Python Math: Find the sum of the specified decimal numbers and display decimal numbers in sorted order
37. Sum and Sort Decimals
Write a Python program to find the sum of the following decimal numbers and display the numbers in sorted order.
Sample Solution :
Python Code :
from decimal import *
data = list(map(Decimal, '2.45 2.69 2.45 3.45 2.00 0.04 7.25'.split()))
print("Sum: ", sum(data))
print("Sorted order: ", sorted(data))
Sample Output:
Sum: 20.33 Sorted order: [Decimal('0.04'), Decimal('2.00'), Decimal('2.45'), Decimal('2.45'), Decimal('2.69'), Decimal(' 3.45'), Decimal('7.25')]
Pictorial Presentation:
Flowchart:
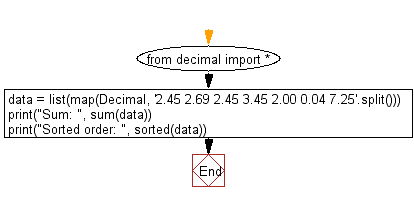
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the sum of a list of Decimal numbers and then print the list sorted in ascending order.
- Write a Python function that takes a list of Decimal values, computes their total sum, and returns both the sum and the sorted list.
- Write a Python script to read several Decimal numbers, calculate their sum, and then display the sorted sequence along with the sum.
- Write a Python program to compute the sum of Decimal numbers from a list and then print the sorted list along with the sum, ensuring high precision.
Go to:
Previous: Write a Python program to find the maximum and minimum numbers from the specified decimal numbers.
Next: Write a Python program to get the square root and exponential of a given decimal number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.