Java ArrayList.addAll (Collection<? extends E> c)() Method
public boolean addAll(Collection<? extends E> c)
This method is used to append all of the elements in the specified collection to the end of this list. The behavior of this operation is undefined if the specified collection is modified while the operation is in progress.
Package: java.util
Java Platform: Java SE 8
Syntax:
addAll(Collection<? extends E> c)
Parameters:
Name | Description |
---|---|
c | Collection containing elements to be added to this list |
Return Value:
true if this list changed as a result of the call.
Return Value Type: boolean
Throws:
NullPointerException - if the specified collection is null
Pictorial presentation of ArrayList.addAll() Method
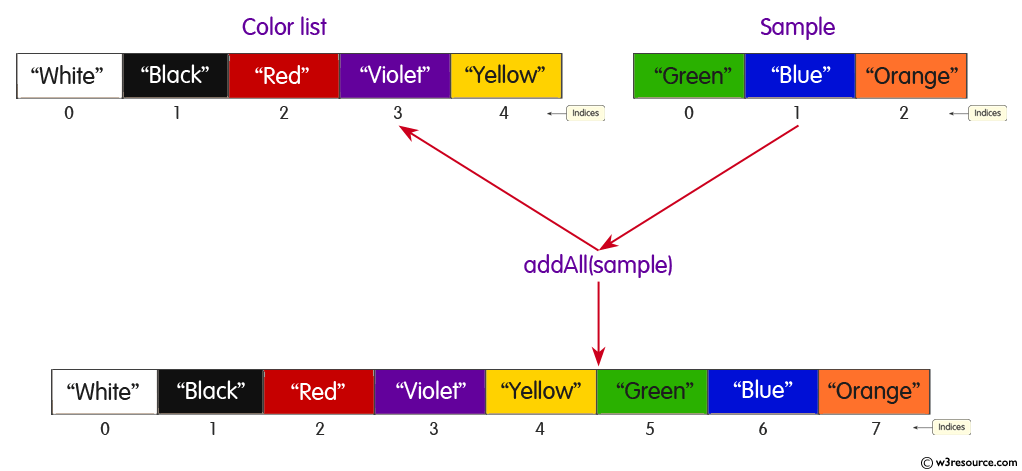
Example: ArrayList.addAll(Collection<? extends E> c) Method
The following example fills an ArrayList object with the contents of another ArrayList object .
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(4);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
// Print out the colors in the ArrayList
System.out.println("****Color list****");
for (int i = 0; i < 4; i++)
{
System.out.println(color_list.get(i).toString());
}
// create an empty array sample with an initial capacity
ArrayList<String> sample = new ArrayList<String>(3);
sample.add("Yellow");
sample.add("Red");
sample.add("White");
// Now add sample with color_list
color_list.addAll(sample);
//New size of the list
System.out.println("New size of the list is: " + color_list.size());
// Print out the colors
System.out.println("****Color list****");
for (int i = 0; i < 7; i++)
{
System.out.println(color_list.get(i).toString());
}
}
}
Output:
F:\java>javac test.java F:\java>java test ****Color list**** White Black Red White New size of the list is: 7 ****Color list**** White Black Red White Yellow Red White
Example of Throws: addAll(Collection&? extends E> c) Method
NullPointerException - if the specified collection is null.
Let
(int i = 0; i < 8; i++)
in the above example.
Output:
****Color list**** White Black Red White New size of the list is: 7 ****Color list**** White Black Red White Yellow Red White Exception in thread "main" java.lang.IndexOutOfBoundsEx ception: Index: 7, Size: 7 at java.util.ArrayList.rangeCheck(ArrayList.jav a:635) at java.util.ArrayList.get(ArrayList.java:411) at test.main(test.java:38)
Java Code Editor:
Previous:clear Method
Next:removeRange Method