NumPy: Compute the outer product of two given vectors
2. Outer Product of Vectors
Write a NumPy program to compute the outer product of two given vectors.
NumPy: Outer product of two vectors
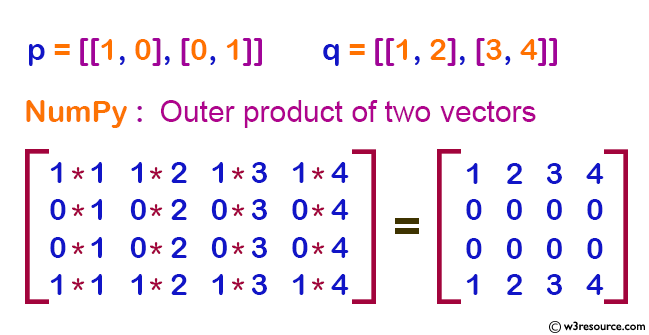
Sample Solution :
Python Code :
import numpy as np
# Define two 2x2 matrices 'p' and 'q'
p = [[1, 0], [0, 1]]
q = [[1, 2], [3, 4]]
# Display the original matrices 'p' and 'q'
print("Original matrices:")
print(p)
print(q)
# Compute the outer product of matrices 'p' and 'q' using np.outer
result = np.outer(p, q)
# Display the outer product of the matrices
print("Outer product of the said two vectors:")
print(result)
Sample Output:
original matrix: [[1, 0], [0, 1]] [[1, 2], [3, 4]] Outer product of the said two vectors: [[1 2 3 4] [0 0 0 0] [0 0 0 0] [1 2 3 4]]
Explanation:
p = [[1, 0], [0, 1]]
q = [[1, 2], [3, 4]]
At first two 2x2 matrixes p and q have been declared.
result = np.outer(p, q) This line calculates the outer product of p and q. The outer product is calculated by taking the Cartesian product of the elements in p and q, and multiplying them together.
[[1*1, 1*2, 1*3, 1*4],
[0*1, 0*2, 0*3, 0*4],
[0*1, 0*2, 0*3, 0*4],
[1*1, 1*2, 1*3, 1*4]]
Finally print() prints the resulting 4x4 matrix.
For more Practice: Solve these Related Problems:
- Compute the outer product of two 1D arrays using np.outer and verify the dimensions of the result.
- Implement the outer product manually by reshaping one vector to a column and the other to a row and multiplying them.
- Compare the result of np.outer with a custom function that uses broadcasting to form the outer product.
- Extend the outer product computation to handle vectors of different lengths and analyze the resulting shape.
Go to:
PREV : Matrix Multiplication
NEXT : Cross Product of Vectors
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.