Launching and Managing Browsers in Cypress for Testing
Whenever you run tests in Cypress, Cypress will launch a browser for you by default. This will enable you to:
- Create a clean, pristine testing environment.
- Access the privileged browser APIs for automation.
Browsers
When you run Cypress from the Test Runner, you can choose to run Cypress in a selected number of browsers, which include:
- Canary
- Chrome
- Chromium
- Edge
- Edge Beta
- Edge Canary
- Edge Dev
- Electron
- Firefox (Beta support)
- Firefox Developer Edition (Beta support)
- Firefox Nightly (Beta support)
By default, Cypress will automatically detect the available browsers on your OS. The browsers can be switched in the Test Runner by using the drop down in the top right corner:
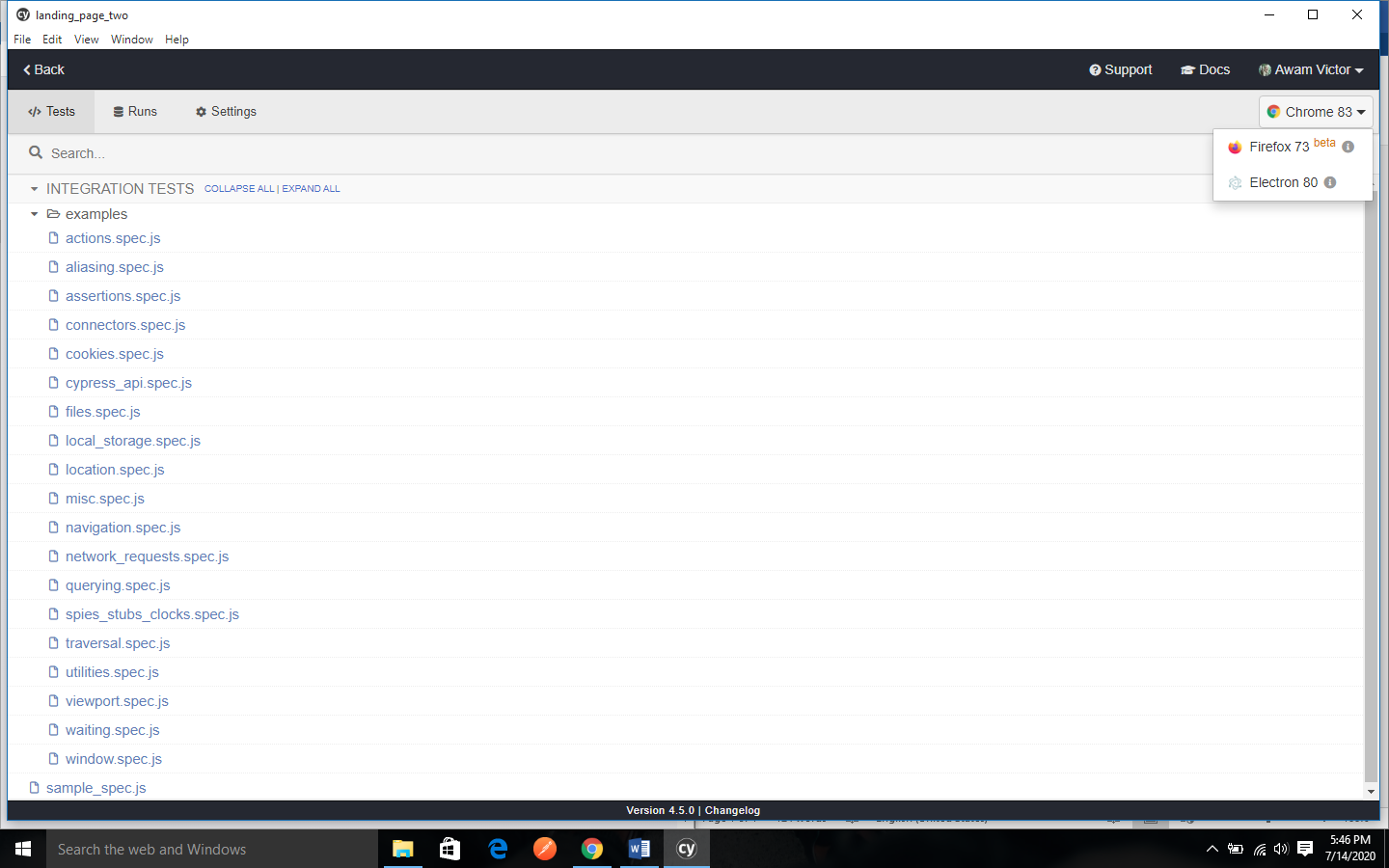
Download specific Chrome version
The chrome browser automatically updates itself. This can cause a breaking change in your automated tests. Links to where you can download a specific release version of Chrome for every platform (dev, Canary and stable) can be found here (https://chromium.cypress.io/).
Electron Browser
Aside the browsers that are found on your system, you will notice that Electron is listed as an available browser. This is because the Electron browser is a version of Chromium that comes with Electron.
The advantage of Electron is that it comes baked into Cypress and you don’t have to install it separately.
Cypress will launch Electron heedlessly by default, when you run cypress run.
To launch Electron headed, you will need to add the headed flag as shown below:
'''cypress run --headed'''
Since Electron is the default Cypress browser, it is typically run in CI. If you see failures in CI,
you can easily debug them by running locally with the –headed option.
Chrome Browsers
All Chrome flavored browsers are supported and will be detected.
To launch Chrome, you can run the command below:
'''cypress run --browser chrome'''
If you want to use the command above in CI, you will need to install the browser that you want- or you use one of Cypress docker images found here
(https://docs.cypress.io/examples/examples/docker.html)
Chrome launches in headed mode by default. If you want to run Chrome heedlessly, you will need to pass the –headless argument to cypress run.
To launch chromium, run cypress run --browser chromium.
To launch Chrome Canary, run cypress run --browser chrome:canary.
To launch Microsoft Edge (Chromium-based), you will need to run
'''cypress run --browser edge'''
and finally, if you want to launch Microsoft Edge Canary (Chromium-based): you will need to run:
'''cypress run --browser edge:canary'''
FireFox Browsers (beta)
The Firefox-family browsers have beta support.
To launch Firefox, run:
cypress run --browser firefox
To use Firefox Developer/Nightly Edition, you will need to run:
cypress run --browser firefox:dev
cypress run --browser firefox:nightly
If you want to use this command in CI, you will need to install these browsers. Firefox will launch in headed mode by default. However, you can pass the –headless argument to cypress run.
Launching by a path
You can launch any supported browser by specifying a path to the binary file. Here is an example:
cypress run --browser /usr/bin/chromium
# or
cypress open --browser /usr/bin/chromium
Customize available browsers
Sometimes you might want to modify the list of browsers that are found before running tests. For instance, you web app may only be designed to work in a Chrome browser and not in the Electron browser. From the plugins file, you can filter the list of browsers that are passed inside the config object and then return the list of browsers that you want to be available for selection during cypress open.
// cypress/plugins/index.js
'''module.exports = (on, config) => {
// inside the config.browsers array each object has information like
// {
// name: 'chrome',
// channel: 'canary',
// family: 'chromium',
// displayName: 'Canary',
// version: '80.0.3966.0',
// path:
// '/Applications/Canary.app/Contents/MacOS/Canary',
// majorVersion: 80
// }
return {
browsers: config.browsers.filter((b) => b.family === 'chromium')
}
}'''
If you open the Test runner in a project that uses the above modifications to your plugins file, it will only display the Chrome browsers found in the list of available browsers.
If installed a Chromium-based browser like Brave, Vivaldi, you can add them to the list of returned browsers. Below is a plugins file that inserts a local Brave browser into the returned list,
// cypress/plugins/index.js
'''const execa = require('execa')
const findBrowser = () => {
// the path is hard-coded for simplicity
const browserPath =
'/Applications/Brave Browser.app/Contents/MacOS/Brave Browser'
return execa(browserPath, ['--version']).then((result) => {
// STDOUT will be similar to "Brave Browser 77.0.69.135"
const [, version] = /Brave Browser (\d+\.\d+\.\d+\.\d+)/.exec(
result.stdout
)
const majorVersion = parseInt(version.split('.')[0])
return {
name: 'Brave',
channel: 'stable',
family: 'chromium',
displayName: 'Brave',
version,
path: browserPath,
majorVersion
}
})
}
module.exports = (on, config) => {
return findBrowser().then((browser) => {
return {
browsers: config.browsers.concat(browser)
}
})
}'''
Once it is selected, the Brave browser will be detected using the same approach as any other browser in the chromium family.
When you modify the list of browsers, you can see the resolved configuration in the Settings tab of the Test Runner.
Unsupported Browsers
Many browsers like Safari and Internet Explorer are not currently supported.
Browser Environment
Cypress will launch the browser in a way that is different from a regular browser environment. However, it will launch it in a way that Cypress believes will make testing more accessible and reliable.
Launching Browsers
Whenever Cypress goes to launch your browser, it will give you an opportunity to modify the arguments used to launch the browser.
This will enable you to do things like:
- Load your own extension
- Enable or disable experimental features
Cypress Profile
Cypress will generate its own isolated profile, aside your normal browser profile. This ensures that things like history entries, cookies, and third party extensions from your regular browsing session will not affect your tests in Cypress.
Disabled Barriers
Cypress will automatically disable certain functionality in the Cypress launched browser that tend to get in the way of automated testing.
The Cypress launched browser will automatically:
- Ignore certificate errors.
- Allow blocked pop-ups.
- Disable ‘Saving passwords’.
- Disable ‘Autofill forms and passwords’.
- Disable asking to become your primary browser.
- Disable device discovery notifications.
- Disable language translations.
- Disable restoring sessions.
- Disable background network traffic.
- Disable background and renderer throttling.
- Disable prompts requesting permission to use devices like cameras or mics
- Disable user gesture requirements for autoplaying videos.
Browser Icon
If you already have the browser open, you might notice that you have two of the same browser icons in your dock. This can make it difficult to tell the difference between your normal browser and Cypress.
To solve this problem, you can download and use a browser’s release version such as (Dev, Canary, etc). The icons of these browsers differ from the standard stable browser, thus, making them more distinguishable.