JavaScript: Repeat a string a specified times
JavaScript String: Exercise-21 with Solution
Write a JavaScript function to repeat a string for a specified time.
Test Data:
console.log(repeat_string('a', 4));
console.log(repeat_string('a'));
Output:
"aaaa"
"Error in string or count."
Visual Presentation:
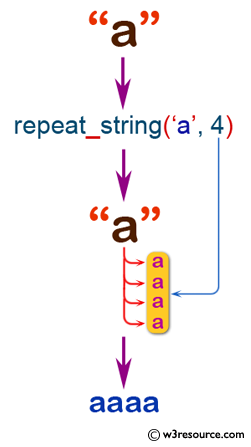
Sample Solution:
JavaScript Code:
// Define a function named repeat_string that takes two parameters: string and count
function repeat_string(string, count)
{
// Check if the string is null, count is negative, count is Infinity, or count is null
if ((string == null) || (count < 0) || (count === Infinity) || (count == null))
{
// If any of the above conditions are true, return an error message
return('Error in string or count.');
}
// Convert count to an integer by bitwise OR operation with 0 (floor count)
count = count | 0; // Floor count.
// Return a new string created by repeating the input string 'count' times
return new Array(count + 1).join(string);
}
// Test the repeat_string function with different arguments and log the results
console.log(repeat_string('a', 4)); // Repeat 'a' 4 times
console.log(repeat_string('a')); // Error due to missing count parameter
console.log(repeat_string('a', -2)); // Error due to negative count
console.log(repeat_string('a', Infinity)); // Error due to count being Infinity
Output:
aaaa Error in string or count. Error in string or count. Error in string or count.
Explanation:
In the exercise above,
- The function "repeat_string(()" takes two parameters: 'string' (the string to repeat) and 'count' (the number of times to repeat the string).
- It checks for various conditions such as if the input string is null, if the count is negative, if the count is Infinity, or if the count is null. If any of these conditions are met, it returns an error message.
- It converts the count to an integer using a bitwise OR operation with 0 to ensure it's a non-negative integer (floor count).
- It then creates a new string by repeating the input string 'count' times using the "Array.join()" method.
- Finally, it tests the "repeat_string()" function with different arguments and logs the results to the console.
Flowchart:
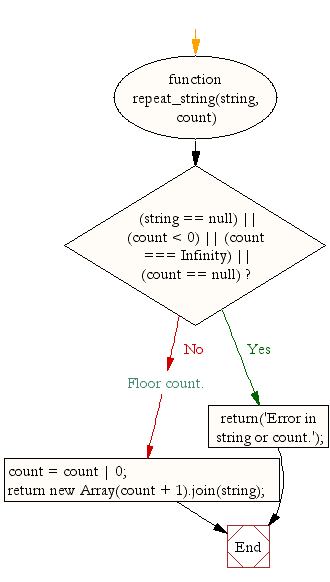
Live Demo:
See the Pen JavaScript - common-editor-exercises by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that can pad (left, right) a string to get to a determined length.
Next: Write a JavaScript function to get a part of string after a specified character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics