MySQL TRIM() function
TRIM() function
The TRIM() function in MySQL is a powerful tool used to remove unwanted characters from the beginning, end, or both ends of a string. This function is particularly useful in data cleansing tasks, ensuring that strings are free from leading and trailing whitespace or specified characters.
Key Uses of the TRIM() Function:
- One of the most common applications is to clean up user input by removing excess spaces.
- TRIM() can strip unwanted prefixes or suffixes from data fields, aiding in maintaining consistent and clean data.
- Before storing or displaying data, TRIM() helps in standardizing strings to remove any unnecessary padding.
Syntax:
TRIM([{BOTH | LEADING | TRAILING} [remstr] FROM ] str)
Arguments:
Name | Description |
---|---|
BOTH | Removes characters from both the left and right sides of the string. |
LEADING | Removes characters only from the left side of the string. |
TRAILING | Removes characters only from the right side of the string. |
remstr | The string to be removed from the specified side(s) of the original string. |
str | The actual string from which characters are to be removed. |
Note: If no remstr is specified, TRIM() defaults to removing spaces.
Syntax Diagram:
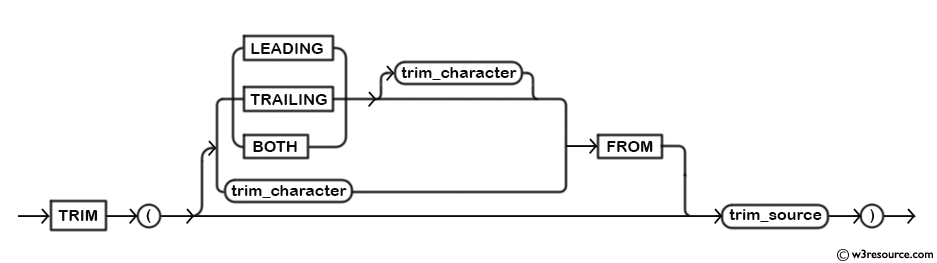
MySQL Version Compatibility:
- Supported Versions: The TRIM() function is available and behaves consistently across MySQL versions 8.0 and later.
Visual Presentation:
Examples : MySQL TRIM() function
Basic Example:
The following MySQL statement returns the string after removing the leading and trailing spaces from the given string ' trim '.
Code:
-- This SQL statement demonstrates the use of the TRIM() function in MySQL
SELECT
TRIM(' trim ') AS "Trimmed String" -- Apply the TRIM() function to
--remove leading and trailing spaces from the string ' trim '
FROM
DUAL; -- The DUAL table is a special dummy table used in MySQL for executing
--queries without referencing any actual database table
Explanation:
- This SQL query uses the TRIM() function to remove any leading and trailing spaces from the given string ' trim '.
- The TRIM() function processes the input string, removing all spaces from both the beginning and the end, resulting in the cleaned string 'trim'. The result is returned with the alias "Trimmed String".
- The FROM DUAL clause indicates the use of the DUAL table, which is a special table in MySQL used for queries that do not require data from any real table.
Output:
mysql> SELECT TRIM(' trim '); +----------------+ | TRIM(' trim ') | +----------------+ | trim | +----------------+ 1 row in set (0.00 sec)
Removing Leading Substring
The following MySQL statement returns the string after removing the leading string 'leading' from the given string 'leadingtext'.
Code:
-- This SQL statement demonstrates the use of the TRIM() function in MySQL to
--remove a specific leading substring
SELECT
TRIM(LEADING 'leading' FROM 'leadingtext') AS "Trimmed String"
-- The TRIM() function removes the specified leading substring 'leading'
--from the start of the string 'leadingtext'
FROM
DUAL;
-- The DUAL table is a special dummy table used in MySQL for executing
--queries that do not require data from any actual database table
Explanation:
- This SQL query uses the TRIM() function to remove a specified leading substring 'leading' from the beginning of the given string 'leadingtext'.
- The TRIM(LEADING ... FROM ...) syntax specifically targets the leading part of the string, ensuring that only the occurrences of 'leading' at the start are removed.
- The cleaned string, 'text', is returned with the alias "Trimmed String".
- The FROM DUAL clause is included as a placeholder to execute the query without referencing any actual table.
Output:
mysql> SELECT TRIM(LEADING 'leading' FROM 'leadingtext' ); +---------------------------------------------+ | TRIM(LEADING 'leading' FROM 'leadingtext' ) | +---------------------------------------------+ | text | +---------------------------------------------+ 1 row in set (0.02 sec)
Removing Trailing Substring
The following MySQL statement returns the string after removing the trailing string 'trailing' from the given string 'texttrailing'.
Code:
-- This SQL statement demonstrates the use of the TRIM() function in
--MySQL to remove a specific trailing substring
SELECT
TRIM(TRAILING 'trailing' FROM 'texttrailing') AS "Trimmed String"
-- The TRIM() function removes the specified trailing substring 'trailing'
-from the end of the string 'texttrailing'
FROM
DUAL;
-- The DUAL table is a special dummy table used in MySQL for executing
--queries that do not require data from any actual database table
Explanation:
- This SQL query uses the TRIM() function to remove a specified trailing substring 'trailing' from the end of the given string 'texttrailing'.
- The TRIM(TRAILING ... FROM ...) syntax specifically targets the trailing part of the string, ensuring that only the occurrences of 'trailing' at the end are removed.
- The cleaned string, 'text', is returned with the alias "Trimmed String".
- The FROM DUAL clause is included as a placeholder to execute the query without referencing any actual table.
Output:
mysql> SELECT TRIM(TRAILING 'trailing' FROM 'texttrailing' ); +------------------------------------------------+ | TRIM(TRAILING 'trailing' FROM 'texttrailing' ) | +------------------------------------------------+ | text | +------------------------------------------------+ 1 row in set (0.00 sec)
Removing Both Leading and Trailing Substrings
The following MySQL statement returns the string after removing the leading and trailing string 'leadtrail' from the given string 'leadtrailtextleadtrail'.
Code:
-- This SQL statement uses the TRIM() function in MySQL to remove a specific substring from both the beginning and the end of a given string.
SELECT
TRIM(BOTH 'leadtrail' FROM 'leadtrailtextleadtrail') AS "Trimmed String"
-- The TRIM(BOTH 'leadtrail' FROM 'leadtrailtextleadtrail') function removes the substring 'leadtrail' from both ends of the string 'leadtrailtextleadtrail'.
FROM
DUAL;
-- The DUAL table is a special table used in MySQL for executing queries that don't require data from any real table.
Explanation:
- The function TRIM(BOTH 'leadtrail' FROM 'leadtrailtextleadtrail') targets and removes the substring 'leadtrail' from both the start and end of 'leadtrailtextleadtrail'.
- The query uses the special MySQL table DUAL, which is commonly used for operations that don't involve real tables.
- The result of the operation is a trimmed string, with the specified substring removed from both ends.
Output:
mysql> SELECT TRIM(BOTH 'leadtrail' FROM 'leadtrailtextleadtrail'); +------------------------------------------------------+ | TRIM(BOTH 'leadtrail' FROM 'leadtrailtextleadtrail') | +------------------------------------------------------+ | text | +------------------------------------------------------+ 1 row in set (0.00 sec)
Common Mistakes and Tips
- Beware of Case Sensitivity: The TRIM() function removes exact matches only, so ensure that the case matches when specifying the remstr.
- Handling Empty Strings: If the remstr is an empty string, the function will still return the original string unchanged.
- Performance Considerations: Although TRIM() is efficient, excessive use on large datasets can impact performance. It's best used in data preprocessing stages.
Alternatives and Related Functions
For more specialized string manipulations, consider using:
- RTRIM(): Removes trailing spaces or specified characters.
- LTRIM(): Removes leading spaces or specified characters.
- REPLACE(): Substitutes all occurrences of a specified substring.
Frequently Asked Questions (FAQ) - MySQL TRIM() Function
1. What is the MySQL TRIM() function?
The TRIM() function in MySQL is used to remove unwanted characters from the beginning, end, or both ends of a string. It is a versatile tool for cleaning and standardizing string data by eliminating leading and trailing whitespace or specified characters.
2. What are the key uses of the TRIM() function?
- Removing Leading and Trailing Spaces: It is commonly used to clean up user input by removing excess spaces from both ends of a string.
- Data Cleansing: TRIM() helps in removing unwanted prefixes or suffixes, ensuring that data fields are clean and consistent.
- Standardizing Data: Before storing or displaying data, TRIM() ensures strings are free of unnecessary padding, leading to better data presentation and storage.
3. What types of substrings can be removed with TRIM()?
TRIM() can remove specified characters or substrings from:
- Both Ends: Removes characters from both the left and right sides of the string.
- Leading End: Removes characters only from the left side (beginning) of the string.
- Trailing End: Removes characters only from the right side (end) of the string.
4. What happens if no specific substring is provided to TRIM()?
If no specific substring (remstr) is provided, TRIM() defaults to removing spaces from the string. This is useful for general whitespace removal without specifying a particular character.
5. What are the supported MySQL versions for the TRIM() function?
The TRIM() function is available and behaves consistently across MySQL versions 8.0 and later. It provides reliable functionality for string manipulation in these versions.
6. What are some common mistakes to avoid when using TRIM()?
- Case Sensitivity: TRIM() is case-sensitive, meaning it only removes exact matches of the specified substring. Ensure the case matches when specifying the substring to be trimmed.
- Handling Empty Strings: If the specified substring is empty, TRIM() will return the original string unchanged.
- Performance Considerations: Although efficient, excessive use of TRIM() on large datasets can impact performance. It's advisable to use it judiciously, especially during data preprocessing stages.
7. Are there alternative functions to TRIM() for specific tasks?
Yes, MySQL offers other functions for specialized string manipulations:
- RTRIM(): Removes trailing spaces or specified characters from the end of a string.
- LTRIM(): Removes leading spaces or specified characters from the beginning of a string.
- REPLACE(): Substitutes all occurrences of a specified substring within a string.
8. How does TRIM() function handle special characters?
TRIM() can handle and remove any specified substring, including special characters, from the ends of a string. This makes it highly adaptable for various data cleaning scenarios.
9. How does TRIM() work with different data types?
TRIM() operates on string data types. It can process strings of varying lengths and characters, provided they are of a type supported by MySQL, such as CHAR, VARCHAR, TEXT, etc.
10. Can TRIM() be used in combination with other string functions?
Yes, TRIM() can be combined with other MySQL string functions like REPLACE(), SUBSTRING(), and CONCAT() for more complex string manipulations. This allows for powerful and flexible data transformation capabilities in SQL queries.
Video Presentation:
All String Functions (Slides presentation)
Previous: SUBSTRING() function
Next: UCASE() function