Java ArrayList.removeAll() Method
public boolean removeAll(Collection<?> c)
The removeAll() method is used to remove all the elements from a list that are contained in the specified collection.
Package: java.util
Java Platform: Java SE 8
Syntax:
removeAll(Collection<?> c)
Parameters:
Name | Description |
---|---|
c | collection containing elements to be removed from this list |
Return Value:
true if this list changed as a result of the call
Throws:
- ClassCastException - if the class of an element of this list is incompatible with the specified collection (optional)
- NullPointerException - if this list contains a null element and the specified collection does not permit null elements (optional), or if the specified collection is null
Pictorial presentation of ArrayList.removeAll() Method
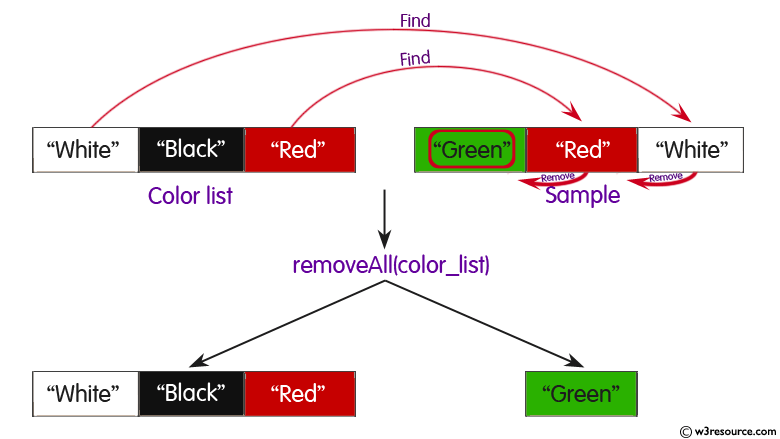
Example: ArrayList.removeAll() Method
The following example the removeAll() method is used to remove all the elements from a list that are contained in the specified collection.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list
ArrayList<String> color_list = new ArrayList<String>();
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
// create an empty array sample with an initial capacity
ArrayList<String> sample = new ArrayList<String>();
// use add() method to add values in the list
sample.add("Green");
sample.add("Red");
sample.add("White");
// remove all elements from second list if it exists in first list
sample.removeAll(color_list);
System.out.println("First List :"+ color_list);
System.out.println("Second List :"+ sample);
}
}
Output:
F:\java>javac test.java F:\java>java test First List :[White, Black, Red] Second List :[Green]
Previous:removeRange Method
Next:retainAll Method