JavaScript: Calculate the area and perimeter of a circle
JavaScript Object: Exercise-9 with Solution
Circle Area & Perimeter
Write a JavaScript program to calculate circle area and perimeter.
Create two methods to calculate the area and perimeter. The radius of the circle will be supplied by the user.
JavaScript: Area and circumference of a circle
In geometry, the area enclosed by a circle of radius r is πr2. Here the Greek letter π represents a constant, approximately equal to 3.14159, which is equal to the ratio of the circumference of any circle to its diameter.
The circumference of a circle is the linear distance around its edge.
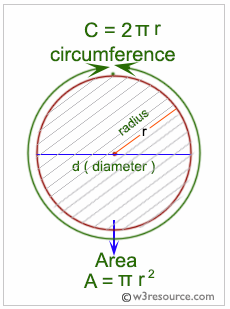
Why is the area of a circle of a circle pi times the square of the radius?
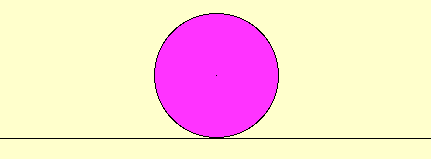
Sample Solution:
JavaScript Code:
function circle(radius)
{
this.radius = radius;
// area method
this.area = function ()
{
return Math.PI * this.radius * this.radius;
};
// perimeter method
this.perimeter = function ()
{
return 2*Math.PI*this.radius;
};
}
var c = new circle(3);
console.log('Area =', c.area().toFixed(2));
console.log('perimeter =', c.perimeter().toFixed(2));
Output:
Area = 28.27 VM1407:17 perimeter = 18.85
Flowchart:
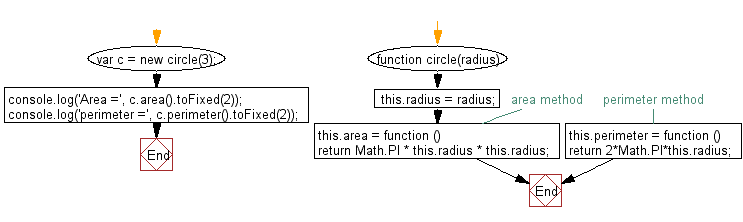
Live Demo:
See the Pen javascript-object-exercise-9 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class that calculates both the area and circumference of a circle given its radius.
- Write a JavaScript function that prompts the user for the radius and then displays the computed area and perimeter.
- Write a JavaScript function that validates the radius input to ensure it is a positive number before calculations.
- Write a JavaScript function that compares the area of a circle to that of a square with an equivalent perimeter.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to create a Clock.
Next: Write a JavaScript program to sort an array of JavaScript objects.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.