Java ArrayList.add(int index, E element) Method
public void add(int index, E element)
This method is used to insert an element to the ArrayList object at a specified index.
Package: java.util
Java Platform : Java SE 8
Syntax:
add(int index, E element)
Parameters:
Name | Description | Type |
---|---|---|
index | Index at which the specified element is to be inserted | int |
element | Element to be inserted |
Return Value:
This method does not return any value.
Throws:
IndexOutOfBoundsException - if the index is out of range (index < 0 || index > size())
Pictorial presentation of ArrayList.add() Method
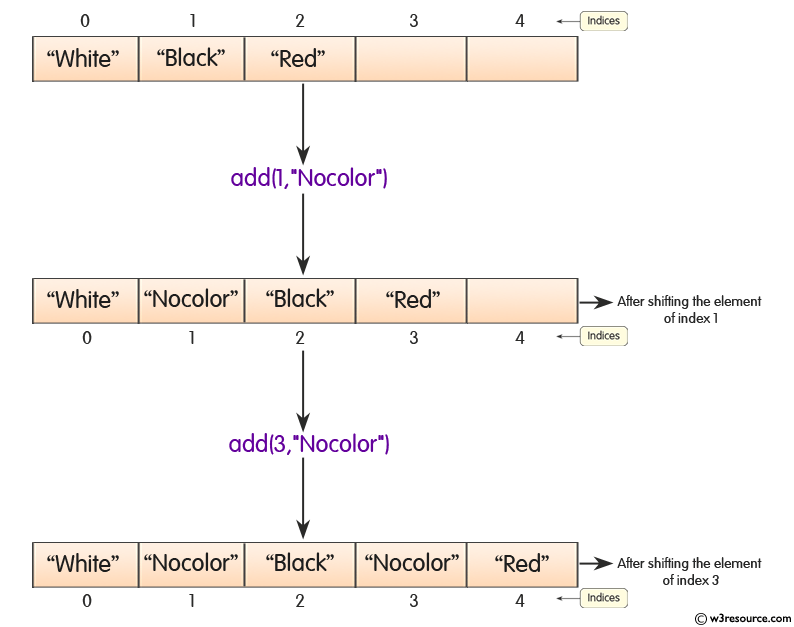
Example: ArrayList.add(int index, E element) Method
The following example creates an ArrayList with a capacity of 7 elements. Using the above method we have inserted two elements in 2nd and 4th position.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(7);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
// Insert Nocolor in 2nd and 4th position in the list
color_list.add(1,"Nocolor");
color_list.add(3,"Nocolor");
// Print out the colors in the ArrayList
for (int i = 0; i < 7; i++)
{
System.out.println(color_list.get(i).toString());
}
}
}
Output:
F:\java>javac test.java F:\java>java test White Nocolor Black Nocolor Red White Yellow
Example of Throws: add(int index, E element) Method
IndexOutOfBoundsException - if the index is out of range (index < 0 || index > size()).
Let
int index = set(-8);
in the above example.
Output:
White Nocolor Black Nocolor Red White Yellow Exception in thread "main" java.lang.IndexOutOfBoundsEx ception: Index: 7, Size: 7 at java.util.ArrayList.rangeCheck(ArrayList.jav a:635) at java.util.ArrayList.get(ArrayList.java:411) at test.main(test.java:23)
Java Code Editor:
Previous:add Method (Object)
Next:remove(int index) Method