Cypress Command Line: Run Tests and Manage Configuration
In this tutorial you will learn how to run Cypress from the command line, we will show you how to specify the spec files that you want to run. We will show you how to launch other browsers and how to record your tests to the Dashboard.
Installation
This guide will assume that you have already read our guide on installing Cypress and you have installed Cypress as an npm module. Once you are done installing, you will be able to execute all the commands that is contained in this tutorial.
How to run the commands?
We have removed the full path to the cypress executable in each command’s documentation for the sake of brevity.
If you want to run a command, you will need to prefix each of the commands in order to properly locate the cypress executable.
'''$(npm bin)/cypress run'''
Or
'''./node_modules/.bin/cypress run'''
Or
'''npx cypress run'''
The last option requires [email protected] or greater.
In most cases, you will find it easier to add the cypress command to the scripts object in your package.json file and then call it from an npm run script.
Whenever you call a command using npm run, you will have to pass the commands arguments using the – string. For instance, if your package.json file has the following command:
'''{
"scripts": {
"cy:run": "cypress run"
}
}'''
To run the tests from a single test file and record the result on the dashboard, the command will be:
'''npm run cy:run -- --record --spec "cypress/integration/sample-spec.js"'''
Commands
cypress run
When you run the Cypress tests to completion, cypress run will run the tests heedlessly in the Electron browser by default.
'''cypress run [options]'''
Option | Description |
---|---|
--browser, -b | This will run Cypress in the browser with the given name. If you supply the file filesystem path, Cypress will then attempt to use the browser at that path. |
--ci-build-id | Used to specify a unique identifier for a run to enable grouping or parallelization. |
--config, -c | Used to specify a configuration. |
--config-file, -C | Used to specify a configuration file |
--env, -e | Used to specify the environment variables |
--group | This is used to group recorded tests together under a single run. |
--headed | This is used to display the browser rather than running headlessly which is the default for Firefox and Chromium-based browsers. |
--headless | This will hide the browser instead of running headed, this is the default for Electron. |
--help, -h | This command will output usage information |
--key, -k | Used to specify your secret key |
--no-exit | The –no-exit option is used to keep the Cypress Test Runner open after the tests in a spec file run. |
--parallel | This is used to run the recorded specs in parallel across multiple machines. |
--port, -p | This is used to override default port. |
--project, -p | This is the path a specific project. |
--quiet, -q | If you pass this option, the Cypress output will not be printed to stdout. It will only print the output from the configured Mocha reporter. |
--record | This option determines whether a test will be recorded to the Dashboard Service. |
--reporter, -r | This is used to specify a Mocha reporter. |
--reporter-options, -o | This option is used to specify Mocha reporter options |
--spec, -s | This is used to specify the spec files that you want to run. |
--tag, -t | This is used to identify a tag or tags. |
cypress run --browser <browser-name-or-path>
'''cypress run --browser chrome'''
You can set the “browser” argument to either chrome, chromium, edge, electron or firefox to launch a browser that is detected by your system. Cypress will automatically try to find the installed browser for you.
If you want to launch a non-stable browser, you will need to add a colon and then the desired release channel. For instance, in order to launch Chrome Canary, you will need to run chrome:canary
you can equally choose a browser by supplying a path as shown below:
'''cypress run --browser /usr/bin/chromium'''
cypress run --ci-build-id <id>
This value can be detected automatically for most CI providers and is unnecessary to define unless Cypress is unable to determine it.
This is typically defined as an environment variable within your CI provider, this define a unique “build” or “run”.
'''cypress run --ci-build-id BUILD_NUMBER'''
This is only valid when you are providing a -group or -parallel flag.
cypress run --config <config>
This is used to set configuration values. Separate multiple values with a comma. The values you set here are override any values set in your configuration file.
'''cypress run --config pageLoadTimeout=100000,watchForFileChanges=false'''
cypress run --config-file <config-file>
This enables you to specify a path to a JSON file where the values are set. The default file is cypress.json .
You can pass false in order to disable the use of configuration file entirely.
'''cypress run --config-file false'''
cypress run --env <env>
This is used to set Cypress environment variables.
'''cypress run --env host=api.dev.local'''
you can pass several variables using commas and no spaces. Numbers will be converted to strings automatically. Here is an example:
'''cypress run --env host=api.dev.local,port=4222'''
You can pass an object as a JSON in a string.
'''cypress run --env flags='{"feature-a":true,"feature-b":false}'''
cypress run –group <name>
It is also possible to add multiple groups to the same run by passing a different name. This can help you to distinguish groups of specs from each other.
'''cypress run --group admin-tests --spec 'cypress/integration/admin/**/*''''
'''cypress run --group user-tests --spec 'cypress/integration/user/**/*''''
it may be necessary to always specify the –ci-build-id.
cypress run --headed
Cypress runs the tests in Electron heedlessly by default.
When you pass –headed, it will force Electron to be shown. This will match how you run Electron via cypress open.
cypress run --headless
Cypress will run the tests in Chrome and Firefox headed by default. Whenever you pass the --headless option, it will force the browser to be hidden.
'''cypress run --headless --browser chrome'''
cypress run --no-exit
If you want to prevent the Cypress Test Runner from exiting after running the tests in a spec file, you will need to use --no-exit.
You can also pass --headed --no-exit, so as to view the command log or to have access to the developer tools after a spec has run.
cypress run --headed --no-exit
cypress run --parallel
This command will run the recorded specs in parallel across multiple machines.
cypress run --record --parallel
Additionally, you can pass a --group flag so that the test will show up as a named group.
'''cypress run --record --parallel --group e2e-staging-specs'''
cypress run --port <port>
'''cypress run --port 8080'''
cypress run --project
This option is used to run the project from a specific project path.
cypress run --record --key <record-key>
You can record he video of tests that is running once you have set up your project to record. This can be done by running the command below:
'''cypress run --record -key'''
if you have set the Record Key as the environment variable CYPRESS_RECORD_KEY, you can omit the --key flag.
If you are running your test within a Continuous Integration, you can set the environment variable as shown below:
'''export CYPRESS_RECORD_KEY=abc-key-123'''
With this you can omit the --key flag, and then the command will thus be:
'''cypress run -record'''
cypress run --reporter <reporter>
You can specify a specific Mocha reporter when you are testing.
'''cypress run --reporter json'''
you can also specify a reporter option by using the --reporter-options <reporter-option> flag.
'''cypress run --reporter junit --reporter-options mochaFile=result.xml,toConsole=true'''
cypress run --spec <spec>
This command below will run tests by specifying a single test file to run instead of all tests:
'''cypress run --spec "cypress/integration/examples/actions.spec.js"'''
This command below will run the tests within the folder that matches the glob (it is recommended that you use double quotes).
'''cypress run --spec "cypress/integration/login/**/*"'''
The command below will run tests specifying multiple test files to run.
'''cypress run --spec "cypress/integration/examples/actions.spec.js,cypress/integration/examples/files.spec.js"'''
cypress run --tag <tag>
This command will add a tag or tags to the recorded run. It can be used to help identify separate run when displayed in the Dashboard.
'''cypress run --record --tag "staging"'''
You can also give a run, multiple tags as shown below:
'''cypress run --record --tag "production,nightly"'''
The Dashboard will display any tags that is sent with the appropriate run.
cypress open
This command will open Test Runner.
'''cypress open [options]'''
Options:
The options that are passed to cypress open will be applied automatically to the project you open. These will persist on all projects till you quit the Cypress Test Runner. These options will equally override values in your configuration file, this will be the cypress.json file by default.
Option | Description |
---|---|
--browser, -b | Path to a custom browser to be added to the list of available browsers in Cypress |
--config, -c | Specify configuration |
--config-file, -C | Specify configuration file |
--detached, -d | Open Cypress in detached mode |
--env, -e | Specify environment variables |
--global | Run in global mode |
--help, -h | Output usage information |
--port, -p | Override default port |
--project, -P | Path to a specific project |
cypress info
This command will print information about Cypress and the current environment such as:
- A list of the browsers that Cypress has detected on the machine.
- Any environment variable that has control over the proxy configuration
- Any environment variable that starts with the CYPRESS prefix (the sensitive variables like the record key will be masked for security)
- The location where the run-time data will be stored.
- The location where Cypress’s binary is cached.
- The operating system’s information.
- The system memory, including free space.
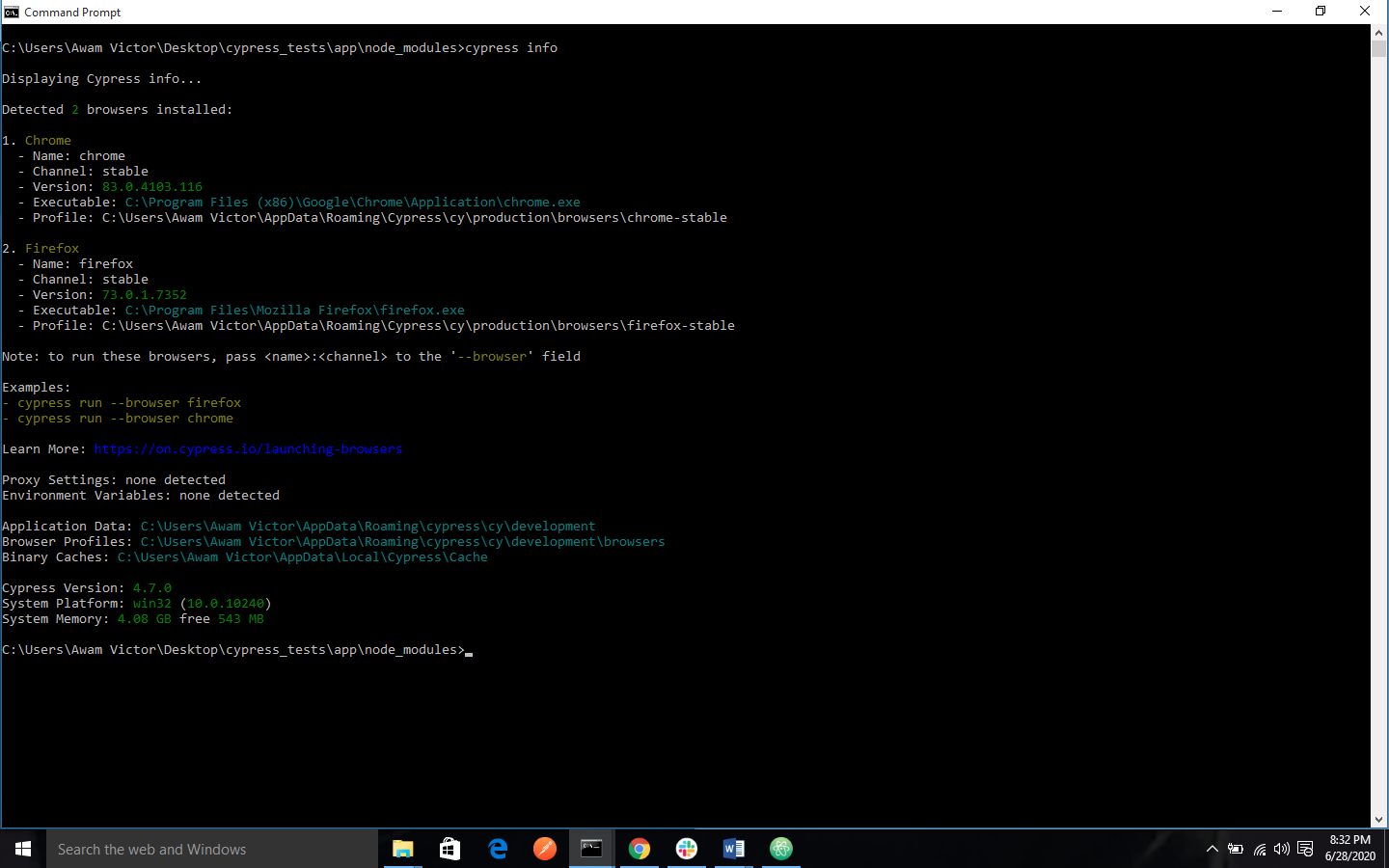
You can set DEBUG environment variable to cypress:launcher when running cypress info to troubleshoot browser detection.
cypress verify
This command will verify that Cypress is installed correctly and is executable.
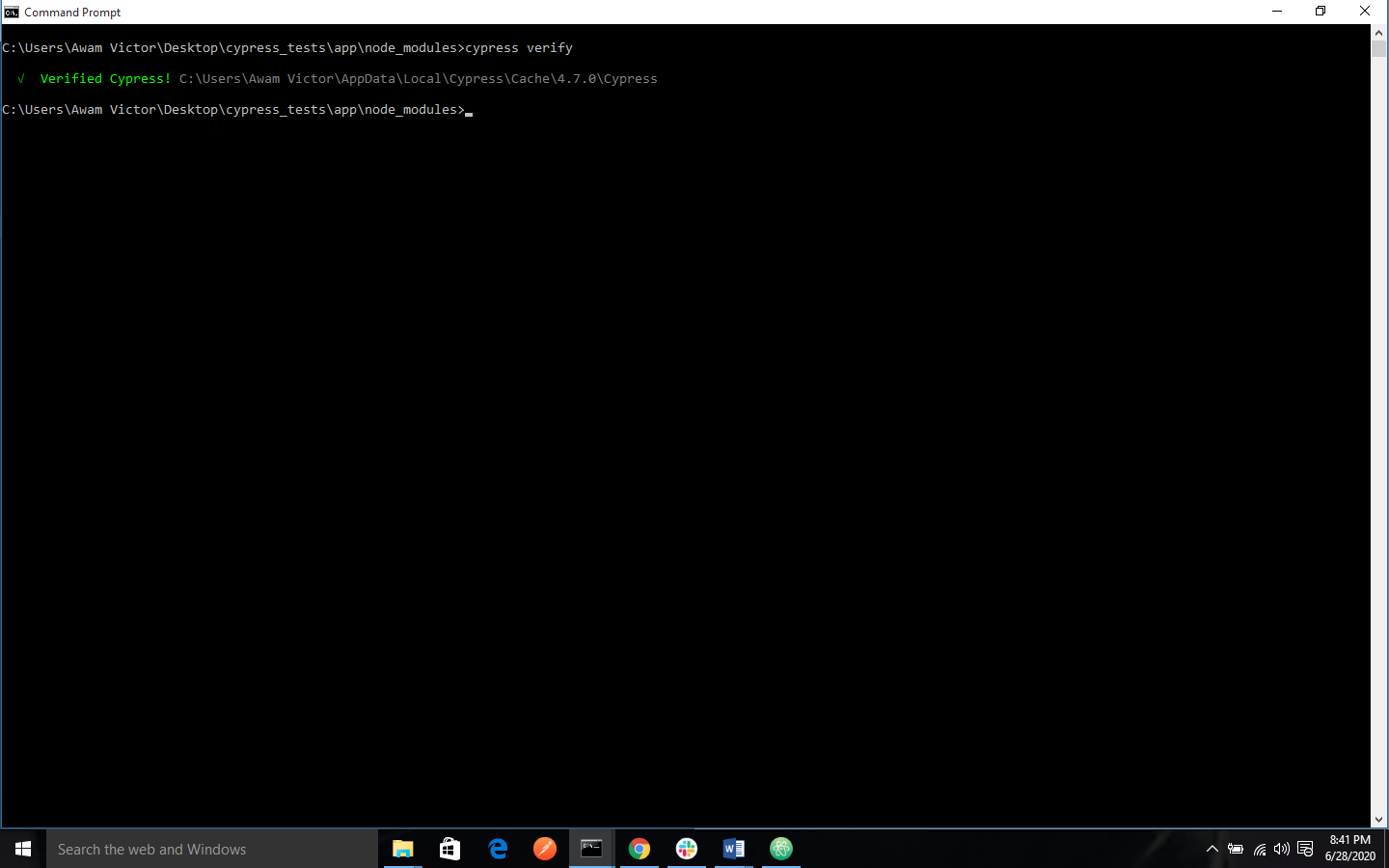
cypress version
This command will output the versions of the Cypress binary application that is installed as well as the npm module.
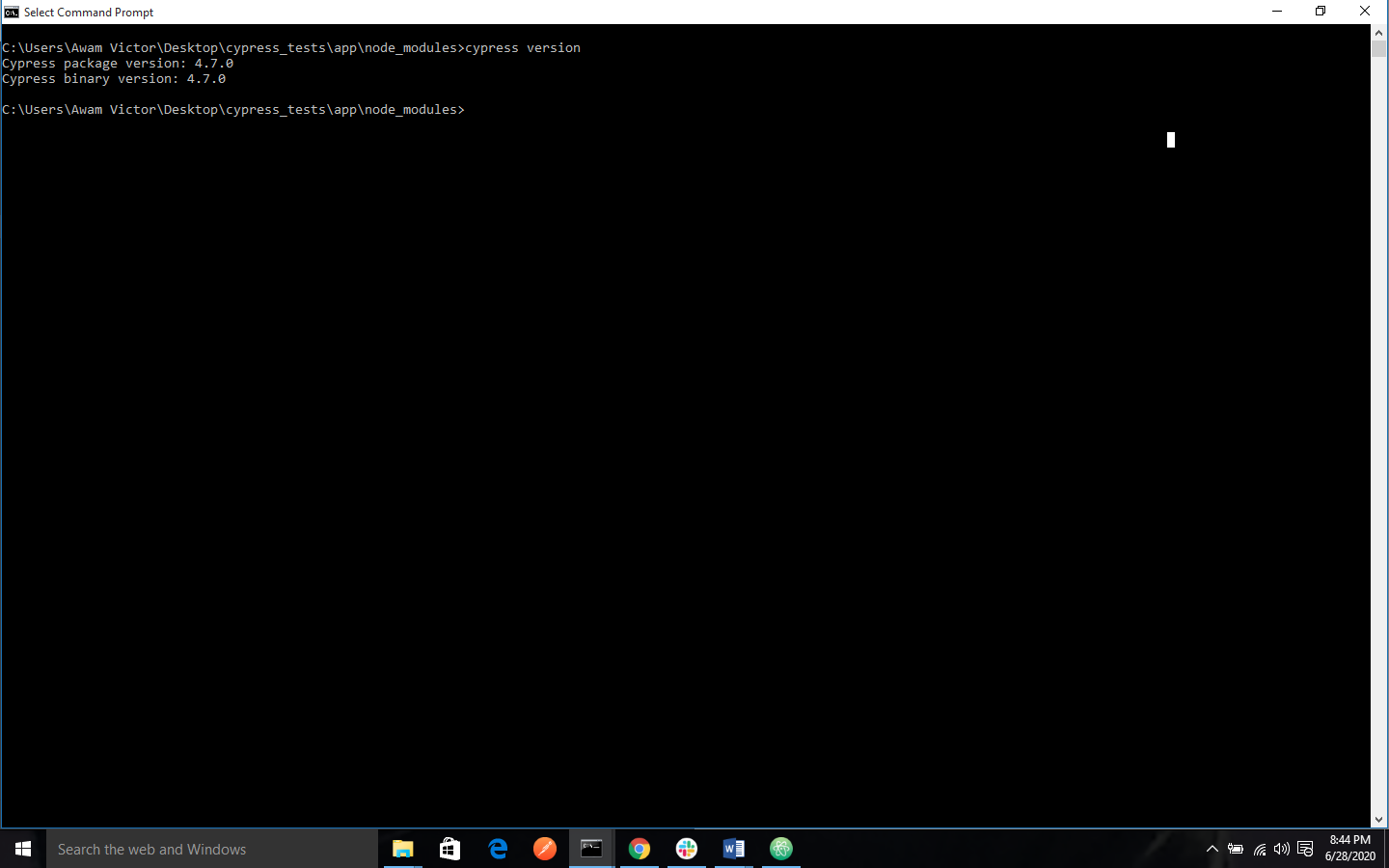
cypress cache [command]
This represents the list of commands used to manage the global Cypress cache. The cache applies to all the installs of Cypress across your machine.
cypress cache path
This will print the path to the Cypress cache folder.
cypress cache list
This will print all the installed versions of Cypress.
cypress cache clear
The cypress cache clear command will clear the contents of the Cypress cache. It is useful when you want to clear out all the installed versions of Cypress hat may be cached on your machine. Once you run this command, you will have to run cypress install before you run cypress install before you run Cypress again.
Debugging commands
Cypress is built with the debug module. This implies that you can receive helpful debugging output by running Cypress with debugging turned on prior to running cypress open or cypress run.
On Mac or Linux:
'''DEBUG=cypress:* cypress open'''
'''DEBUG=cypress:* cypress run'''
On Windows
'''set DEBUG=cypress:*'''
'''cypress run'''
Cypress is a very large and complex project that involves a dozen or more submodules, and the default output can be very overwhelming. If you want to filter the debug output to a specific module, you will need to run:
'''DEBUG=cypress:cli cypress run'''
'''DEBUG=cypress:launcher cypress run'''
Or even a third level deep submodule
'''DEBUG=cypress:server:project cypress run'''
History
The --quiet flag was added to cypress run in version 4.9.0
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics