Python List sort() Method
sort() Method
The sort() method is used to sort the items of a given list.
The sort() method is a powerful tool for organizing lists in Python. It allows for ascending and descending order sorting, and with the key parameter, you can customize the sorting criteria. Whether you are sorting simple lists, lists of dictionaries, or complex nested structures, understanding the sort() method is essential for effective data manipulation.
Visual Explanation:
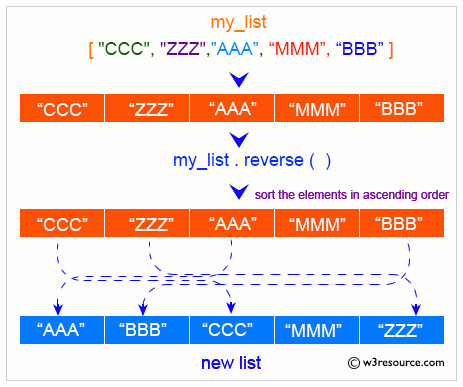
Syntax:
list.sort(*, key=None, reverse=False)
Parameter:
- Key: Optional. A function that specifies the sorting criteria.
- reverse - Default value of reverse is False. False sort the list in ascending order and True sort the list in descending order.
Return Value:
The sort() method doesn't return any value. Rather, it modifies the original list.
Example 1: Sorting a List in Ascending and Descending Order
# colors list
colors = ['Red', 'Green', 'Orange', 'Pink']
print("Original List:")
print(colors)
# Sort the list in ascending order
colors.sort()
print("\nSorted list in ascending order:")
print(colors)
# Sort the list in descending order
colors.sort(reverse=True)
print("\nSorted list in descending order:")
print(colors)
Output:
Original List: ['Red', 'Green', 'Orange', 'Pink'] Sorted list in ascending order: ['Green', 'Orange', 'Pink', 'Red'] Sorted list in descending order: ['Red', 'Pink', 'Orange', 'Green']
Example 2: Sorting a List Using a Custom Key Function
# Define a custom key function
def get_math_score(student):
return student['Math']
# List of students with their math scores
students = [
{'V': 'Jenny Vang', 'Math': 99},
{'V': 'Allan Barker', 'Math': 100},
{'V': 'Bradley Whitney', 'Math': 97},
{'V': 'Ellie Ross', 'Math': 98},
{'V': 'Antoine Booker', 'Math': 99}
]
print("Original students list:")
print(students)
# Sort the list of students by their math scores
students.sort(key=get_math_score)
print("\nStudents list after sorting by Math scores:")
print(students)
Output:
Original students list: [{'V': 'Jenny Vang', 'Math': 99}, {'V': 'Allan Barker', 'Math': 100}, {'V': 'Bradley Whitney', 'Math': 97}, {'V': 'Ellie Ross', 'Math': 98}, {'V': 'Antoine Booker', 'Math': 99}] Students list after sorting by Math scores: [{'V': 'Bradley Whitney', 'Math': 97}, {'V': 'Ellie Ross', 'Math': 98}, {'V': 'Jenny Vang', 'Math': 99}, {'V': 'Antoine Booker', 'Math': 99}, {'V': 'Allan Barker', 'Math': 100}]
Example 3: Sorting a List of Tuples
# List of tuples
tuples_list = [(1, 'one'), (3, 'three'), (2, 'two'), (4, 'four')]
print("Original List of Tuples:")
print(tuples_list)
# Sort the list of tuples by the second element
tuples_list.sort(key=lambda x: x[1])
print("\nSorted List of Tuples by second element:")
print(tuples_list)
Output:
Original List of Tuples: [(1, 'one'), (3, 'three'), (2, 'two'), (4, 'four')] Sorted List of Tuples by second element: [(4, 'four'), (1, 'one'), (3, 'three'), (2, 'two')]
Example 4: Sorting a List of Dictionaries by Multiple Keys
# List of dictionaries
students = [
{'name': 'Jenny', 'grade': 'A', 'age': 16},
{'name': 'Tom', 'grade': 'B', 'age': 17},
{'name': 'Sue', 'grade': 'A', 'age': 15},
{'name': 'Mike', 'grade': 'B', 'age': 16},
]
# Sort by grade and then by age
students.sort(key=lambda x: (x['grade'], x['age']))
print("\nSorted list of students by grade and age:")
print(students)
Output:
Sorted list of students by grade and age: [{'name': 'Sue', 'grade': 'A', 'age': 15}, {'name': 'Jenny', 'grade': 'A', 'age': 16}, {'name': 'Mike', 'grade': 'B', 'age': 16}, {'name': 'Tom', 'grade': 'B', 'age': 17}]
Python list.sort() Method - FAQ
1. What does the list.sort() method do?
The list.sort() method sorts the elements of a list in ascending order by default.
2. Can the list.sort() method sort in descending order?
Yes, the list.sort() method can sort the elements in descending order by using an optional argument.
3. Does the list.sort() method change the original list?
Yes, the list.sort() method sorts the list in place, meaning it modifies the original list.
4. Can the list.sort() method handle different data types?
The list.sort() method can sort lists containing elements of the same data type. Sorting lists with mixed data types may result in a TypeError.
5. Is the list.sort() method stable?
Yes, the list.sort() method is stable, meaning it preserves the order of equal elements.
6. Can I customize the sorting order with the list.sort() method?
Yes, you can customize the sorting order by providing a key function as an optional argument.
7. Is the list.sort() method efficient for large lists?
The list.sort() method is efficient and uses Timsort, which has an average time complexity of O(n log n).
8. What happens if the list contains None values?
If the list contains None values, they will typically cause a TypeError unless handled appropriately with a key function.
9. Is there an alternative to the list.sort() method that does not modify the original list?
Yes, the sorted() function returns a new sorted list without modifying the original list.
10. How does the list.sort() method handle case sensitivity?
By default, the list.sort() method is case-sensitive and sorts uppercase letters before lowercase ones. Custom key functions can be used to perform case-insensitive sorting.
Python Code Editor:
Previous: Python List reverse() Method
Next: Python List
Test your Python skills with w3resource's quiz