Python: Calculate the average of a given list, after mapping each element to a value using the provided function
Average Mapped Values
Write a Python program to calculate the average of a given list, after mapping each element to a value using the provided function.
- Use map() to map each element to the value returned by fn.
- Use sum() to sum all of the mapped values, divide by len(lst).
- Omit the last argument, fn, to use the default identity function.
Sample Solution:
Python Code:
# Define a function 'average_by' that takes a list 'lst' and an optional function 'fn' as input.
def average_by(lst, fn=lambda x: x):
# Calculate the average of the values in the list 'lst' by performing the following steps:
# 1. Use the 'map' function to apply the function 'fn' to each element of the list 'lst'.
# 2. Use 'sum' to calculate the sum of the mapped values, starting with a floating-point 0.0.
# 3. Divide the sum by the length of the list 'lst' to compute the average.
return sum(map(fn, lst), 0.0) / len(lst)
# Call the 'average_by' function with a list of dictionaries and a lambda function to extract the 'n' key's value.
# Print the result, which is the average of the 'n' values.
print(average_by([{ 'n': 4 }, { 'n': 2 }, { 'n': 8 }, { 'n': 6 }], lambda x: x['n']))
print(average_by([{ 'n': 10 }, { 'n': 20 }, { 'n': -30 }, { 'n': 60 }], lambda x: x['n']))
Sample Output:
5.0 15.0
Flowchart:
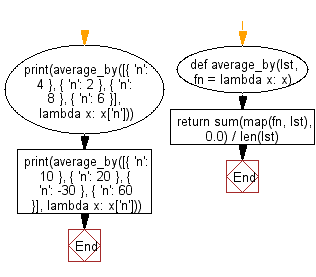
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the average of a list after mapping each string element to its length.
- Write a Python program to compute the average of a list of numbers after normalizing them to a 0-1 scale via a provided function.
- Write a Python program to calculate the average of values obtained by mapping each element to its square root.
- Write a Python program to compute the average of a list after applying a logarithmic transformation to each element.
Go to:
Previous: Write a Python program to convert a given list of dictionaries into a list of values corresponding to the specified key.
Next: Write a Python program to find the value of the first element in the given list that satisfies the provided testing function.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.