C Programming Exercises: Graph Structure and Algorithms
C Program to implement Graph Structure [10 exercises with solution]
[An editor is available at the bottom of the page to write and execute the scripts. Go to the editor]
From Wikipedia,
In computer science, a graph is an abstract data type that is meant to implement the undirected graph and directed graph concepts from the field of graph theory within mathematics.
A graph data structure consists of a finite (and possibly mutable) set of vertices (also called nodes or points), together with a set of unordered pairs of these vertices for an undirected graph or a set of ordered pairs for a directed graph. These pairs are known as edges (also called links or lines), and for a directed graph are also known as edges but also sometimes arrows or arcs. The vertices may be part of the graph structure, or may be external entities represented by integer indices or references.
Visual Presentation:
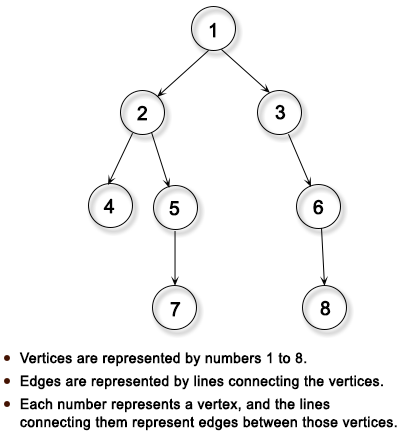
1. Write a C program to represent a graph using an adjacency matrix.
Click me to see the solution
2. Write a C program that implements a function to add a new vertex to an existing graph.
Click me to see the solution
3. Write a C function to add a directed edge between two vertices in a graph.
Click me to see the solution
4. Write a C program that implements DFS (Depth-First Search) traversal for a graph in C. Print the order of visited vertices.
Click me to see the solution
5. Write a C program to perform BFS (Breadth-First Search) traversal on a graph. Print the order of visited vertices.
Click me to see the solution
6. Write a C program that implements a function in C to check whether a given graph contains a cycle or not.
Click me to see the solution
7. Write a C program to perform topological sorting on a directed acyclic graph (DAG).
Click me to see the solution
8. Write a C program that implements Prim's algorithm to find the minimum spanning tree of a connected, undirected graph in C.
Click me to see the solution
9. Write a C program that creates a function to find the shortest path from a source vertex to all other vertices using Dijkstra's algorithm.
Click me to see the solution10. Write a C program to find the traversal order (pre-order, in-order, post-order) of a binary tree that represents a graph.
Click me to see the solutionC Programming Code Editor:
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics