Python Math: Create a range for floating numbers
72. Floating Point Range Creator
Write a Python program to create a range for floating numbers.
Sample Solution:
Python Code:
#https://gist.github.com/axelpale/3e780ebdde4d99cbb69ffe8b1eada92c
def frange(x, y, jump=1.0):
i = 0.0
x = float(x) # Prevent yielding integers.
y = float(y) # Comparison converts y to float every time otherwise.
x0 = x
epsilon = jump / 2.0
yield x # yield always first value
while x + epsilon < y:
i += 1.0
x = x0 + i * jump
yield x
print(list(frange(0.0, 1.0, 0.1)))
Sample Output:
[0.0, 0.1, 0.2, 0.30000000000000004, 0.4, 0.5, 0.6000000000000001, 0.7000000000000001, 0.8, 0.9, 1.0]
Flowchart:
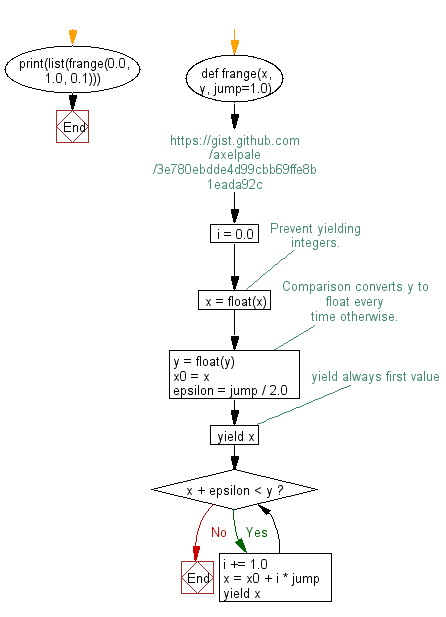
For more Practice: Solve these Related Problems:
- Write a Python program to create a list of floating-point numbers from 0.0 to 1.0 with a step of 0.1 using a while loop.
- Write a Python function that generates a range of floating-point numbers with a specified start, stop, and step, and returns the list.
- Write a Python script to compare a custom floating point range generator with numpy.arange() and print both outputs.
- Write a Python program to generate and print a list of floats from 0 to 1 inclusive, ensuring proper floating-point precision.
Go to:
Previous: Write a Python program to reverse a range.
Next: Write a Python program to generate (given an integer n) a square matrix filled with elements from 1 to n2 in spiral order.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.