C - Arrays and Pointers
Arrays and Pointers
Pointer
Arrays:
Array is a group of elements that share a common name, and that are different from one another by their positions within the array. Under one name, a collection of elements of the same type is stored in memory.
Advantages:
How arrays are stored in memory?
An array of one-dimensional elements consists of a series of individual variables of the array data type, each stored one after the other in the computer's memory. Each element has an address, just as all other variables do. An array's address is its first element, which corresponds to the first byte of memory it occupies.
Here we declare an array of seven integers like this:
int nums[7] = {54, 6, 23, 45, 32, 78, 89};
Let's assume that the first element of the array scores has the address 1200. This means that &nums[0] would be 1000. Since an int occupies 4 bytes, the address of the second element of the array, &nums[1] would be 1204, the address of the third element of the array, &nums[2] would be 1208, and so on.
[0] | [1] | [2] | [3] | [4] | [5] | [6] | |
nums | 54 | 6 | 23 | 45 | 32 | 78 | 89 |
1200 | 1204 | 1208 | 1212 | 1216 | 1220 | 1224 |
What about a two-dimensional array declared like this?
int nums [2][4] = {{1, 2, 3, 4}, {5, 6, 7, 8}};
Visualize the said two-dimensional array as a table of rows and columns:
column | |||||
[0] | [1] | [2] | [3] | ||
row | [0] | 1 | 2 | 3 | 4 |
[1] | 5 | 6 | 7 | 8 | |
numbers |
C Array: Syntax and Declaration
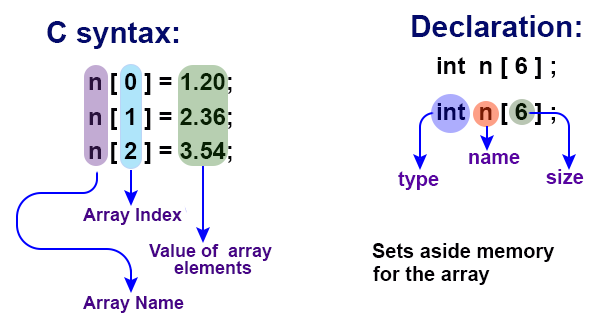
Pointers and arrays in C:
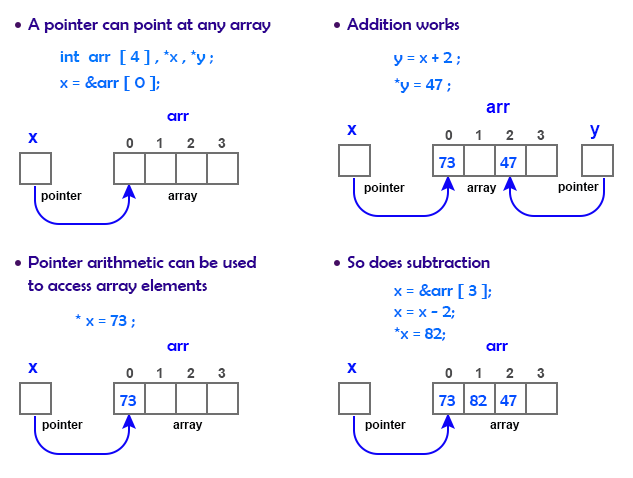
Relationship between Arrays and Pointers
Following 3 for loops are equivalent:
Code:
#include <stdio.h>
#define N 5
int main() {
int i, * ptr, sum = 0;
int nums[N] = {1, 2, 3, 4, 5};
for (ptr = nums; ptr < & nums[N]; ++ptr)
sum += * ptr;
printf("Sum = %d ", sum); // Sum = 15
}
Output:
Sum = 15
Code:
#include <stdio.h>
#define N 5
int main() {
int i, * ptr, sum = 0;
int nums[N] = {1, 2, 3, 4, 5};
for(i = 0; i < N; ++i)
sum += *(nums+i);
printf("Sum = %d ", sum); // Sum = 15
}
Output:
Sum = 15
Code:
#include <stdio.h>
#define N 5
int main() {
int i, * ptr, sum = 0;
int nums[N] = {1, 2, 3, 4, 5};
ptr = nums;
for(i = 0; i < N; ++i)
sum += ptr[i];
printf("Sum = %d ", sum); // Sum = 15
}
Output:
Sum = 15
Example: Arrays and Pointers
Following example print i) array elements using the array notation and ii) by dereferencing the array pointers:
Code:
#include <stdio.h>
int nums[] = {0, 5, 87, 32, 4, 5};
int *ptr;
int main(void)
{
int i;
ptr = &nums[0]; /* pointer to the first element of the array */
printf("Print array elements using the array notation\n");
printf("and by dereferencing the array pointers:\n");
for (i = 0; i < 6; i++)
{
printf("\nnums[%d] = %d ", i , nums[i]);
printf("\nptr + %d = %d\n",i, *(ptr + i));
}
return 0;
}
Output:
Print array elements using the array notation and by dereferencing the array pointers: nums[0] = 0 ptr + 0 = 0 nums[1] = 5 ptr + 1 = 5 nums[2] = 87 ptr + 2 = 87 nums[3] = 32 ptr + 3 = 32 nums[4] = 4 ptr + 4 = 4 nums[5] = 5 ptr + 5 = 5
Passing Arrays as Parameters
Code:
#include <stdio.h>
int sum( int *a, int l) {
int i, s = 0;
for(i = 0; i < l; i++)
s += a[i];
return s;
}
int main()
{
int nums[5] = {1, 2, 3, 4, 5};
int size = sizeof(nums)/sizeof(nums[0]);
printf("Sum is %d\n", sum(nums, size)); //15
return 0;
}
Output:
Sum is 15
Example: Passing a 2D Array to a Function
In the following example, a 2D array is passed as a parameter, where the second dimension is specified and the first one is not specified:
Code:
#include <stdio.h>
void test(int N[][4]) {
int i, j;
printf("\n\nPrint the matrix within the test function:");
for (i = 0; i < 4; i++) {
printf("\n");
for (j = 0; j < 4; j++)
printf("%d ", N[i][j]);
}
printf("\n");
}
int main() {
int nums[4][4], i, j;
for (i = 0; i < 4; i++)
for (j = 0; j < 4; j++)
nums[i][j] = i;
printf("Original Matrix: ");
for (i = 0; i < 4; i++) {
printf("\n");
for (j = 0; j < 4; j++)
printf("%d ", nums[i][j]);
}
test(nums);
}
In the following example, a pointer to a 2D array is passed as a parameter, where the second dimension is specified:
Code:
#include <stdio.h>
void test(int (*N)[4])
{
int i, j;
printf("\n\nPrint the matrix within the test function:");
for(i = 0 ; i < 4 ; i++) {
printf("\n");
for(j = 0 ; j < 4 ; j++)
printf("%d ", N[i][j]);
}
printf("\n");
}
int main() {
int nums[4][4], i, j;
for (i = 0; i < 4; i++)
for (j = 0; j < 4; j++)
nums[i][j] = i;
printf("Original Matrix: ");
for (i = 0; i < 4; i++) {
printf("\n");
for (j = 0; j < 4; j++)
printf("%d ", nums[i][j]);
}
test(nums);
}
In the following example, a pointer to a 2D array is passed as a parameter, where the second dimension is specified:
Code:
#include <stdio.h>
void test(int (*N)[4])
{
int i, j;
printf("\n\nPrint the matrix within the test function:");
for(i = 0 ; i < 4 ; i++) {
printf("\n");
for(j = 0 ; j < 4 ; j++)
printf("%d ", N[i][j]);
}
printf("\n");
}
int main() {
int nums[4][4], i, j;
for (i = 0; i < 4; i++)
for (j = 0; j < 4; j++)
nums[i][j] = i;
printf("Original Matrix: ");
for (i = 0; i < 4; i++) {
printf("\n");
for (j = 0; j < 4; j++)
printf("%d ", nums[i][j]);
}
test(nums);
}
In the following example, a single pointer of a 2D array is passed as parameter:
Code:
#include <stdio.h>
void test(int *N) {
int i, j;
printf("\n\nPrint the matrix within the test function:");
for(i = 0 ; i < 4 ; i++) {
printf("\n");
for(j = 0 ; j < 4 ; j++)
printf("%d ", *(N+ 4*i + j));
}
printf("\n");
}
int main() {
int nums[4][4], i, j;
for (i = 0; i < 4; i++)
for (j = 0; j < 4; j++)
nums[i][j] = i;
printf("Original Matrix: ");
for (i = 0; i < 4; i++) {
printf("\n");
for (j = 0; j < 4; j++)
printf("%d ", nums[i][j]);
}
test(*nums);
}
Output of the said three programs:
Original Matrix: 0 0 0 0 1 1 1 1 2 2 2 2 3 3 3 3 Print the matrix within the test function: 0 0 0 0 1 1 1 1 2 2 2 2 3 3 3 3
Previous: C Pointers and Functions
Next: C <ctype.h>
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming/c-arrays-and-pointers.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics