567890
Visual Presentation:
Flowchart:
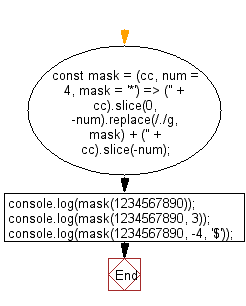
Live Demo:
See the Pen javascript-basic-exercise-85-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that masks all characters in a string except for the last four using a specified mask character.
- Write a JavaScript function that replaces all but the final n characters of a string with asterisks.
- Write a JavaScript program that takes a sensitive string and returns a masked version that reveals only the last few characters.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an object with the same keys as the provided object and values generated by running the provided function for each value.
Next: Write a JavaScript program to get the maximum value of an array, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.