Python: Calculate the maximum profit from selling and buying values of stock
Python Basic - 1: Exercise-74 with Solution
Max Profit from Stocks
Write a Python program to calculate the maximum profit from selling and buying values of stock. An array of numbers represent the stock prices in chronological order.
For example, given [8, 10, 7, 5, 7, 15], the function will return 10, since the buying value of the stock is 5 dollars and sell value is 15 dollars.
Sample Solution:
Python Code:
# Define a function to calculate the maximum profit from buying and selling stocks
def buy_and_sell(stock_price):
# Initialize variables to store the maximum profit and current maximum value
max_profit_val, current_max_val = 0, 0
# Iterate through the reversed stock prices
for price in reversed(stock_price):
# Update the current maximum value with the maximum of the current value and the previous maximum
current_max_val = max(current_max_val, price)
# Calculate the potential profit by subtracting the current price from the current maximum value
potential_profit = current_max_val - price
# Update the maximum profit with the maximum of the potential profit and the previous maximum profit
max_profit_val = max(potential_profit, max_profit_val)
# Return the maximum profit
return max_profit_val
# Test the function with different stock price lists and print the results
print(buy_and_sell([8, 10, 7, 5, 7, 15])) # Maximum Profit: 10
print(buy_and_sell([1, 2, 8, 1])) # Maximum Profit: 7
print(buy_and_sell([])) # Maximum Profit: 0
Sample Output:
10 7 0
Explanation:
Here is a breakdown of the above Python code:
- The function "buy_and_sell()" calculates the maximum profit from buying and selling stocks.
- It iterates through the reversed 'stock_price' list, starting from the end.
- For each price, it updates the 'current_max_val' with the maximum of the current value and the previous maximum.
- It calculates the potential profit by subtracting the current price from the 'current_max_val'.
- It updates the 'max_profit_val' with the maximum of the potential profit and the previous maximum profit.
- The function returns the final maximum profit value.
- Test the function with different stock price lists and print the results.
Flowchart:
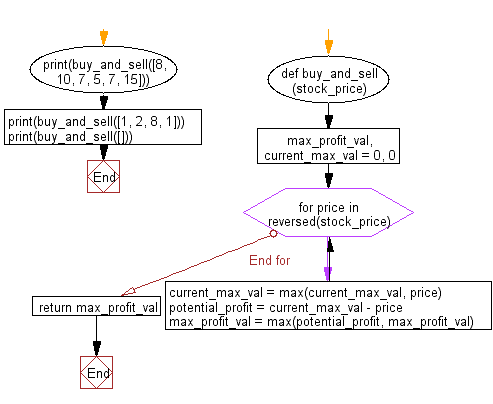
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to remove the duplicate elements of a given array of numbers such that each element appear only once and return the new length of the given array.
Next: Write a Python program to remove all instances of a given value from a given array of integers and find the length of the new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-74.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics