NumPy: numpy.arange() function
numpy.arange() function
The numpy.arange() function is used to generate an array with evenly spaced values within a specified interval. The function returns a one-dimensional array of type numpy.ndarray.
Syntax:
numpy.arange([start, ]stop, [step, ]dtype=None)
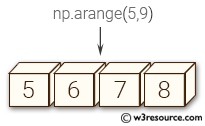
Parameters:
Name | Description | Required / Optional |
---|---|---|
start | The starting value of the interval. Default is 0. | Optional |
stop | The end value of the interval. The interval does not include this value. | Required |
step | The spacing between values. Default is 1. | Optional |
dtytpe | The desired data type of the output array. If not specified, the data type is inferred. | Optional |
Return value:
arange : ndarray containing evenly spaced values.
For floating-point arguments, the length of the result is ceil((stop - start)/step). Note that floating-point round-off can affect the length of the output.
Example: arange() function in NumPy.
>>> import numpy as np
>>> np.arange(5)
array([0, 1, 2, 3, 4])
>>> np.arange(5.0)
array([ 0., 1., 2., 3., 4.])
In the above example the first line of the code creates an array of integers from 0 to 4 using np.arange(5). The arange() function takes only one argument, which is the stop value, and defaults to start value 0 and step size of 1.
The second line of the code creates an array of floating-point numbers from 0.0 to 4.0 using np.arange(5.0). Here, 5.0 is provided as the stop value, indicating that the range should go up to (but not include) 5.0. Since floating-point numbers are used, the resulting array contains floating-point values.
Both arrays have the same length and contain evenly spaced values.
Visual Presentation:
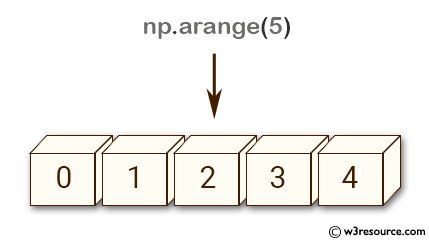
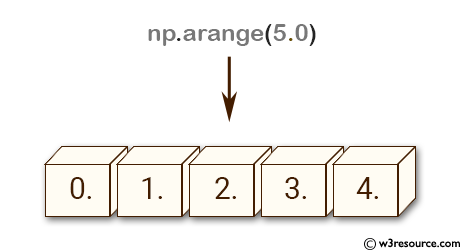
Example: Numpy arange function with start, stop, and step arguments
>>> import numpy as np
>>> np.arange(5,9)
array([5, 6, 7, 8])
>>> np.arange(5,9,3)
array([5, 8])
In the above code 'np.arange(5,9)' creates an array of integers from 5 to 8 (inclusive).
In the second example, 'np.arange(5,9,3)' creates an array of integers from 5 to 8 (inclusive) with a step size of 3. Since there is no integer between 5 and 8 that is evenly divisible by 3, the resulting array only contains two values, 5 and 8.
Frequently Asked Questions (FAQ): numpy.arrange Function
1. What is the purpose of the numpy.arange() function?
The numpy.arange() function generates an array of evenly spaced values within a specified interval.
2. What parameters does numpy.arange() accept?
numpy.arange() accepts the following parameters:
- start (optional): The starting value of the interval. Default is 0.
- stop (required): The end value of the interval, not included in the output array.
- step (optional): The spacing between values. Default is 1.
- dtype (optional): The desired data type of the output array.
3. Can I use numpy.arange() with floating-point numbers?
Yes, numpy.arange() can generate arrays with floating-point values by specifying floating-point start, stop, or step values.
4. How does numpy.arange() handle the step parameter?
The step parameter defines the spacing between consecutive values in the array. If not specified, it defaults to 1. If step is provided as a positional argument, the start value must also be specified.
5. What happens if I omit the start parameter?
If the start parameter is omitted, numpy.arange() defaults to starting the array at 0.
6. How does numpy.arange() determine the data type of the output array?
If the dtype parameter is not specified, numpy.arange() infers the data type from the other input arguments. You can specify a desired data type using the dtype parameter.
7. What is the difference between numpy.arange() and Python's built-in range()?
Unlike Python's range(), numpy.arange() returns a numpy.ndarray and supports generating arrays with floating-point values and specifying the data type.
8. Can numpy.arange() generate multi-dimensional arrays?
No, numpy.arange() generates only one-dimensional arrays. To create multi-dimensional arrays, you can reshape the result using numpy.reshape().
9. Why might the output of numpy.arange() not include the exact stop value?
The stop value is not included in the output array. For floating-point ranges, floating-point round-off can also affect whether the stop value appears to be included.
10. How can I create an array with a specific number of elements using numpy.arange()?
To create an array with a specific number of elements, adjust the start, stop, and step values such that the desired number of elements is generated. Alternatively, use numpy.linspace() for more direct control over the number of elements.
Python - NumPy Code Editor:
Previous: loadtxt()
Next: linspace()