Python List append() method
append() method
The append() method is used to add an item to the end of a list.
The append() method is a simple yet powerful way to add elements to the end of a list. It supports adding various data types, including numbers, strings, lists, and more. Understanding its syntax and usage can help you manage and manipulate lists more effectively in Python.
Visual Explanation:
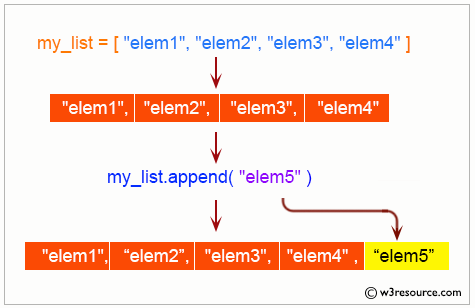
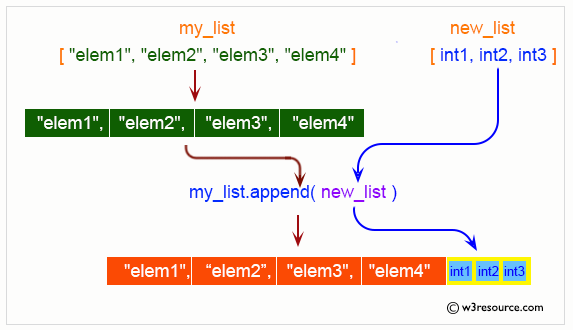
Syntax:
list.append(item)
Parameter:
- item: The item (number, string, list, etc.) to be added to the end of the list.
Equivalent Command of append() method:
The append() method can be equivalently represented by the following command:
a[len(a):] = [x]
Example 1: Adding an Element to a List
# colors list
colors = ['Red', 'Green', 'Black']
print("Original list:", colors)
# Add 'White' to the list
colors.append('White')
print("New list:", colors)
Output:
Original list: ['Red', 'Green', 'Black'] Add 'White' to the list: New list: ['Red', 'Green', 'Black', 'White']
Example 2: Adding a List to a List
# colors list
colors = ['Red', 'Green', 'Black']
nums = [1, 2, 3]
print("Original lists:")
print(colors)
print(nums)
# Append nums to colors
colors.append(nums)
print("New list:", colors)
Output:
Original lists: ['Red', 'Green', 'Black'] [1, 2, 3] Append nums to colors: New list: ['Red', 'Green', 'Black', [1, 2, 3]]
Example 3: Adding Different Data Types
# Mixed list
items = ['apple', 42, 3.14]
print("Original list:", items)
# Add a boolean value
items.append(True)
print("List after adding a boolean:", items)
# Add a dictionary
items.append({"key": "value"})
print("List after adding a dictionary:", items)
Output:
Original list: ['apple', 42, 3.14] List after adding a boolean: ['apple', 42, 3.14, True] List after adding a dictionary: ['apple', 42, 3.14, True, {'key': 'value'}]
Example 4: Building a List Dynamically
# Empty list to hold squares
squares = []
# Add squares of numbers from 1 to 5
for i in range(1, 6):
squares.append(i**2)
print("List of squares:", squares)
Output:
List of squares: [1, 4, 9, 16, 25]
Example 5: Using append() in a Function
# Function to add elements to a list
def add_elements(lst, elements):
for elem in elements:
lst.append(elem)
return lst
# Original list
original_list = ['Python', 'is']
# Elements to add
new_elements = ['fun', 'and', 'powerful']
# Add elements using the function
updated_list = add_elements(original_list, new_elements)
print("Updated list:", updated_list)
Output:
Updated list: ['Python', 'is', 'fun', 'and', 'powerful']
Python append() Method FAQ
1. What does the append() method do in Python?
The append() method adds a single item to the end of a list.
2. Can append() be used to add multiple items to a list at once?
No, append() can only add one item at a time to the end of the list.
3. Does append() create a new list or modify an existing one?
The append() method modifies the existing list in place and does not create a new list.
4. Can the item added by append() be of any data type?
Yes, the item can be of any data type, including numbers, strings, lists, and other objects.
5. What happens if you use append() to add a list to another list?
The entire list is added as a single element to the end of the target list.
6. Is append() an efficient way to add elements to a list?
Yes, append() is efficient as it adds an item to the end of the list in constant time, O(1).
7. Can append() be used inside a loop?
Yes, append() is often used inside loops to build or modify lists dynamically.
8. Does append() return any value?
No, append() does not return a value; it modifies the list in place and returns None.
9. Is there a limit to the number of items you can append to a list?
The only limit is available memory; otherwise, you can append as many items as needed.
10. How does append() differ from extend()?
append() adds its argument as a single element to the end of the list, whereas extend() iterates over its argument adding each element to the list, extending the list.
Python Code Editor:
Previous: Python List Methods home page
Next: Python List clear() Method.
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics