Python Exercises: Rearrange the digits of a number
93. Rearrange Digits for Extremes
Write a Python program that takes an integer and rearranges the digits to create two maximum and minimum numbers.
Sample Solution-1:
Python Code:
def test(n):
temp = []
for e in str(n):
temp.append(e)
max_num = "".join(sorted(temp)[::-1])
min_num = "".join(sorted(temp))
return int(max_num), int(min_num)
n = 1254
print("Original number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum numbers:")
print("Maximum and Minimum Numbers:",test(n))
n = 6
print("\nOriginal number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum numbers:")
print("Maximum and Minimum Numbers:",test(n))
n = 1000
print("\nOriginal number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum numbers:")
print("Maximum and Minimum Numbers:",test(n))
Sample Output:
Original number: 1254 Rearrange the digits of the said number to get Maximum and Minimum numbers: Maximum and Minimum Numbers: (5421, 1245) Original number: 6 Rearrange the digits of the said number to get Maximum and Minimum numbers: Maximum and Minimum Numbers: (6, 6) Original number: 1000 Rearrange the digits of the said number to get Maximum and Minimum numbers: Maximum and Minimum Numbers: (1000, 1)
Flowchart:
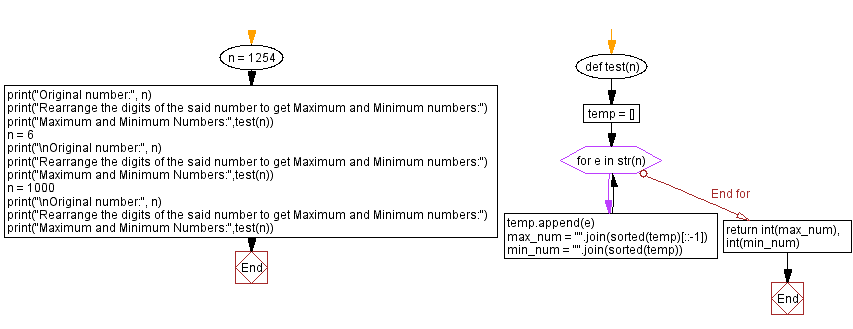
Sample Solution-2:
Python Code:
def test(n):
temp = ''.join(sorted(str(n)))
return int(temp[::-1]), int(temp)
n = 1254
print("Original number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum Numbers:")
print("Maximum and Minimum Numbers:",test(n))
n = 6
print("\nOriginal number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum Numbers:")
print("Maximum and Minimum Numbers:",test(n))
n = 1000
print("\nOriginal number:", n)
print("Rearrange the digits of the said number to get Maximum and Minimum Numbers:")
print("Maximum and Minimum Numbers:",test(n))
Sample Output:
Original number: 1254 Rearrange the digits of the said number to get Maximum and Minimum Numbers: Maximum and Minimum Numbers: (5421, 1245) Original number: 6 Rearrange the digits of the said number to get Maximum and Minimum Numbers: Maximum and Minimum Numbers: (6, 6) Original number: 1000 Rearrange the digits of the said number to get Maximum and Minimum Numbers: Maximum and Minimum Numbers: (1000, 1)
Flowchart:
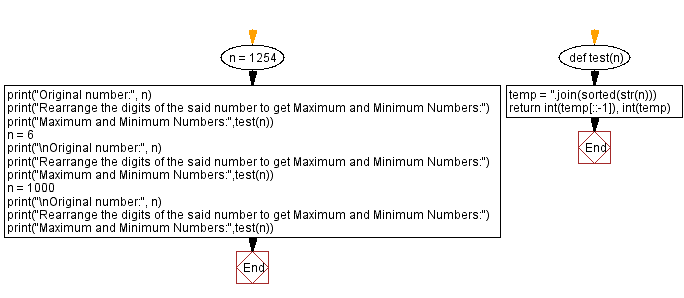
For more Practice: Solve these Related Problems:
- Write a Python program to rearrange the digits of an integer to form the maximum and minimum numbers possible, and then print both values.
- Write a Python function that takes an integer and returns a tuple containing the maximum and minimum numbers that can be formed from its digits.
- Write a Python script to prompt the user for a number, compute the maximum and minimum numbers from its digits, and then print them.
- Write a Python program to compare the sum of the maximum and minimum numbers formed from an integer's digits with the original number, and print the results.
Go to:
Previous Python Exercise: Absolute difference between two consecutive digits.
Next Python Exercise: Sum of all prime numbers in a list of integers.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.