Java ArrayList.replaceAll() Method
public void replaceAll(UnaryOperator<E> operator)
The replaceAll() method is used to replace each element of this list with the result of applying the operator to that element.
Errors or runtime exceptions are thrown by the operator are relayed to the caller.
Package: java.util
Java Platform: Java SE 8
Syntax:
replaceAll(UnaryOperator<E> operator)
Parameters:
Name | Description |
---|---|
operator | The operator to apply to each element. |
Return Value:
This method does not return any value.
Pictorial presentation of ArrayList.replaceAll() Method
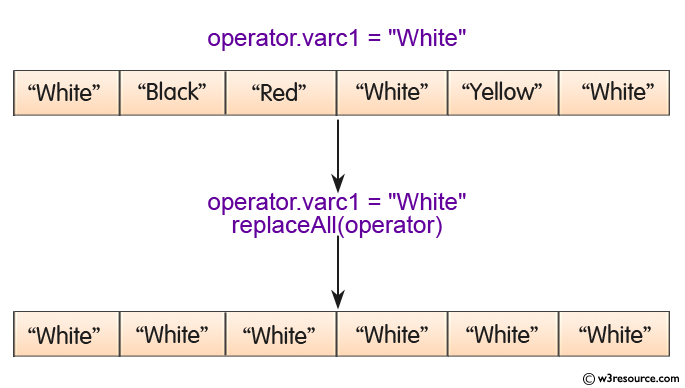
Example: Java ArrayList.replaceAll() Method
The following example removes all of the elements of this collection that satisfy the given predicate.
import java.util.function.*;
class MyOperator<T> implements UnaryOperator<T>{
T varc1;
public T apply(T varc){
return varc1;
}
}
import java.util.*;
public class test {
public static void main(String[] args) {
ArrayList<String> color_list;
MyOperator<String> operator;
color_list = new ArrayList<> ();
operator = new MyOperator<> ();
operator.varc1 = "White";
// use add() method to add values in the list
color_list.add("White");
color_list.add("Black");
color_list.add("Red");
color_list.add("White");
color_list.add("Yellow");
color_list.add("White");
System.out.println("List of Colors");
System.out.println(color_list);
// Replace all colors with White color
color_list.replaceAll(operator);
System.out.println("Color list, after replacing all colors with White color :");
System.out.println(color_list);
}
}
Output:
F:\java>javac test.java F:\java>java test List of Colors [White, Black, Red, White, Yellow, White] Color list, after replacing all colors with White color : [White, White, White, White, White, White]