Python: Find the position of the second occurrence of a given string in another given string
Python Basic - 1: Exercise-99 with Solution
Second Occurrence Position
Write a Python program to find the position of the second occurrence of a given string in another given string. If there is no such string return -1.
Sample Solution:
Python Code:
# Define a function named find_string that takes two strings (txt and str1) as arguments.
def find_string(txt, str1):
# Use the find method to locate the second occurrence of str1 in txt.
# The expression txt.find(str1) finds the first occurrence, and adding 1 finds the starting position for searching the second occurrence.
return txt.find(str1, txt.find(str1) + 1)
# Test the function with different strings and print the results.
# Test case 1
print(find_string("The quick brown fox jumps over the lazy dog", "the"))
# Test case 2
print(find_string("the quick brown fox jumps over the lazy dog", "the"))
Sample Output:
-1 31
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "find_string()" that takes two strings (txt and str1) as arguments.
- Find Method:
- The function uses the "find()" method to locate the second occurrence of 'str1' in 'txt'.
- Starting Position for Search:
- The expression txt.find(str1) + 1 finds the starting position for searching the second occurrence of 'str1' in 'txt'.
- Return Statement:
- The function returns the result of the second find operation.
Visual Presentation:
Flowchart:
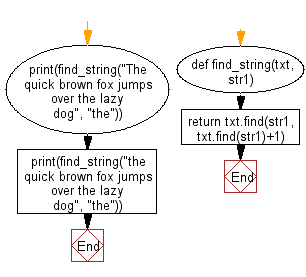
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether a sequence of numbers has an increasing trend or not.
Next: Write a Python program to compute the sum of all items of a given array of integers where each integer is multiplied by its index.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-99.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics