JavaScript: HTML Form - email validation
Email validation
Validating email is a very important point while validating an HTML form. In this page we have discussed how to validate an email using JavaScript :
An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is personal_info@domain. The length of the personal_info part may be up to 64 characters long and domain name may be up to 253 characters.
The personal_info part contains the following ASCII characters.
- Uppercase (A-Z) and lowercase (a-z) English letters.
- Digits (0-9).
- Characters ! # $ % & ' * + - / = ? ^ _ ` { | } ~
- Character . ( period, dot or fullstop) provided that it is not the first or last character and it will not come one after the other.
The domain name [for example com, org, net, in, us, info] part contains letters, digits, hyphens, and dots.
Example of valid email id
Example of invalid email id
- mysite.ourearth.com [@ is not present]
- [email protected] [ tld (Top Level domain) can not start with dot "." ]
- @you.me.net [ No character before @ ]
- [email protected] [ ".b" is not a valid tld ]
- [email protected] [ tld can not start with dot "." ]
- [email protected] [ an email should not be start with "." ]
- mysite()*@gmail.com [ here the regular expression only allows character, digit, underscore, and dash ]
- [email protected] [double dots are not allowed]
JavaScript code to validate an email id
function ValidateEmail(mail)
{
if (/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/.test(myForm.emailAddr.value))
{
return (true)
}
alert("You have entered an invalid email address!")
return (false)
}
To get a valid email id we use a regular expression /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/. According to http://tools.ietf.org/html/rfc3696#page-5 ! # $ % & ‘ * + – / = ? ^ ` . { | } ~ characters are legal in the local part of an e-mail address but in the above regular expression those characters are filtered out. You can modify or rewrite the said regular expression.
Flowchart :
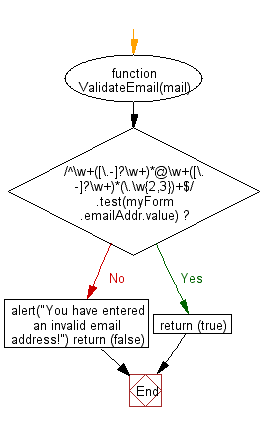
Regular Expression Pattern
/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/
Let apply the above JavaScript function in an HTML form.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking email</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input an email and Submit</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li class="submit"><input type="submit" name="submit" value="Submit" onclick="ValidateEmail(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="email-validation.js"></script>
</body>
</html>
JavaScript Code
function ValidateEmail(inputText)
{
var mailformat = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
if(inputText.value.match(mailformat))
{
alert("Valid email address!");
document.form1.text1.focus();
return true;
}
else
{
alert("You have entered an invalid email address!");
document.form1.text1.focus();
return false;
}
}
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px soild silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
View the Javascript email validation in the browser
RFC 2822 standard email validation
Regular Expression Pattern (Ref: https://bit.ly/33cv2vn):
/(?:[a-z0-9!#$%&'*+/=?^_`{|}~-]+(?:\.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*|"(?:[\x01-\x08\x0b\x0c\x0e-\x1f\x21\x23-\x5b\x5d-\x7f]| \\[\x01-\x09\x0b\x0c\x0e-\x7f])*")@(?:(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?\.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?| \[(?:(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?|[a-z0-9-]*[a-z0-9]: (?:[\x01-\x08\x0b\x0c\x0e-\x1f\x21-\x5a\x53-\x7f]|\\[\x01-\x09\x0b\x0c\x0e-\x7f])+)\])/
View the Javascript email validation (RFC 2822) in the browser
You can use the following email addresses to test the said Regular Expression:
Ref: https://bit.ly/35g81dj
List of Valid Email Addresses
- [email protected]
- [email protected]
- [email protected]
- [email protected]
- [email protected]
- email@[123.123.123.123]
- "email"@example.com
- [email protected]
- [email protected]
- [email protected]
- [email protected]
- [email protected]
- [email protected]
- [email protected]
List of Strange Valid Email Addresses
- much.”more\ unusual”@example.com
- very.unusual.”@”[email protected]
- very.”(),:;<>[]”.VERY.”very@\\ "very”[email protected]
file_download Download the validation code from here.
Other JavaScript Validation:
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript: HTML Form - restricting the length
Next: JavaScript: HTML Form - Date validation
Test your Programming skills with w3resource's quiz.