JavaScript: Get the maximum value of an array, after mapping each element to a value using the provided function
JavaScript fundamental (ES6 Syntax): Exercise-86 with Solution
Write a JavaScript program to get the maximum value of an array, after mapping each element to a value using the provided function.
- Use Array.prototype.map() to map each element to the value returned by fn.
- Use Math.max() to get the maximum value.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'maxBy' to find the maximum value in an array based on a given function.
const maxBy = (arr, fn) => Math.max(...arr.map(typeof fn === 'function' ? fn : val => val[fn]));
// Example usage:
console.log(maxBy([{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }], o => o.n)); // Outputs: 8 (maximum value of 'n')
console.log(maxBy([{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }], 'n')); // Outputs: 8 (maximum value of 'n')
Output:
8 8
Visual Presentation:
Flowchart:
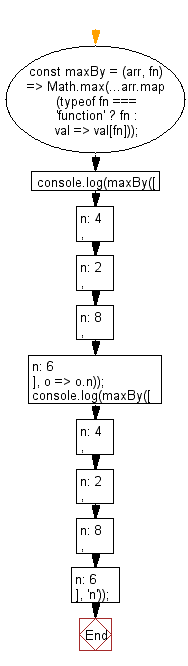
Live Demo:
See the Pen javascript-basic-exercise-86-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to replace all but the last number of characters with the specified mask character.
Next: Write a JavaScript program to get the n maximum elements from the provided array. If n is greater than or equal to the provided array's length, then return the original array(sorted in descending order).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics