PHP Math Exercises: Convert scientific notation to an int and a float
4. Convert Scientific Notation to Int and Float
Write a PHP script to convert scientific notation to an int and a float.
Sample scientific notation : 4.5e3
Sample Solution:
PHP Code:
<?php
$val = '4.5e3'; // Assign a string containing a number in scientific notation ('4.5e3') to the variable $val
$ival = (int) $val; // Convert the string $val to an integer and store it in $ival
$fval = (float) $val; // Convert the string $val to a float and store it in $fval
echo $ival."\n"; // Output the integer value stored in $ival followed by a newline character ("\n")
echo $fval."\n"; // Output the float value stored in $fval followed by a newline character ("\n")
?>
Output:
4 4500
Explanation:
In the exercise above,
- $val = '4.5e3';: This line assigns the string '4.5e3' to the variable $val. This string represents the number 4500 in scientific notation (4.5 * 10^3).
- $ival = (int) $val;: This line converts the string $val to an integer and stores the result in the variable $ival. When casting a string to an integer, PHP converts it to an integer if possible. In this case, it truncates the fractional part and converts '4.5e3' to the integer 4500.
- $fval = (float) $val;: This line converts the string $val to a float and stores the result in the variable $fval. When casting a string to a float, PHP interprets the string as a floating-point number. In this case, it converts '4.5e3' to the float 4500.0.
- echo $ival."\n";: This line outputs the integer value stored in $ival, followed by a newline character ("\n").
- echo $fval."\n";: This line outputs the float value stored in $fval, followed by a newline character ("\n").
Flowchart :
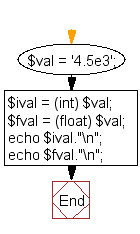
For more Practice: Solve these Related Problems:
- Write a PHP script to parse a scientific notation string and output its integer part (using floor) and its full floating-point value.
- Write a PHP function that converts a scientific notation value to both an integer and a float, handling edge cases like negative exponents.
- Write a PHP program to accept a scientific notation string, extract its mantissa and exponent, and then compute and display the corresponding integer and float values.
- Write a PHP script to convert multiple scientific notation values from an array into integer and float formats and output the results in a formatted table.
Go to:
PREV : Generate Random 11-Character String.
NEXT : Convert Date Format from yyyy-mm-dd to dd-mm-yyyy.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.