Laravel (5.7) Compiling Assets
Step 1: Install Laravel 5.7
Go to your terminal and type the following.
composer create-project laravel/laravel mix --prefer-dist
Now, after installing, go into the project directory and open the package.json file. It has devDependencies like the following.
"devDependencies": {
"axios": "^0.17",
"bootstrap": "^4.0.0",
"popper.js": "^1.12",
"cross-env": "^5.1",
"jquery": "^3.2",
"laravel-mix": "^2.0",
"lodash": "^4.17.4",
"vue": "^2.5.7"
}
So basically, what we need is to hit the following command to install our dependencies local in our project.
npm install
It will grab all the packages and dump in the node_modules folder inside our root directory.
Step 2: Running Mix.
A mix is a configuration layer on top of Webpack, so to run the Mix tasks you only need to execute one of the NPM scripts that are included with the default Laravel package.json file.
// Run all Mix tasks...
npm run dev
// Run all Mix tasks and minify output...
npm run production
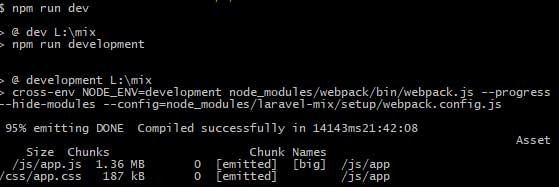
It will compile our CSS and JS files and put the build inside a public folder. We then include this CSS and JS files inside our master blade file. You can see the code inside the file webpack.mix.js file. The webpack.mix.js file resides in the Laravel project root folder.
// webpack.mix.js
let mix = require('laravel-mix');
/*
|--------------------------------------------------------------------------
| Mix Asset Management
|--------------------------------------------------------------------------
|
| Mix provides a clean, fluent API for defining some Webpack build steps
| for your Laravel application. By default, we are compiling the Sass
| file for the application as well as bundling up all the JS files.
|
*/
mix.js('resources/assets/js/app.js', 'public/js')
.sass('resources/assets/sass/app.scss', 'public/css');
First, we have required the mix module and then call its different methods. We can chain those methods.
Less Files
The less method used to compile Less into CSS. First, let us create one new folder inside resources >> assets directory called less. Now in that folder make one file caledl app.less.
@nice-blue: #5B83AD;
@light-blue: @nice-blue + #111;
.title {
color: @light-blue;
}
Now, we need to include this class in the welcome.blade.php file. So we can see the changes.
<!doctype html>
<html lang="{{ app()->getLocale() }}">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Laravel</title>
<!-- Fonts -->
<link href="https://fonts.googleapis.com/css?family=Raleway:100,600" rel="stylesheet" type="text/css"/>
</head>
<body>
<div class="flex-center position-ref full-height ">
<div class="content">
<div class="title">
Laravel
</div>
</div>
</div>
</body>
</html>
Now, write the code inside a webpack.mix.js file.
// webpack.mix.js
mix.js('resources/assets/js/app.js', 'public/js')
.less('resources/assets/less/app.less', 'public/css');
Okay, so we have written the code to compile the less code into the CSS code. Start the webpack compilation process by hitting the following command.
npm run watch
Also, we need to include the app.css file inside the welcome.blade.php file.
<link href="{{ asset('css/app.css') }}" rel="stylesheet" type="text/css"/>
Now, you can start the Laravel server.
php artisan serve
Go to the http://localhost:8000. You can see the changes are reflected in the file.
Sass Files
The sass method allows you to compile Sass into CSS. You may use the way like so.
mix.sass('resources/assets/sass/app.scss', 'public/css');
So you can use sass files same as less files.
Stylus Files
Similar to Less and Sass, the stylus method allows you to compile Stylus into CSS.
// webpack.config.js
mix.stylus('resources/assets/stylus/app.styl', 'public/css');
PostCSS Files
PostCSS, a powerful tool for transforming your CSS, is included with Laravel Mix out of the box.
// webpack.config.js
mix.sass('resources/assets/sass/app.scss', 'public/css')
.options({
postCss: [
require('postcss-css-variables')()
]
});
Source Maps
Though disabled by default, source maps may be activated by calling the mix.sourceMaps() method in your webpack.mix.js file.
// webpack.mix.js
mix.js('resources/assets/js/app.js', 'public/js')
.sourceMaps();
Javascript Files
The mix provides several features to help you work with your JavaScript files, such as compiling ECMAScript 2015, module bundling, minification, and concatenating plain JavaScript files.
mix.js('resources/assets/js/app.js', 'public/js');
With this single line of code, you may now take advantage of:
- ES2015 syntax.
- Modules
- Compilation of .vue files.
- Minification for production environments.
React
Mix can automatically install the Babel plug-ins necessary for React support. To get started, replace your mix.js() call with mix.react().
mix.react('resources/assets/js/app.jsx', 'public/js');
Vanilla JS
// webpack.mix.js
mix.scripts([
'public/js/admin.js',
'public/js/dashboard.js'
], 'public/js/all.js');
So this is how you can use Laravel Mix to compile your assets.
Previous:
Laravel (5.7) Localization
Next:
Laravel (5.7) Frontend Scaffolding