JavaScript: HTML Form - Date validation
Date validation
It is very important to validate the data supplied by the user through a form before you process it. Among various kind of data validation, validation of date is one.
In this tutorial, we discussed how you can perform JavaScript date validation in
1.
dd/mm/yyyy or dd-mm-yyyy format.
2.
mm/dd/yyyy or mm-dd-yyyy format.
In the following examples, a JavaScript function is used to check a valid date format against a regular expression. Later we take each part of the string supplied by user (i.e. dd, mm and yyyy) and check whether dd is a valid date, mm is a valid month or yyyy is a valid year. We have also checked the leap year factor for the month of February. We have used "/" and "-" character as a separator within the date format but you are free to change that separator the way you want.
Validate dd/mm/yyyy or dd-mm-yyyy format
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking date</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input a valid date [dd/mm/yyyy or dd-mm-yyyy format]</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li class="submit"><input type="submit" name="submit" value="Submit" onclick="validatedate(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="ddmmyyyy-validation.js"></script>
</body>
</html>
JavaScript Code
function validatedate(inputText)
{
var dateformat = /^(0?[1-9]|[12][0-9]|3[01])[\/\-](0?[1-9]|1[012])[\/\-]\d{4}$/;
// Match the date format through regular expression
if(inputText.value.match(dateformat))
{
document.form1.text1.focus();
//Test which seperator is used '/' or '-'
var opera1 = inputText.value.split('/');
var opera2 = inputText.value.split('-');
lopera1 = opera1.length;
lopera2 = opera2.length;
// Extract the string into month, date and year
if (lopera1>1)
{
var pdate = inputText.value.split('/');
}
else if (lopera2>1)
{
var pdate = inputText.value.split('-');
}
var dd = parseInt(pdate[0]);
var mm = parseInt(pdate[1]);
var yy = parseInt(pdate[2]);
// Create list of days of a month [assume there is no leap year by default]
var ListofDays = [31,28,31,30,31,30,31,31,30,31,30,31];
if (mm==1 || mm>2)
{
if (dd>ListofDays[mm-1])
{
alert('Invalid date format!');
return false;
}
}
if (mm==2)
{
var lyear = false;
if ( (!(yy % 4) && yy % 100) || !(yy % 400))
{
lyear = true;
}
if ((lyear==false) && (dd>=29))
{
alert('Invalid date format!');
return false;
}
if ((lyear==true) && (dd>29))
{
alert('Invalid date format!');
return false;
}
}
}
else
{
alert("Invalid date format!");
document.form1.text1.focus();
return false;
}
}
Flowchart:
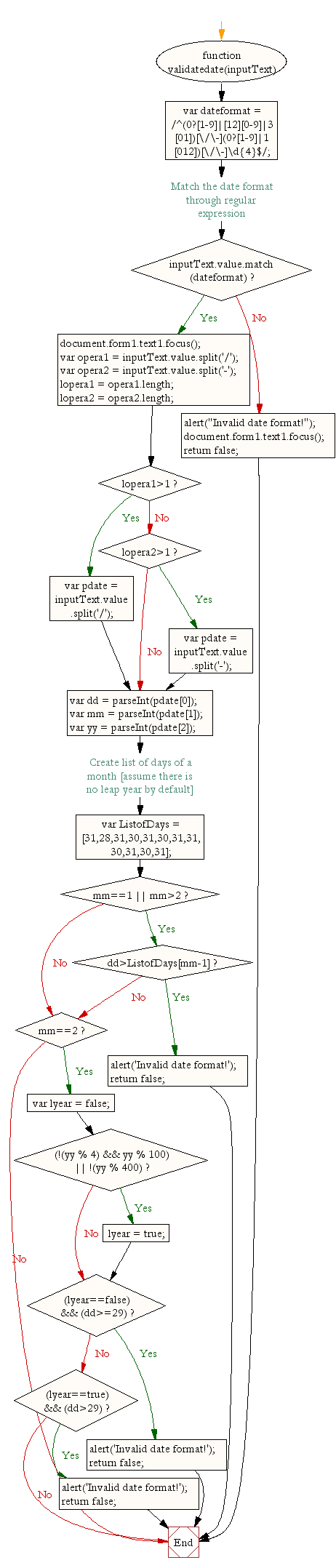
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px soild silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
View the example in the browser
Validate mm/dd/yyyy or mm-dd-yyyy format
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking date [mm/dd/yyyy or mm-dd-yyyy format]</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input a valid date [mm/dd/yyyy or mm-dd-yyyy format]</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li class="submit"><input type="submit" name="submit" value="Submit" onclick="validatedate(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="mmddyyyy-validation.js"></script>
</body>
</html>
JavaScript Code
function validatedate(inputText)
{
var dateformat = /^(0?[1-9]|1[012])[\/\-](0?[1-9]|[12][0-9]|3[01])[\/\-]\d{4}$/;
// Match the date format through regular expression
if(inputText.value.match(dateformat))
{
document.form1.text1.focus();
//Test which seperator is used '/' or '-'
var opera1 = inputText.value.split('/');
var opera2 = inputText.value.split('-');
lopera1 = opera1.length;
lopera2 = opera2.length;
// Extract the string into month, date and year
if (lopera1>1)
{
var pdate = inputText.value.split('/');
}
else if (lopera2>1)
{
var pdate = inputText.value.split('-');
}
var mm = parseInt(pdate[0]);
var dd = parseInt(pdate[1]);
var yy = parseInt(pdate[2]);
// Create list of days of a month [assume there is no leap year by default]
var ListofDays = [31,28,31,30,31,30,31,31,30,31,30,31];
if (mm==1 || mm>2)
{
if (dd>ListofDays[mm-1])
{
alert('Invalid date format!');
return false;
}
}
if (mm==2)
{
var lyear = false;
if ( (!(yy % 4) && yy % 100) || !(yy % 400))
{
lyear = true;
}
if ((lyear==false) && (dd>=29))
{
alert('Invalid date format!');
return false;
}
if ((lyear==true) && (dd>29))
{
alert('Invalid date format!');
return false;
}
}
}
else
{
alert("Invalid date format!");
document.form1.text1.focus();
return false;
}
}
Note: CSS code is similar for both examples.
View the example in the browse
file_download Download the validation code from here.
Other JavaScript Validation:
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript: HTML Form - email validation
Next: JavaScript Form Validation using a Sample Registration Form
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics