Java ArrayList.sort() Method
public void sort(Comparator<? super E> c)
The sort() method is used to sort a list according to the order induced by the specified Comparator.
All elements in this list must be mutually comparable using the specified comparator (that is, c.compare(e1, e2) must not throw a ClassCastException for any elements e1 and e2 in the list).
If the specified comparator is null then all elements in this list must implement the Comparable interface and the elements' natural ordering should be used.
This list must be modifiable, but need not be resizable.
Package: java.util
Java Platform: Java SE 8
Syntax:
sort(Comparator<? super E> c)
Parameters:
Name | Description |
---|---|
c | The Comparator used to compare list elements. A null value indicates that the elements' natural ordering should be used |
Return Value:
This method does not return any value.
Pictorial presentation of ArrayList.size() Method
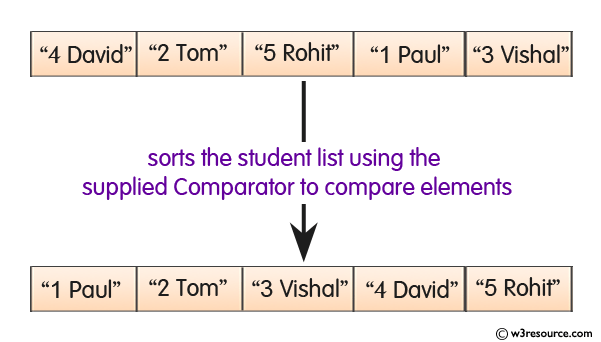
Example: Java ArrayList.sort() Method
The following example sorts the student list using the supplied Comparator to compare elements.
class Student{
String student_name;
Integer id;
Student(int id, String student_name){
this.id = id;
this.student_name = student_name;
}
public String toString(){
return id + " " + student_name;
}
}
import java.util.Comparator;
class StudentIdComparator implements Comparator<Student>{
public int compare(Student e1, Student e2){
return e1.id.compareTo(e2.id);
}
public String toString(){
return "StudentIdComparator";
}
}
import java.util.*;
public class test {
public static void main(String[] args) {
ArrayList<Student> myList;
StudentIdComparator comparator;
myList = new ArrayList<> ();
comparator = new StudentIdComparator();
myList.add(new Student(4, "David"));
myList.add(new Student(2, "Tom"));
myList.add(new Student(5, "Rohit"));
myList.add(new Student(1, "Paul"));
myList.add(new Student(3, "Vishal"));
System.out.println("Elements Before Sorting");
System.out.println(myList);
myList.sort(comparator);
System.out.println("Elements After Sorting");
System.out.println(myList);
}
}
Output:
F:\java>javac test.java F:\java>java test Elements Before Sorting [4 David, 2 Tom, 5 Rohit, 1 Paul, 3 Vishal] Elements After Sorting [1 Paul, 2 Tom, 3 Vishal, 4 David, 5 Rohit]
Previous:replaceAll
Next:Date Class Methods
Home