C Programming Binary Heap (Tree-Based Structure) Exercises with Explanations and Solutions
C Program to implement Heap [9 exercises with solution]
[An editor is available at the bottom of the page to write and execute the scripts. Go to the editor]
From Wikipedia,
In computer science, a heap is a specialized tree-based data structure that satisfies the heap property: In a max heap, for any given node C, if P is a parent node of C, then the key (the value) of P is greater than or equal to the key of C. In a min heap, the key of P is less than or equal to the key of C. The node at the "top" of the heap (with no parents) is called the root node.
The heap is one maximally efficient implementation of an abstract data type called a priority queue, and in fact, priority queues are often referred to as "heaps", regardless of how they may be implemented. In a heap, the highest (or lowest) priority element is always stored at the root.
Visual Presentation:
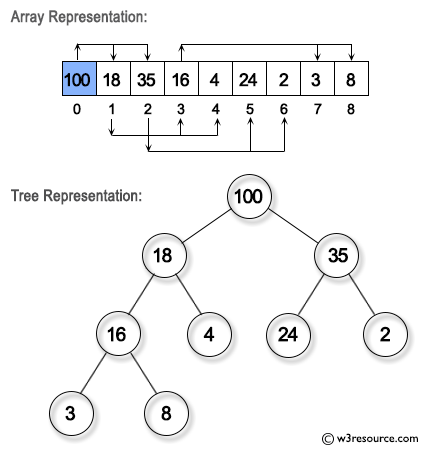
1. Write a C program that implements the basic operations of a heap - insert, delete, and display.
Click me to see the solution
2. Write a C program function that constructs a max heap from an array of elements. Test it with both random and sorted input arrays.
Click me to see the solution
3. Write a C program function that constructs a min heap from an array of elements. Test it with both random and sorted input arrays.
Click me to see the solution
4. Write a C program that uses the max heap data structure to implement heap sort. Show the sorting process on an array of integers.
Click me to see the solution
5. Write a C program that implements a function to heapify a given node in a heap. Check the function with different positions in the heap.
Click me to see the solution
6. Write a C program that implements a priority queue using a max heap. Apply enqueue and dequeue operations on the priority queue.
Click me to see the solution
7. Write a C program that creates a function to merge two heaps into a single heap. Please make sure that the resulting heap satisfies the heap property.
Click me to see the solution
8. Write a C program that implements the decreaseKey operation in a maximum heap. Check the function by decreasing the key of a node and validating the heap property.
Click me to see the solution
9. Write a C program that uses a max heap to find the kth largest element in an array. Write a function that takes an array and an integer k as input and returns the kth largest element.
Click me to see the solutionC Programming Code Editor:
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics