Java ArrayList.removeIf() Method
public boolean removeIf(Predicate<? super E> filter)
The removeIf() method is used to remove all of the elements of this collection that satisfy the given predicate. Errors or runtime exceptions are thrown during iteration or by the predicate are relayed to the caller.
Package: java.util
Java Platform: Java SE 8
Syntax:
removeIf(Predicate<? super E> filter)
Parameters:
Name | Description |
---|---|
filter | A predicate which returns true for elements to be removed |
Return Value:
true if any elements were removed
Pictorial presentation of ArrayList.removeIf() Method
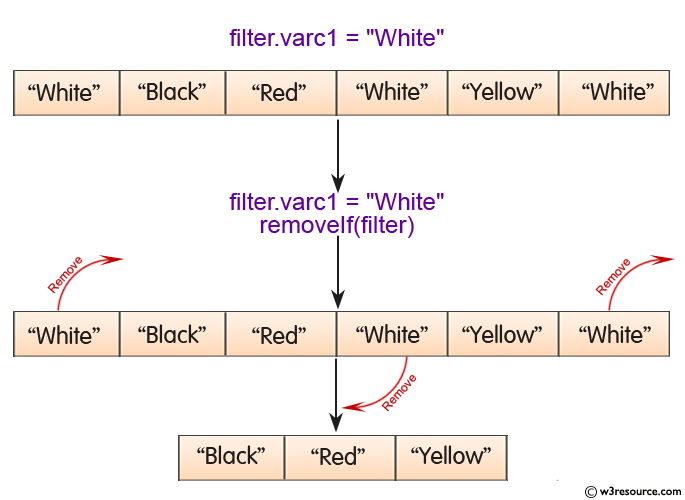
Example: Java ArrayList.removeIf() Method
The following example removes all of the elements of this collection that satisfy the given predicate.
import java.util.function.*;
class SamplePredicate<T> implements Predicate<T>{
T varc1;
public boolean test(T varc){
if(varc1.equals(varc)){
return true;
}
return false;
}
}
import java.util.*;
public class test {
public static void main(String[] args) {
ArrayList<String> color_list;
SamplePredicate<String> filter;
color_list = new ArrayList<> ();
filter = new SamplePredicate<> ();
filter.varc1 = "White";
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
color_list.add("White");
System.out.println("List of Colors");
System.out.println(color_list);
// Remove all White colors from color_list
color_list.removeIf(filter);
System.out.println("Color list, after removing White colors :");
System.out.println(color_list);
}
}
Output:
F:\java>javac test.java F:\java>java test List of Colors [White, Black, Red, White, Yellow, White] Color list, after removing White colors : [Black, Red, Yellow]
Previous:spliterator
Next:replaceAll