Creating, Publishing, and Updating Node.js Modules
In the previous tutorial we talked about the installation, update and uninstallation of a global package. The tutorial you are about to read will work you through how to create a Node.js Module, as well as publish and updating a package.
Wait, what is a Node.js module: a node.js is a type of package that you can publish to npm. If you want to create a new module, you should start by creating a package.json file.
Recall that, to create a package.json file, you need to run npm init. This command will prompt you to enter values for fields. "name" and "version" will be two of the required fields. You will need to set a value for "main" as well. If you want to use the default, use index.js. We have covered these steps in our tutorial on working with package.json
If you need to add information for the author field, you should use the following format (email and website are both optional):
Your Name <[email protected]> (http://example.com)
After you create your package.json file, you should create the file that will be loaded when your module is required. As we stated earlier the default is index.js, but you can name it anytime you want, however ensure that is what is contained in the "main" field of your package.json.
Inside the file you created, you should add a function as a property of the exports object. This makes the function to be available for require.
exports.displayMsg = function() {
console.log("hurrah! I just created my first package!");
}
Testing:
- You should publish your package to npm.
- Then create a new directory outside of your project.
- Switch to the new directory (cd) you created
- Run npm install <yourpackage>.
- Create a test.js file which will require the package and make calls to the method.
- Finally, you should run node test.js. The message that is sent to the console.log should appear.
Publishing and updating a Package
The first section of this tutorial showed you how to create a package. The current section will show you how to publish and update your package.
How to Publish a package?
Preparation
Understand npm policies
Before you start, we recommend, that you review npm's polices, it covers questions on the site etiquette, naming, licensing, or other guidelines.
Create an account
If you have been following our series on npm from the beginning, you can skip this.
For you to publish, you have to be a user on the npm registry. If you are not already a user, you can create an account by using npm adduser. If you created your account on the site, you should use npm login to access your account from the terminal.
Test:
- Type npm whoami from your terminal to see if you are already logged in (technically, this will also mean that your credentials have been stored locally).
- You should then check that your username has been added to the registry at https://npmjs.com/~username.
For example,
https://www.npmjs.com/~carolynawombat
Review the package directory
Review the Contents
Note that everything in the directory are included unless it is ignored by a local .gitignore or .npmignore file.
Review the package.json File
ead our tutorial on Working with package.json to be sure that the details you want are reflected in your package.
Choose a name
You should choose a unique name for your package. Try choosing a descriptive name that:
- Is not already owned by somebody else
- Isn't spelled like another name, except with typos
- Won't confuse others about the authorship
- Will meet npm policy guidelines. For instance, don't name your package something offensive, and do not use someone else's trademarked name.
- Specify the name in the appropriate line of the package.json file.
Note: The first 3 caveats do not apply if you are using scopes.
Include Documentation (readme.md)
It is recommended by npm that you include a readme file to document your package. The readme file should have the filename readme.md. The file extension .md will indicate that the file is a markdown file. This file appears on the npm website when someone finds your package.
To create a readme.md:
- You should create a file using any text editor.
- Then save it in the project directory with the name readme.md
- When you publish the package, this documentation displays on the web page where people download your package.
Publish!
You should use npm publish to publish the package.
Test
Visit https://npmjs.com/package/<package>. You will see a page all about your new package. It might look somewhat like this:
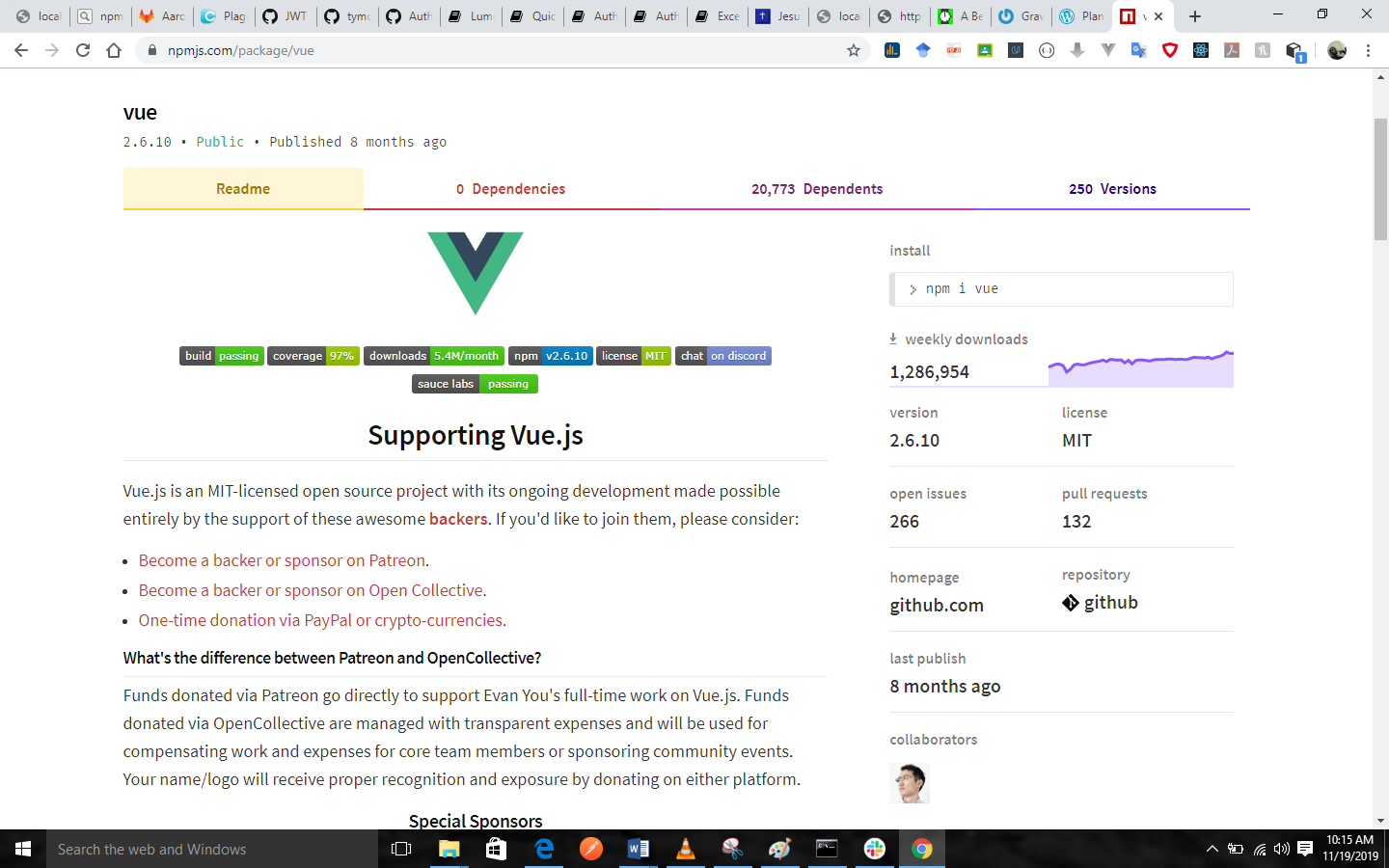
How to Update a Package
How to Update the Version Number
Whenever you make changes, you can make updates to the package using
npm version <update_type>
Here, <update_type> is one of the semantic versioning release types, patch, minor, or major.
This command changes the version number in package.json.
Note: this also adds a tag with the updated release number to your git repository if you have linked one to your npm account.
Once you have updated the version number, you can run npm publish again.
Test:
You should go to https://npmjs.com/package/
How to Update the Read Me File
The README displayed on the site is not updated unless a new version of your package is published, so you will need to run npm version patch and npm publish to update the documentation that is displayed on the site.
Previous:
How to install global packages, update global packages and uninstall global packages
Next:
Using Semantic Versioning, Scoped Packages, and Dist-tags in npm
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/npm/how-to-create-node-and-how-to-publish-and-update-a-package.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics