JavaScript: Concatenate two strings except their first character
JavaScript Basic: Exercise-61 with Solution
Concatenate Two Strings Without First Character
Write a JavaScript program to concatenate two strings except for their first character.
This JavaScript program concatenates two input strings by omitting their first character from each string before concatenation. The length of both input strings should be at least 2.
Visual Presentation:
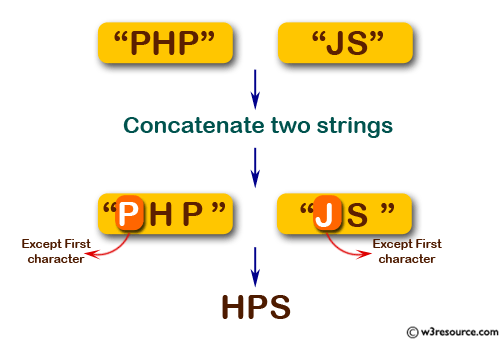
Sample Solution:
JavaScript Code:
// Define a function named concatenate with parameters str1 and str2
function concatenate(str1, str2) {
// Modify str1 to exclude the first character
str1 = str1.substring(1, str1.length);
// Modify str2 to exclude the first character
str2 = str2.substring(1, str2.length);
// Return the concatenation of modified str1 and str2
return str1 + str2;
}
// Call the function with sample arguments and log the results to the console
console.log(concatenate("PHP", "JS"));
console.log(concatenate("A", "B"));
console.log(concatenate("AA", "BB"));
Output:
HPS AB
Live Demo:
See the Pen JavaScript - Concatenate two strings except their first character - basic-ex-61 by w3resource (@w3resource) on CodePen.
Flowchart:
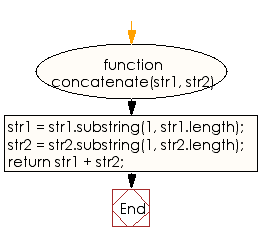
ES6 Version:
// Define a function named concatenate with parameters str1 and str2
const concatenate = (str1, str2) => {
// Use substring to get substrings excluding the first characters
str1 = str1.substring(1);
str2 = str2.substring(1);
// Concatenate the modified substrings
return str1 + str2;
};
// Call the function with sample arguments and log the results to the console
console.log(concatenate("PHP", "JS"));
console.log(concatenate("A", "B"));
console.log(concatenate("AA", "BB"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that concatenates two input strings after removing their first character, ensuring both strings have at least one character.
- Write a JavaScript function that validates the length of two strings and returns their concatenation without the initial characters.
- Write a JavaScript program that safely concatenates two strings, removing the first character of each and handling empty string cases.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string without the first and last character of a given string.
Next: JavaScript program to move last three character to the start of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.