Python Tkinter GUI program: Adding labels and buttons
Write a Python GUI program that adds labels and buttons to the Tkinter window.
Sample Solution:
Python Code:
import tkinter as tk
# Create the main window
parent = tk.Tk()
parent.title("Button Click Event Handling")
# Create a label widget
label = tk.Label(parent, text="Click the button and check the label text:")
label.pack()
# Function to handle button click event
def on_button_click():
label.config(text="Button Clicked!")
# Create a button widget
button = tk.Button(parent, text="Click Me", command=on_button_click)
button.pack()
# Start the Tkinter event loop
parent.mainloop()
In the exercise above the code creates a simple “Tkinter” window with a label that says " Tkinter basic exercises!" and a button that says " Click here!" When you click the button, the label text will change to "Button Clicked!".
Sample Output:
Flowchart:
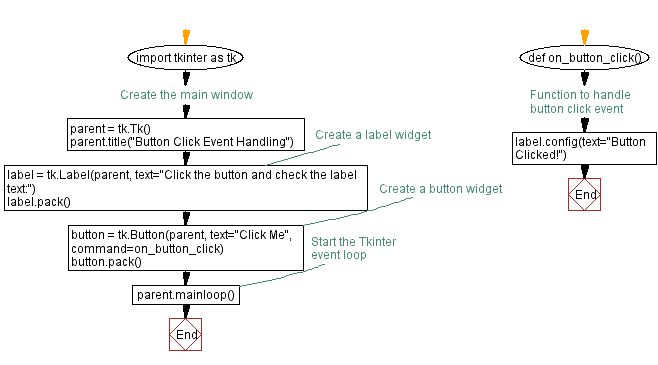
Go to:
Previous: Adding labels and buttons.
Next: Python Tkinter event handling: Button clicks.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.