SQL MAX() with COUNT()
MAX() with Count function
Overview
Combining the SQL MAX() and COUNT() functions allows for powerful data analysis within our database queries. These functions together can help us find the maximum values in datasets while counting occurrences, enabling insights into the distribution and characteristics of our data.
- MAX(): Returns the highest value in a specified column.
- COUNT(): Returns the number of rows that match a specified condition.
Using these functions together is particularly useful for summarizing and analyzing grouped data, such as finding the maximum count of occurrences of a specific column value.
Key Concepts
- Aggregation: Both MAX() and COUNT() are aggregate functions that perform calculations on multiple rows of a table's column and return a single value.
- Grouping: To effectively use these functions together, grouping by a specific column is often necessary. The GROUP BY clause is crucial in SQL to group rows that have the same values into summary rows.
- Subqueries: A subquery can be used to perform an intermediate calculation that is then used in the main query. This is common in scenarios where you need to combine MAX() with COUNT().
Finding the Maximum Count of Groups
Sample table: ordersORD_NUM ORD_AMOUNT ADVANCE_AMOUNT ORD_DATE CUST_CODE AGENT_CODE ORD_DESCRIPTION ---------- ---------- -------------- --------- --------------- --------------- ----------------- 200114 3500 2000 15-AUG-08 C00002 A008 200122 2500 400 16-SEP-08 C00003 A004 200118 500 100 20-JUL-08 C00023 A006 200119 4000 700 16-SEP-08 C00007 A010 200121 1500 600 23-SEP-08 C00008 A004 200130 2500 400 30-JUL-08 C00025 A011 200134 4200 1800 25-SEP-08 C00004 A005 200108 4000 600 15-FEB-08 C00008 A004 200103 1500 700 15-MAY-08 C00021 A005 200105 2500 500 18-JUL-08 C00025 A011 200109 3500 800 30-JUL-08 C00011 A010 200101 3000 1000 15-JUL-08 C00001 A008 200111 1000 300 10-JUL-08 C00020 A008 200104 1500 500 13-MAR-08 C00006 A004 200106 2500 700 20-APR-08 C00005 A002 200125 2000 600 10-OCT-08 C00018 A005 200117 800 200 20-OCT-08 C00014 A001 200123 500 100 16-SEP-08 C00022 A002 200120 500 100 20-JUL-08 C00009 A002 200116 500 100 13-JUL-08 C00010 A009 200124 500 100 20-JUN-08 C00017 A007 200126 500 100 24-JUN-08 C00022 A002 200129 2500 500 20-JUL-08 C00024 A006 200127 2500 400 20-JUL-08 C00015 A003 200128 3500 1500 20-JUL-08 C00009 A002 200135 2000 800 16-SEP-08 C00007 A010 200131 900 150 26-AUG-08 C00012 A012 200133 1200 400 29-JUN-08 C00009 A002 200100 1000 600 08-JAN-08 C00015 A003 200110 3000 500 15-APR-08 C00019 A010 200107 4500 900 30-AUG-08 C00007 A010 200112 2000 400 30-MAY-08 C00016 A007 200113 4000 600 10-JUN-08 C00022 A002 200102 2000 300 25-MAY-08 C00012 A012
In this part, you will see the usage of SQL COUNT() along with the SQL MAX().
To get the maximum number of agents (mycount) from the orders table, grouped by agent_code, you can use the following SQL query:
SELECT MAX(mycount) -- Selecting the maximum value of the column 'mycount'
FROM ( -- Subquery: Creating a derived table
SELECT agent_code, COUNT(agent_code) AS mycount -- Selecting 'agent_code' and its count, aliased as 'mycount'
FROM orders -- From the 'orders' table
GROUP BY agent_code -- Grouping the results by 'agent_code'
);
Explanation:
- SELECT MAX(mycount): This line selects the maximum value of the column mycount from the result set returned by the subquery.
- (SELECT agent_code, COUNT(agent_code) AS mycount FROM orders GROUP BY agent_code): This is a subquery that generates a derived table. It selects the agent_code column and counts the occurrences of each agent_code in the orders table. The COUNT(agent_code) function is used to count the occurrences of each agent_code. The results are grouped by agent_code.
- SELECT agent_code, COUNT(agent_code) AS mycount: This line within the subquery selects the agent_code column and counts the occurrences of each agent_code in the orders table. The COUNT(agent_code) function is used to count the occurrences of each agent_code, and it is aliased as mycount.
- FROM orders: This specifies the table from which the subquery is selecting data, which is the orders table.
- GROUP BY agent_code: This line groups the results of the subquery by the agent_code column. This is necessary because we're using an aggregate function (COUNT) in conjunction with a non-aggregated column (agent_code). Grouping allows us to count the occurrences of each agent_code separately.
Output:
MAX(MYCOUNT) ------------ 7
Visual Presentation :
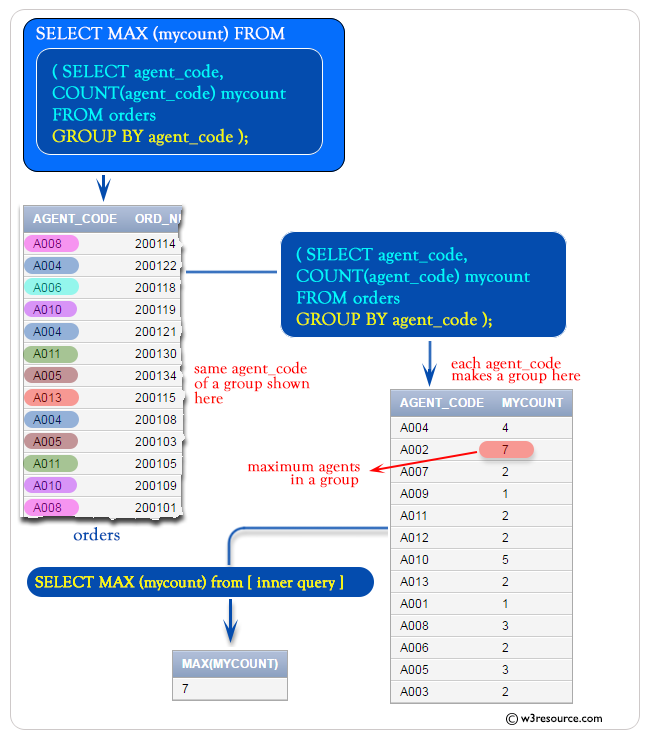
SQL MAX() and COUNT() with HAVING
Finding Groups with the Maximum Count Using HAVING Sample table: ordersORD_NUM ORD_AMOUNT ADVANCE_AMOUNT ORD_DATE CUST_CODE AGENT_CODE ORD_DESCRIPTION ---------- ---------- -------------- --------- --------------- --------------- ----------------- 200114 3500 2000 15-AUG-08 C00002 A008 200122 2500 400 16-SEP-08 C00003 A004 200118 500 100 20-JUL-08 C00023 A006 200119 4000 700 16-SEP-08 C00007 A010 200121 1500 600 23-SEP-08 C00008 A004 200130 2500 400 30-JUL-08 C00025 A011 200134 4200 1800 25-SEP-08 C00004 A005 200108 4000 600 15-FEB-08 C00008 A004 200103 1500 700 15-MAY-08 C00021 A005 200105 2500 500 18-JUL-08 C00025 A011 200109 3500 800 30-JUL-08 C00011 A010 200101 3000 1000 15-JUL-08 C00001 A008 200111 1000 300 10-JUL-08 C00020 A008 200104 1500 500 13-MAR-08 C00006 A004 200106 2500 700 20-APR-08 C00005 A002 200125 2000 600 10-OCT-08 C00018 A005 200117 800 200 20-OCT-08 C00014 A001 200123 500 100 16-SEP-08 C00022 A002 200120 500 100 20-JUL-08 C00009 A002 200116 500 100 13-JUL-08 C00010 A009 200124 500 100 20-JUN-08 C00017 A007 200126 500 100 24-JUN-08 C00022 A002 200129 2500 500 20-JUL-08 C00024 A006 200127 2500 400 20-JUL-08 C00015 A003 200128 3500 1500 20-JUL-08 C00009 A002 200135 2000 800 16-SEP-08 C00007 A010 200131 900 150 26-AUG-08 C00012 A012 200133 1200 400 29-JUN-08 C00009 A002 200100 1000 600 08-JAN-08 C00015 A003 200110 3000 500 15-APR-08 C00019 A010 200107 4500 900 30-AUG-08 C00007 A010 200112 2000 400 30-MAY-08 C00016 A007 200113 4000 600 10-JUN-08 C00022 A002 200102 2000 300 25-MAY-08 C00012 A012
To get data of 'agent_code', and number of agents for each group of 'agent_code' from the orders table with the following conditions -
'agent_code' for a group will be equal to the result of an outer query [SELECT MAX(agent_code).......] with following condition -
the outer query produce the maximum number of agents mentioned as
'mycount' from an inner query [SELECT agent_code,
COUNT(agent_code) mycount FROM orders GROUP BY agent_code]
with following condition -
the inner query produced the data 'agent_code' number of agents as column alias 'mycount' from the 'orders' table with the following condition -
'agent_code' should be in a group,
the following SQL statement can be used :
SELECT agent_code, COUNT(agent_code) -- Selecting 'agent_code' and its count
FROM orders -- From the 'orders' table
GROUP BY agent_code -- Grouping the results by 'agent_code'
HAVING COUNT(agent_code) = ( -- Applying a condition on the grouped counts
SELECT MAX(mycount) -- Selecting the maximum count from a subquery
FROM ( -- Subquery: Creating a derived table
SELECT agent_code, COUNT(agent_code) AS mycount -- Selecting 'agent_code' and its count, aliased as 'mycount'
FROM orders -- From the 'orders' table
GROUP BY agent_code -- Grouping the results by 'agent_code'
)
);
Explanation:
- SELECT agent_code, COUNT(agent_code): This line selects the agent_code column and counts the occurrences of each agent_code in the orders table.
- FROM orders: This specifies the table from which data is being selected, which is the orders table.
- GROUP BY agent_code: This line groups the results by the agent_code column. This is necessary because we're using an aggregate function (COUNT) in conjunction with a non-aggregated column (agent_code). Grouping allows us to count the occurrences of each agent_code separately.
- HAVING COUNT(agent_code) = (...): This line filters the grouped results based on a condition. It selects groups where the count of agent_code matches the result of the subquery.
- SELECT MAX(mycount): This subquery selects the maximum value of the column mycount.
- (SELECT agent_code, COUNT(agent_code) AS mycount ... ): This is a subquery that generates a derived table. It calculates the count of occurrences of each agent_code in the orders table and aliases it as mycount.
- SELECT agent_code, COUNT(agent_code) AS mycount: This line within the subquery selects the agent_code column and counts the occurrences of each agent_code in the orders table. The COUNT(agent_code) function is used to count the occurrences of each agent_code, and it is aliased as mycount.
- FROM orders: This specifies the table from which the subquery is selecting data, which is the orders table.
- GROUP BY agent_code: This line groups the results of the subquery by the agent_code column. This is necessary because we're using an aggregate function (COUNT) in conjunction with a non-aggregated column (agent_code). Grouping allows us to count the occurrences of each agent_code separately.
Output:
AGENT_CODE COUNT(AGENT_CODE) ---------- ----------------- A002 7
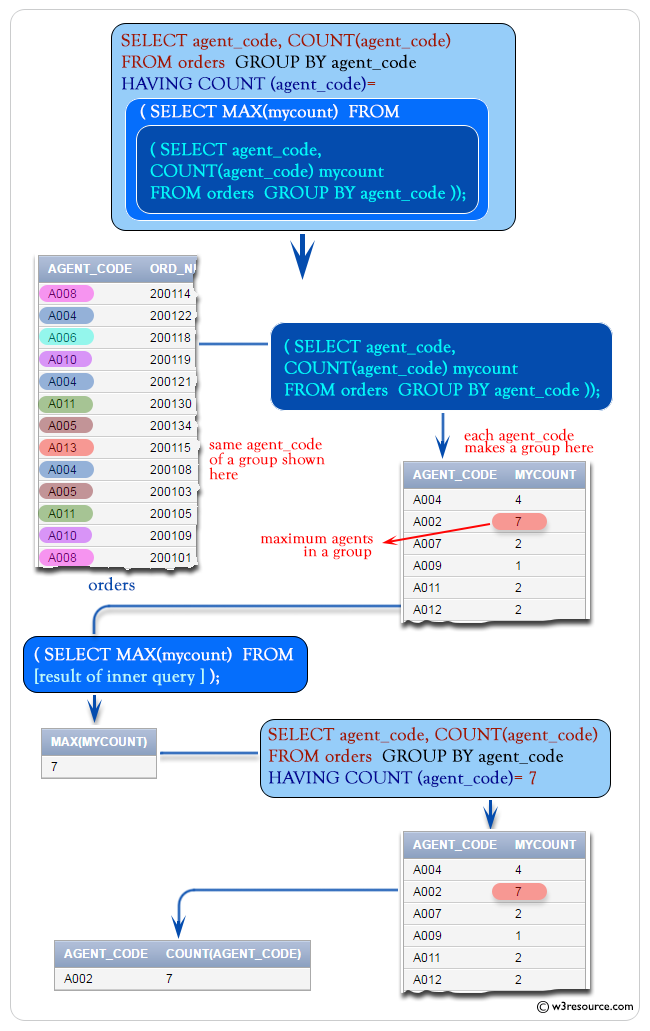
Note: Outputs of the said SQL statement shown here is taken by using Oracle Database 10g Express Edition
Performance Considerations
- Indexes: Ensure that columns involved in GROUP BY and aggregate functions are indexed to optimize performance.
- Query Complexity: Using subqueries and aggregation can increase query complexity and execution time. Consider optimizing your database schema or using materialized views for complex aggregations.
- Database Load: Aggregations and groupings on large datasets can be resource-intensive. Monitor and optimize queries to minimize the impact on database performance.
Comparison with Similar Functions
- SUM(): Useful when you need the total sum rather than the maximum count.
- AVG(): Use AVG() when interested in the average value of a column rather than the maximum or count.
Frequently Asked Questions (FAQ) - SQL MAX() with COUNT()
1. What is the purpose of combining SQL MAX() with COUNT()?
- Combining SQL MAX() with COUNT() is used to analyze data by finding the maximum values while counting occurrences. This combination helps in summarizing and understanding the distribution and characteristics of data within grouped categories.
2. How do the SQL MAX() and COUNT() functions work together?
- The MAX() function returns the highest value in a specified column, while the COUNT() function returns the number of rows that match a specified condition. Together, they can be used to find the highest count of occurrences for specific data groups.
3. What is aggregation in the context of SQL MAX() and COUNT()?
- Aggregation refers to performing calculations on multiple rows to return a single summary value. Both MAX() and COUNT() are aggregate functions, meaning they compute a single value from a set of input values.
4. Why is grouping necessary when using SQL MAX() with COUNT()?
- Grouping is necessary to organize rows with the same values into summary rows. It allows the application of aggregate functions like MAX() and COUNT() on each group separately. This is done using the GROUP BY clause.
5. What role do subqueries play in combining SQL MAX() with COUNT()?
- Subqueries are used to perform intermediate calculations that provide results for the main query. When combining MAX() with COUNT(), subqueries often calculate the count of occurrences, which are then used to determine the maximum value across these counts.
6. How can SQL MAX() and COUNT() be used to find the maximum count of groups?
- By grouping data by a specific column and counting the occurrences in each group, we can then apply MAX() to find the group with the highest count of occurrences.
7. What is the significance of using the HAVING clause with SQL MAX() and COUNT()?
- The HAVING clause is used to filter the results of a GROUP BY query based on conditions applied to aggregate functions. It is essential for comparing group counts with the maximum count found using a subquery.
8. What are some performance considerations when using SQL MAX() with COUNT()?
- Ensuring that columns used in GROUP BY and aggregate functions are indexed can improve performance. Complex queries with subqueries and aggregations can increase execution time and database load. Optimizing query design and using materialized views can help manage performance.
9. How do SQL MAX() and COUNT() compare to similar functions like SUM() and AVG()?
- While MAX() and COUNT() focus on finding the highest value and counting occurrences, SUM() calculates the total sum of values, and AVG() computes the average value. Each function serves different purposes depending on the data analysis requirement.
10. What are some common use cases for combining SQL MAX() with COUNT()?
- Common use cases include identifying top performers (e.g., the salesperson with the most sales), analyzing peaks in data (e.g., the most frequent error codes), and summarizing grouped data (e.g., the agent with the highest number of orders).
Here is a slide presentation of all aggregate functions.
Check out our 1000+ SQL Exercises with solution and explanation to improve your skills.
Previous: Max Date
Next: Min function
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/sql/aggregate-functions/max-count.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics