Java ArrayList.remove(Object o) Method
public boolean remove(Object o)
This method is used to remove the first occurrence of the specified element from this list, if it is present. If the element is not present within the list, it is unchanged.
Package: java.util
Java Platform: Java SE 8
Syntax:
remove(Object o)
Parameters:
Name | Description |
---|---|
o | Element to be removed from this list, if present |
Return Value:
true if this list contained the specified element.
Return Value Type: boolean
Pictorial presentation of ArrayList.remove(Object o) Method
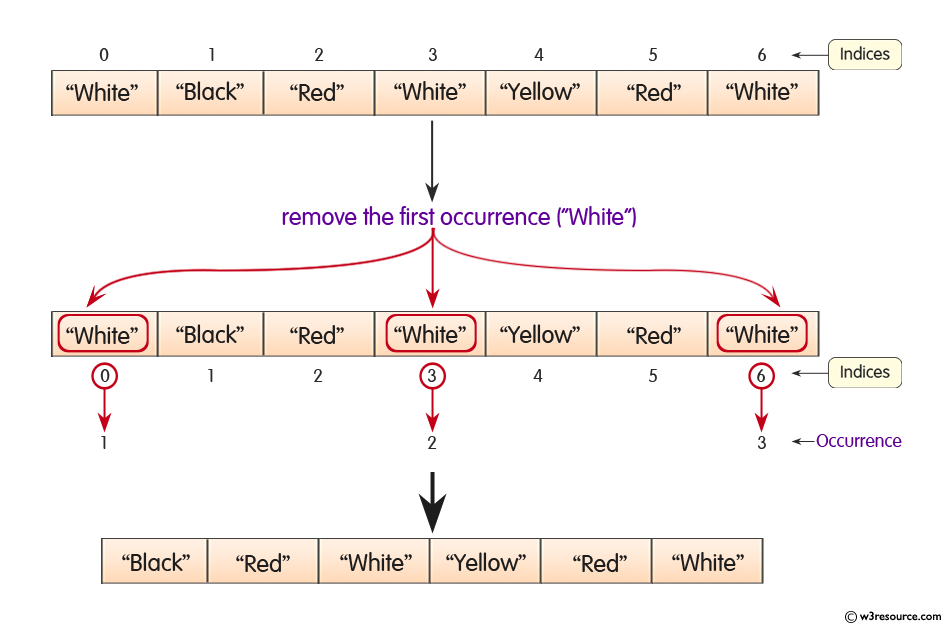
Example: ArrayList.remove(Object o) Method
The following example creates an ArrayList with a capacity of 7 elements ('White' and 'Red 'colors added twice in the list). After adding 7 colors we have removed the first occurrence of "Red" and "White" colors.
import java.util.*;
public class test {
public static void main(String[] args) {
// create an empty array list with an initial capacity
ArrayList<String> color_list = new ArrayList<String>(7);
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
color_list.add("Red");
color_list.add("White");
// Print out the colors in the ArrayList
System.out.println("****Color list****");
for (int i = 0; i < 7; i++)
{
System.out.println(color_list.get(i).toString());
}
// Removes first occurrence of "Red" and "White" colors
color_list.remove("White");
color_list.remove("Red");
// Fresh Color list
System.out.println("****Fresh Color list****");
for (int i = 0; i < 5; i++)
{
System.out.println(color_list.get(i).toString());
}
}
}
Output:
F:\java>javac test.java F:\java>java test ****Color list**** White Black Red White Yellow Red White ****Fresh Color list**** Black White Yellow Red White
Java Code Editor:
Previous:remove(int index) Method
Next:clear Method
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-tutorial/arraylist/arraylist_remove-object-o.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics