Create a Python Tkinter timer application
Python tkinter Basic: Exercise-20 with Solution
Write a Python program that implements a Tkinter-based timer application that counts down from a specified time when started.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
# Function to start the timer
def start_timer():
global remaining_time
try:
minutes = int(minutes_entry.get())
seconds = int(seconds_entry.get())
remaining_time = minutes * 60 + seconds
update_timer()
start_button.config(state="disabled")
stop_button.config(state="active")
except ValueError:
pass
# Function to update the timer
def update_timer():
global remaining_time
if remaining_time > 0:
minutes = remaining_time // 60
seconds = remaining_time % 60
timer_label.config(text=f"{minutes:02d}:{seconds:02d}")
remaining_time -= 1
parent.after(1000, update_timer) # Update every 1000 milliseconds (1 second)
else:
timer_label.config(text="00:00")
start_button.config(state="active")
stop_button.config(state="disabled")
# Function to stop the timer
def stop_timer():
global remaining_time
remaining_time = 0
timer_label.config(text="00:00")
start_button.config(state="active")
stop_button.config(state="disabled")
# Create the main window
parent = tk.Tk()
parent.title("Timer Application")
# Create and place input fields for minutes and seconds
minutes_label = tk.Label(parent, text="Minutes:")
minutes_label.grid(row=0, column=0)
minutes_entry = tk.Entry(parent)
minutes_entry.grid(row=0, column=1)
minutes_entry.insert(0, "0")
seconds_label = tk.Label(parent, text="Seconds:")
seconds_label.grid(row=1, column=0)
seconds_entry = tk.Entry(parent)
seconds_entry.grid(row=1, column=1)
seconds_entry.insert(0, "0")
# Create and place timer label
timer_label = tk.Label(parent, text="00:00", font=("Helvetica", 48))
timer_label.grid(row=2, columnspan=2)
# Create and place start and stop buttons
start_button = ttk.Button(parent, text="Start", command=start_timer)
start_button.grid(row=3, column=0)
stop_button = ttk.Button(parent, text="Stop", command=stop_timer, state="disabled")
stop_button.grid(row=3, column=1)
# Initialize the remaining time
remaining_time = 0
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- First we create a Tkinter window with input fields for minutes and seconds, a timer label, and start and stop buttons.
- When the user enters the desired time in minutes and seconds and clicks the "Start" button, the "start_timer()" function is called. It calculates the total remaining time in seconds and updates the timer using the "update_timer()" function.
- By subtracting one second from the remaining time, "update_timer()" updates the timer label every second and schedules itself to run again after 1000 milliseconds (1 second). The timer stops when the remaining time reaches zero, and the "Start" button is reactivated.
- The "Stop" button can stop the timer at any time.
Sample Output:
Flowchart:
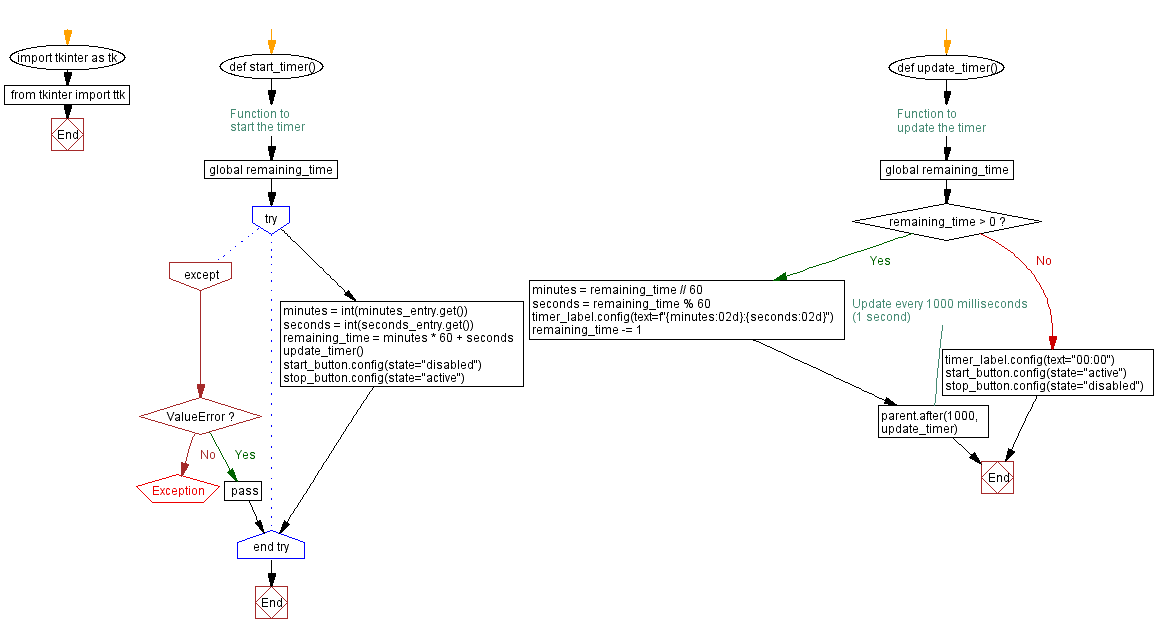
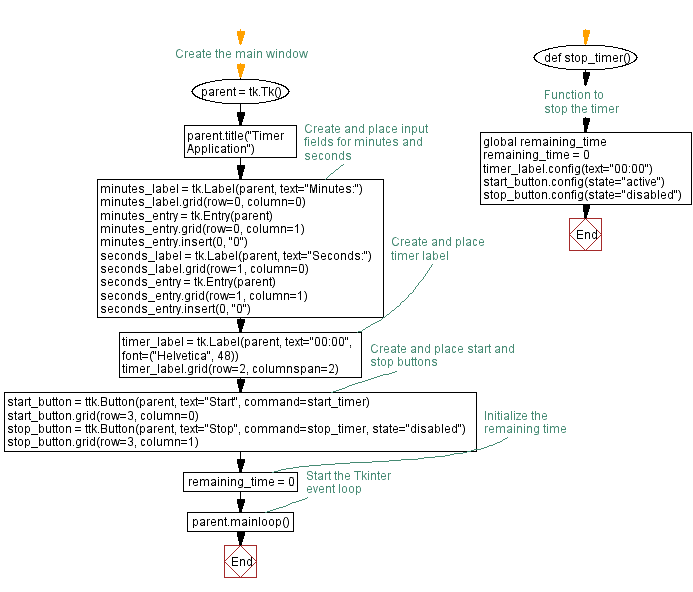
Python Code Editor:
Previous: Create a Python Tkinter application with color picker.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/tkinter/python-tkinter-basic-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics