JavaScript: HTML Form validation - checking for all numbers
Checking for all numbers
Sometimes situations arise (input a phone number, zip code or credit card number) when the user should fill a single or more than one fields with numbers (0-9) in an HTML form. You can write JavaScript scripts to check the following validations.
- To check for all numbers in a field.
- An integer with an optional leading plus(+) or minus(-) sign.
Following code blocks contain actual codes for the said validations. We have kept the CSS code part common for all the validations.
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px soild silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
To check for all numbers in a field
To get a string contains only numbers (0-9) we use a regular expression (/^[0-9]+$/) which allows only numbers. Next, the match() method of the string object is used to match the said regular expression against the input value. Here is the complete web document.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking all numbers</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input your registration number and submit</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li class="rq">*Enter numbers only.</li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="allnumeric(document.form1.text1)" /></li>
<li> </li>
</ul>
</form>
</div>
<script src="all-numbers.js"></script>
</body>
</html>
JavaScript Code
function allnumeric(inputtxt)
{
var numbers = /^[0-9]+$/;
if(inputtxt.value.match(numbers))
{
alert('Your Registration number has accepted....');
document.form1.text1.focus();
return true;
}
else
{
alert('Please input numeric characters only');
document.form1.text1.focus();
return false;
}
}
Flowchart:
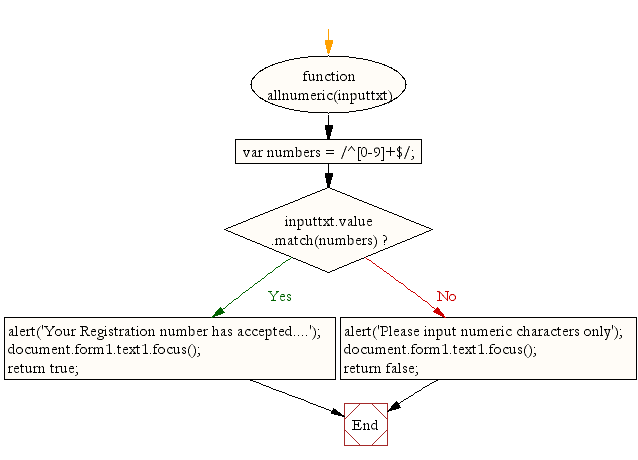
View the example in the browser
An integer with an optional leading plus(+) or minus(-) sign
To get a string contains only numbers (0-9) with a optional + or - sign (e.g. +1223, -4567, 1223, 4567) we use the regular expression (/^[-+]?[0-9]+$/). Next, the match() method of the string object is used to match the said regular expression against the input value. Here is the complete web document.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - An integer with an optional leading plus(+) or minus(-) sign</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input your registration number and submit</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li class="rq">*Enter numbers only.</li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="allnumericplusminus(document.form1.text1)" /></li>
<li> </li>
</ul>
</form>
</div>
<script src="all-numbers-with-plus-minus.js"></script>
</body>
</html>
JavaScript Code
function allnumericplusminus(inputtxt)
{
var numbers = /^[-+]?[0-9]+$/;
if(inputtxt.value.match(numbers))
{
alert('Correct...Try another');
document.form1.text1.focus();
return true;
}
else
{
alert('Please input correct format');
document.form1.text1.focus();
return false;
}
}
Flowchart:
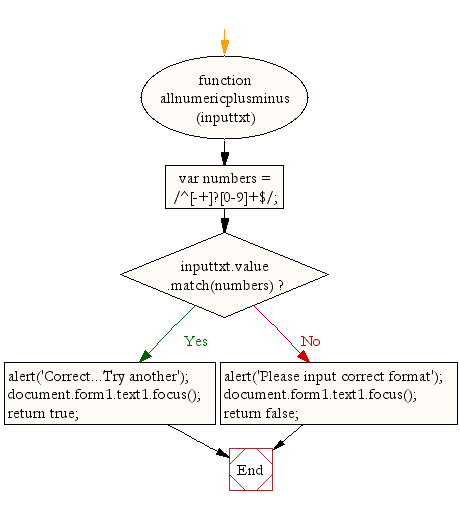
View the example in the browser
file_download Download the validation code from here.
Other JavaScript Validation :
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript: HTML Form validation - checking for all letters
Next: JavaScript: HTML Form validation - checking for Floating point numbers
Test your Programming skills with w3resource's quiz.