Python: Compute the amount of the debt in n months
Python Basic - 1: Exercise-36 with Solution
Write a Python program to compute the amount of debt in n months. Each month, the loan adds 5% interest to the $100,000 debt and rounds to the nearest 1,000 above.
Input:
An integer n (0 ≤ n ≤ 100)
Input number of months: 7
Amount of debt: $144000
Sample Solution:
Python Code:
# Function to round a given number to the nearest thousand
def round_n(n):
if n % 1000:
return (1 + n // 1000) * 1000
else:
return n
# Recursive function to compute the debt amount for a given number of months
def compute_debt(n):
if n == 0:
return 100000
return int(round_n(compute_debt(n - 1) * 1.05))
# Print statement to prompt the user to input the number of months
print("Input number of months:")
# Calling the compute_debt function with user input, calculating the debt amount, and printing the result
result = compute_debt(int(input()))
print("Amount of debt: ", "$" + str(result).strip())
Sample Output:
Input number of months: 7 Amount of debt: $144000
Explanation:
The above Python code calculates the amount of debt after a given number of months based on a recursive formula. Here's a brief explanation:
- The "round_n()" function rounds a given number to the nearest thousand.
- The "compute_debt()" function is a recursive function that calculates the debt amount for a given number of months. The base case is when the number of months is 0, and it returns a fixed debt amount of 100,000. Otherwise, it recursively calculates the debt amount for the previous month, multiplies it by 1.05, and rounds the result to the nearest thousand using the "round_n()" function.
- The code prompts the user to input the number of months.
- It calls the "compute_debt()" function with the user input, calculates the debt amount, and prints the result, formatted as a dollar amount.
Flowchart:
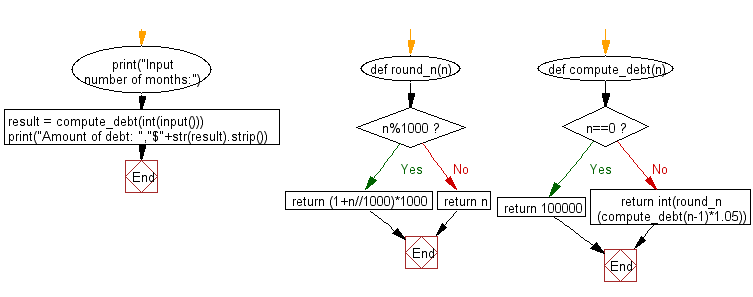
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program which solve the specified equation.
Next: Write a Python program which reads an integer n and find the number of combinations of a,b,c and d (0 ≤ a,b,c,d ≤ 9) where (a + b + c + d) will be equal to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics