Retry tests and dynamically generating tests
Retry tests
You can decide to retry tests that failed up to a certain number of times. The retry feature is designed to handle end-to-end tests (functional tests/Selenium) where resources cannot be easily mocked/stubbed. It is not recommended to use this feature for unit tests.
The retry feature does re-run beforeEach/afterEach hooks but it doesn't run before/after hooks.
Note: The example below was written using Selenium webdriver (which will overwrite global Mocha hooks for promise chain).
describe('retries', function() {
// this will retry all tests in this suite up to 4 times
this.retries(4);
beforeEach(function() {
browser.get('http://www.yahoo.com');
});
it('has to succeed on the 4th try', function() {
// Specify that this test is to only retry up to 3 times
this.retries(3);
expect($('.foo').isDisplayed()).to.eventually.be.true;
});
});
Let us consider another example:
describe('retries', function() {
// this will retry all tests in this suite up to 4 times
this.retries(4);
beforeEach(function() {
browser.get('http://www.w3resource.com');
});
it('should succeed on the 2nd try', function() {
// Specify this test to only retry up to 1 times
this.retries(1);
expect($('.foo').isDisplayed()).to.eventually.be.true;
});
});
As can be seen from these two examples, the number of times that a test reruns is dynamic and depends on you.
Dynamically generating tests
Because of Mocha's use of Function.prototype.call and function expressions in defining suites and test cases, it is straightforward to generate your tests dynamically. You don't need special syntax - you can use plain ol' JavaScript can be used to achieve functionality similar to "parameterized" tests, which you might have seen in other frameworks.
Consider the following example:
var assert = require('chai').assert;
function add() {
return Array.prototype.slice.call(arguments).reduce(function(prev, curr) {
return prev + curr;
}, 0);
}
describe('add()', function() {
var tests = [
{args: [1, 2], expected: 3},
{args: [1, 2, 3], expected: 6},
{args: [1, 2, 3, 4], expected: 10}
];
tests.forEach(function(test) {
it('will correctly add ' + test.args.length + ' args', function() {
var res = add.apply(null, test.args);
assert.equal(res, test.expected);
});
});
});
The code above will produce a suite that has three specs:
mocha
add()
correctly adds 2 args
correctly adds 3 args
correctly adds 4 args
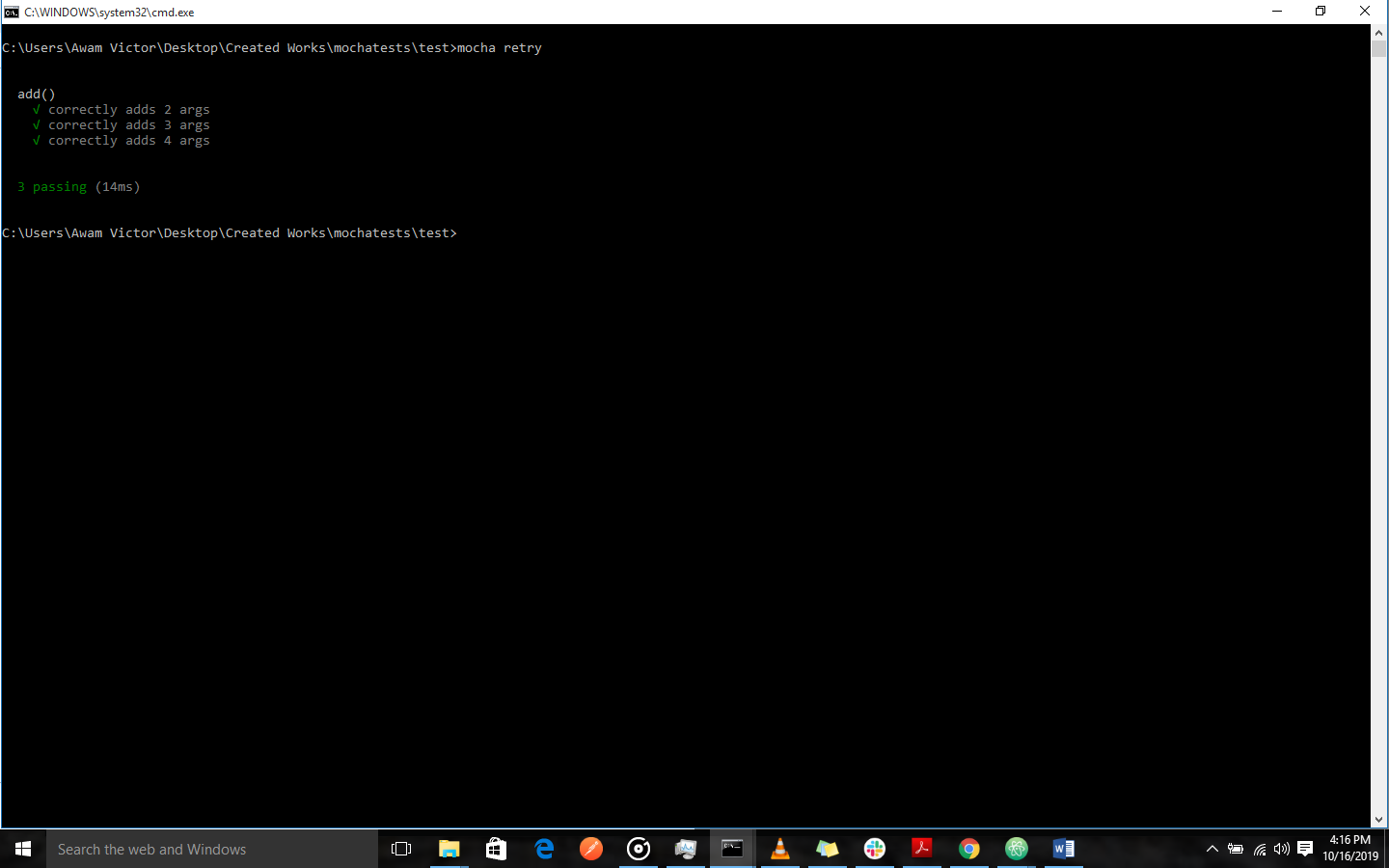
Figure showing the result of a dynamically generated test.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics