Python Math: Clamps number within the inclusive range
Python Math: Exercise-87 with Solution
Write a Python program to cap a number within the inclusive range specified by the given boundary values x and y.
- If num falls within the range (a, b), return num.
- Otherwise, return the nearest number in the range.
Sample Solution:
Python Code:
def clamp_number(num, a, b):
return max(min(num, max(a, b)), min(a, b))
print(clamp_number(2, 4, 7))
print(clamp_number(1, -1, -4))
print(clamp_number(22, -10, 10))
print(clamp_number(20, 1, 10))
print(clamp_number(5, 1, 20))
print(clamp_number(1, 10, 20))
Sample Output:
4 -1 10 10 5 10
Flowchart:
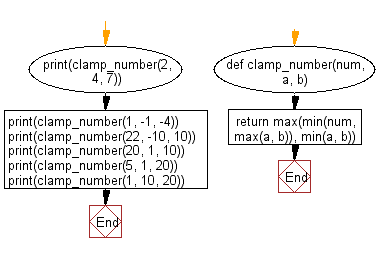
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the Hamming distance between two given values.
Next: Write a Python program to check whether a given number is a Disarium number or unhappy number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/math/python-math-exercise-87.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics