Python Math: Find perfect squares between two given numbers
78. Perfect Squares Finder
Write a Python program to find perfect squares between two given numbers.
Sample Solution:
Python Code:
def squares(a, b):
lists=[]
# Traverse through all numbers
for i in range (a,b+1):
j = 1;
while j*j <= i:
if j*j == i:
lists.append(i)
j = j+1
i = i+1
return lists
print(squares(1, 30))
Sample Output:
[1, 4, 9, 16, 25]
Flowchart:
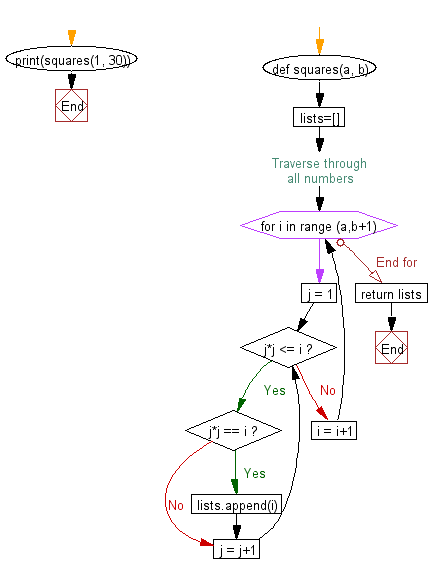
For more Practice: Solve these Related Problems:
- Write a Python program to find and print all perfect squares between two specified integers.
- Write a Python function that returns a list of perfect squares within a given range and prints the count of such numbers.
- Write a Python script to generate perfect squares up to 100 and then print them in reverse order.
- Write a Python program to prompt the user for a range and output all perfect squares within that range, formatted in columns.
Go to:
Previous: Write a Python program to convert RGB color to HSV color.
Next: Write a Python program to compute Euclidean distance.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.