Python: Convert a given string to Snake case
Python String: Exercise-97 with Solution
Convert string to snake_case.
From Wikipedia,
Snake case (stylized as snake_case) refers to the style of writing in which each space is replaced by an underscore (_) character, and the first letter of each word written in lowercase. It is a commonly used naming convention in computing, for example for variable and subroutine names, and for filenames. One study has found that readers can recognize snake case values more quickly than camel case.
Write a Python program to convert a given string to Snake case.
- Use re.sub() to match all words in the string, str.lower() to lowercase them.
- Use re.sub() to replace any - characters with spaces.
- Finally, use str.join() to combine all words using - as the separator.
Sample Solution:
Python Code:
# Import the 'sub' function from the 're' module for regular expression substitution
from re import sub
# Define a function to convert a string to snake case
def snake_case(s):
# Replace hyphens with spaces, then apply regular expression substitutions for title case conversion
# and add an underscore between words, finally convert the result to lowercase
return '_'.join(
sub('([A-Z][a-z]+)', r' \1',
sub('([A-Z]+)', r' \1',
s.replace('-', ' '))).split()).lower()
# Test the function with different input strings and print the results
print(snake_case('JavaScript'))
print(snake_case('Foo-Bar'))
print(snake_case('foo_bar'))
print(snake_case('--foo.bar'))
print(snake_case('Foo-BAR'))
print(snake_case('fooBAR'))
print(snake_case('foo bar'))
Sample Output:
java_script foo_bar foo_bar foo.bar foo_bar foo_bar foo_bar
Flowchart:
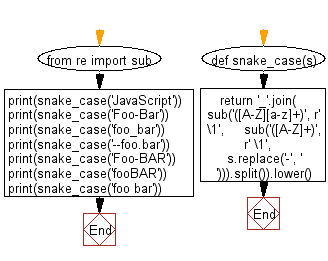
Python Code Editor:
Previous: Write a Python program to convert a given string to camelcase.
Next: Write a Python program to decapitalize the first letter of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/string/python-data-type-string-exercise-97.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics