Python: Check the sum of three elements from three arrays is equal to a target value
Python Basic - 1: Exercise-11 with Solution
Three Array Sum Combinations
Write a Python program to check the sum of three elements (each from an array) from three arrays is equal to a target value. Print all those three-element combinations.
Sample data:
/*
X = [10, 20, 20, 20]
Y = [10, 20, 30, 40]
Z = [10, 30, 40, 20]
target = 70
*/
Sample Solution:
Python Code:
# Import the 'itertools' module for advanced iteration tools and 'partial' from 'functools'.
import itertools
from functools import partial
# Define three lists and a target sum value.
X = [10, 20, 20, 20]
Y = [10, 20, 30, 40]
Z = [10, 30, 40, 20]
T = 70
# Define a function to check if the sum of a set of numbers equals a given target.
def check_sum_array(N, *nums):
if sum(x for x in nums) == N:
return (True, nums)
else:
return (False, nums)
# Generate the Cartesian product of the three lists.
pro = itertools.product(X, Y, Z)
# Create a partial function using the 'check_sum_array' function and the target sum 'T'.
func = partial(check_sum_array, T)
# Use 'starmap' to apply the partial function to each element in the Cartesian product.
sums = list(itertools.starmap(func, pro))
# Use a set to store unique valid combinations.
result = set()
# Iterate through the sums and print unique valid combinations.
for s in sums:
if s[0] == True and s[1] not in result:
result.add(s[1])
print(result)
Sample Output:
{(10, 20, 40)} {(10, 20, 40), (10, 30, 30)} {(10, 20, 40), (10, 30, 30), (10, 40, 20)} {(10, 20, 40), (10, 30, 30), (20, 10, 40), (10, 40, 20)} {(10, 20, 40), (20, 20, 30), (10, 30, 30), (20, 10, 40), (10, 40, 20)} {(10, 20, 40), (10, 40, 20), (20, 10, 40), (10, 30, 30), (20, 20, 30), (20, 30, 20)} {(10, 20, 40), (10, 40, 20), (20, 10, 40), (20, 40, 10), (10, 30, 30), (20, 20, 30), (20, 30, 20)}
Explanation:
The above Python code tries to find unique combinations of elements from three lists (X, Y, Z) whose sum is equal to a target value T. Here's a brief explanation:
- Import the "itertools" module for advanced iteration and the "partial()" function from the "functools" module.
- Define three lists (X, Y, Z) and a target sum value (T).
- Define a function (check_sum_array) that checks if the sum of a set of numbers equals a given target.
- Generate the Cartesian product of the three lists (X, Y, Z).
- Create a partial function using the "check_sum_array()" function and the target sum T.
- Apply the partial function to each element in the Cartesian product using "starmap".
- Use a set (result) to store unique valid combinations.
- Iterate through the sums and print unique valid combinations.
Flowchart:
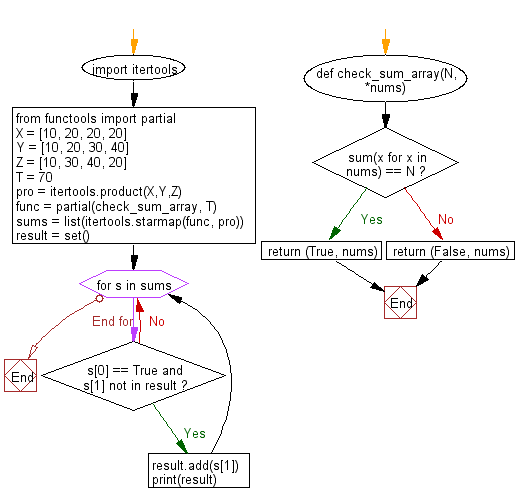
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to display some information about the OS where the script is running.
Next: Write a Python program to create all possible permutations from a given collection of distinct numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics