Python List clear() Method
clear() Method
The clear() method is used to remove all items from a given list.
The clear() method is a convenient way to empty a list. Understanding the different methods to clear a list, such as del statement and *= 0, as well as their impact on list references, can enhance your ability to manage lists effectively in Python.
Visual Explanation:
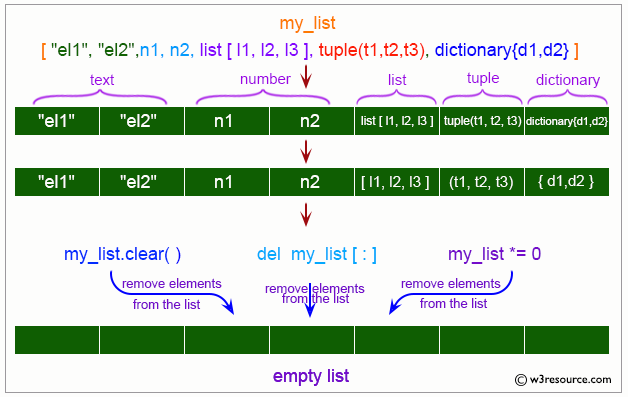
Syntax:
list.clear()
Parameter:The clear() method does not take any parameters.
Equivalent Command of clear() method:
The clear() method can be equivalently represented by:
del a[:]
Example 1: Using the clear() Method
# colors list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Clear the list
colors.clear()
print("Clearing the said list:")
print("New list:", colors)
Output:
Original list: ['Red', 'Green', 'Black'] Clearing the said list: New list: []
Example 2: Removing Miscellaneous Items from a List
# mixed list
items = ["Red", 'd', {1, 2, 3}, [10.10, 45.2, 500]]
print("Original list:")
print(items)
# Clear the list
items.clear()
print("Clearing the said list:")
print("New list:", items)
Output:
Original list: ['Red', 'd', {1, 2, 3}, [10.1, 45.2, 500]] Clearing the said list: New list: []
Clearing List Items Without clear() Method
You can clear a list using alternative methods such as the del statement or list slicing.
Example 3: Using the del Statement
# colors list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Clear the list using del statement
del colors[:]
print("Clearing the said list using del statement:")
print("New list:", colors)
Output:
Original list: ['Red', 'Green', 'Black'] Clearing the said list using del statement: New list: []
Example 4: Using *= 0
# colors list
colors = ['Red', 'Green', 'Black']
print("Original list:")
print(colors)
# Clear the list using *= 0
colors *= 0
print("Clearing the said list using *= 0:")
print("New list:", colors)
Output:
Original list: ['Red', 'Green', 'Black'] Clearing the said list using *= 0: New list: []
Understanding List References
Clearing a list with the clear() method or other methods does not affect other references to the original list.
Example 5: Impact on References
# colors list
colors = ['Red', 'Green', 'Black']
temp = colors
# Clear the list using clear() method
colors.clear()
print("Cleared list:", colors)
print("Reference to original list remains unchanged:", temp)
Output:
Cleared list: [] Reference to original list remains unchanged: []
Note: When we assign temp = colors, both temp and colors point to the same list object in memory. They are two references to the same underlying list. Therefore, any modification to the list through one reference affects the other reference since both point to the same object.
Python clear() Method FAQ
1. What does the clear() method do?
The clear() method removes all items from a list, making it empty.
2. Does the clear() method delete the list itself?
No, the clear() method only removes the list items, not the list object itself.
3. Is the clear() method part of the standard Python library?
Yes, the clear() method is a built-in method for Python lists.
4. Can clear() be used on any other data structures in Python?
No, the clear() method is specific to lists. Other data structures like sets and dictionaries have their own clear() methods.
5. Does the clear() method return any value?
No, the clear() method does not return a value; it modifies the list in place.
6. Is using clear() the same as reassigning the list to an empty list?
Functionally similar, but reassigning a list to [] will break references, while clear() retains them.
7. Is there any difference in performance between clear() and other methods to empty a list?
The clear() method is generally the most efficient and readable way to empty a list.
8. Can the clear() method be used conditionally?
Yes, you can use clear() conditionally within control structures like if statements.
9. Is it necessary to import any modules to use the clear() method?
No, the clear() method is built into Python and does not require any additional modules.
Python Code Editor:
Previous: Python List append() Method
Next: Python List copy() Method
Test your Python skills with w3resource's quiz
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics