NumPy: numpy.ascontiguousarray() function
numpy.ascontiguousarray() function
The numpy.ascontiguousarray() function is used to get a contiguous array in memory (C order). np.ascontiguousarray() function is useful when working with arrays that have a non-contiguous memory layout, as it can improve performance by ensuring that the data is stored in contiguous memory locations.
Syntax:
numpy.ascontiguousarray(a, dtype=None)
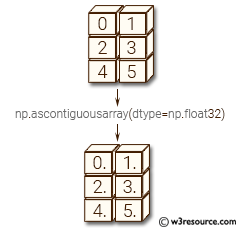
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input array. | Required |
dtype | Data-type of returned array. | optional |
Return value:
Contiguous array of same shape and content as a, with type dtype if specified.
Example: Creating a contiguous copy of an array with a specified data type
>>> import numpy as np
>>> a = np.arange(8). reshape (4, 2)
>>> np.ascontiguousarray(a, dtype=np.float32)
array([[ 0., 1.],
[ 2., 3.],
[ 4., 5.],
[ 6., 7.]], dtype=float32)
In the above example the second line of code creates a 2-dimensional numpy array a with shape (4, 2) using np.arange() function and reshaping the output to the specified shape.
Then np.ascontiguousarray() function is applied on 'a' with the specified data type np.float32. This function returns a contiguous array, which may be a new view of the original array if the original array was already contiguous with the same data type, or a new copy of the original array with the desired data type.
Visual Presentation:
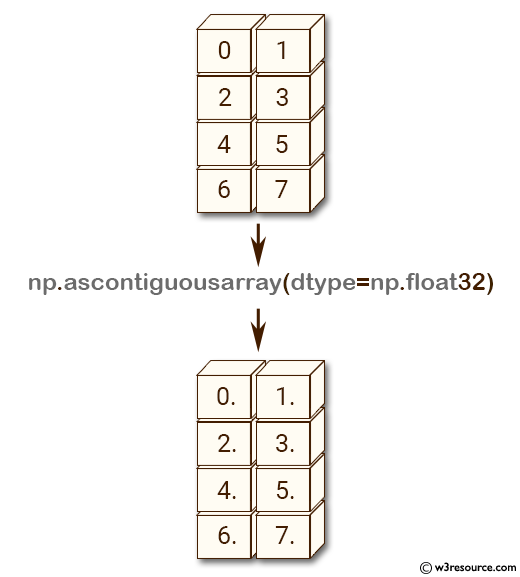
Example: Contiguous memory layout using np.ascontiguousarray()
>>> import numpy as np
>>> a = np.arange(8). reshape (4, 2)
>>> np.ascontiguousarray(a, dtype=np.float32)
array([[ 0., 1.],
[ 2., 3.],
[ 4., 5.],
[ 6., 7.]], dtype=float32)
>>> a.flags['C_CONTIGUOUS']
True
In the above code, a two-dimensional array a is created using the arange() and reshape() functions. Then, the np.ascontiguousarray() function is used to create a new array that has a contiguous memory layout. The dtype parameter is used to specify that the new array should have a data type of np.float32.
Frequently asked questions (FAQ) - numpy.ascontiguousarray ()
1. What is numpy.ascontiguousarray() used for?
numpy.ascontiguousarray() is used to create a contiguous array (a.k.a. C-style array) from a given array-like object.
2. What is a contiguous array?
A contiguous array is one where the elements are stored in adjacent memory locations, allowing for efficient access and manipulation.
3. When should we use numpy.ascontiguousarray()?
Use numpy.ascontiguousarray() when you need to ensure that the array's data is stored in contiguous memory, which is often required for certain operations or interactions with other libraries.
4. What happens if the input array is already contiguous?
If the input array is already contiguous, numpy.ascontiguousarray() returns the input array without making a copy, preserving memory efficiency.
5. Does numpy.ascontiguousarray() modify the input array?
No, numpy.ascontiguousarray() does not modify the input array. It returns a new array with the same data but guaranteed to be contiguous.
6. What are the benefits of using numpy.ascontiguousarray()?
- Ensures efficient memory access and compatibility with other libraries or functions that require contiguous arrays.
- Helps avoid potential performance penalties associated with non-contiguous memory layouts during array operations.
7. Are there any performance considerations when using numpy.ascontiguousarray()?
While numpy.ascontiguousarray() ensures contiguous memory layout, it may involve making a copy of the input array if it is not already contiguous, which could impact memory usage and performance in some cases.
Python - NumPy Code Editor:
Previous: asanyarray()
Next: asmatrix()