Python: Get the largest number from a list
Python List: Exercise-3 with Solution
Write a Python program to get the largest number from a list.
Visual Presentation:
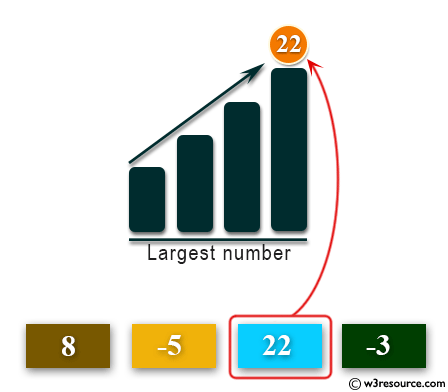
Sample Solution:
Python Code:
# Define a function called max_num_in_list that takes a list 'list' as input
def max_num_in_list(list):
# Initialize a variable 'max' with the first element of the input list as the initial maximum
max = list[0]
# Iterate through each element 'a' in the input list 'list'
for a in list:
# Check if the current element 'a' is greater than the current maximum 'max'
if a > max:
# If 'a' is greater, update the maximum 'max' to 'a'
max = a
# Return the final maximum value in the list
return max
# Call the max_num_in_list function with the list [1, 2, -8, 0] as input and print the result
print(max_num_in_list([1, 2, -8, 0]))
Sample Output:
2
Explanation:
In the above code -
def max_num_in_list( list ): -> This code defines a function called max_num_in_list that takes a single argument list. This function will be used to find the maximum number in the list.
max = list[ 0 ] -> We assume the list is not empty. This line initializes a variable called max to the first element of the list.
for a in list: -> This line starts a loop that will iterate over each element in the list, one at a time. The loop variable 'a' will take on the value of each element in the list during each iteration of the loop.
if a > max: max = a
The above line compares the current element 'a' to the current maximum max. If 'a' is greater than 'max', 'max' is updated to equal 'a'. After iterating through the entire list, 'max' will hold the value of the largest number in the list.
return max -> This line returns the final value of the ‘max’ variable after the loop has finished.
print(max_num_in_list([1, 2, -8, 0])) -> This line calls the ‘max_num_in_list()’ function and passes in the list [1, 2, -8, 0]. Finally print() function prints the resulting maximum value.
Flowchart:
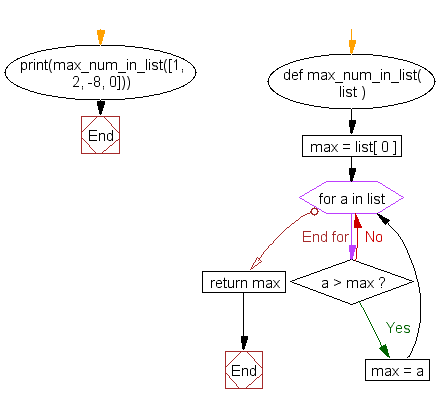
Python Code Editor:
Previous: Write a Python program to multiply all the items in a list.
Next: Write a Python program to get the smallest number from a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics