NumPy: numpy.asanyarray() function
numpy.asanyarray() function
The numpy.asanyarray() function is used to convert the input to an ndarray, but pass ndarray subclasses through.
Syntax:
numpy.asanyarray(a, dtype=None, order=None)
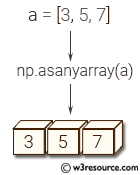
Parameters:
Name | Description | Required / Optional |
---|---|---|
a | Input data, in any form that can be converted to an array. This includes scalars, lists, lists of tuples, tuples, tuples of tuples, tuples of lists, and ndarrays. | Required |
dtype | By default, the data-type is inferred from the input data. | optional |
order | Whether to use row-major (C-style) or column-major (Fortran-style) memory representation. Defaults to 'C'. | optional |
Return value:
[ndarray]
Array interpretation of a. No copy is performed if the input is already an ndarray with matching dtype and order. If a is a subclass of ndarray, a base class ndarray is returned.
Example: Convert a list to a numpy array using asanyarray()
>>> import numpy as np
>>> a = [2, 4]
>>> np.asanyarray(a)
array([2, 4])
In the above code the input a is a Python list and not a NumPy array, asanyarray() creates a new NumPy array of dtype int64 by default to represent the elements of the input list. The resulting NumPy array can be used for further mathematical computations and operations.
Visual Presentation:
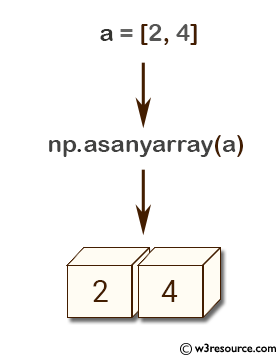
Example: np.asanyarray() with NumPy recarrays
>>> import numpy as np
>>> a = np.array([(2.0, 3), (3.0, 5)], dtype='f4,i4').view(np.recarray)
>>> np.asanyarray(a) is a
True
In the above code, first, a NumPy record array 'a' is created using the np.array() function and the view() method to convert a structured array to a record array. Then, the np.asanyarray() function is used to return 'a' as an array object. Finally, the code uses the 'is' operator to compare if the returned array object is the same as the original record array a. The code returns True indicating that the returned array object is the same as the original record array.
Frequently asked questions (FAQ) - numpy.asanyarray ()
1. What is numpy.asanyarray() used for?
numpy.asanyarray() is used to convert input to an ndarray but does not copy if the input is already an ndarray.
2. What is the difference between numpy.asarray() and numpy.asanyarray()?
numpy.asarray() always creates a new ndarray object from the input, whereas numpy.asanyarray() avoids copying the input ndarray if it's already an ndarray.
3. When should we use numpy.asanyarray()?
Use numpy.asanyarray() when you want to ensure the input is converted to an ndarray but prefer not to create a new ndarray object unnecessarily.
4. Does numpy.asanyarray() preserve the input type?
Yes, numpy.asanyarray() preserves the input type if the input is already an ndarray.
5. Does numpy.asanyarray() perform type casting?
numpy.asanyarray() may perform type casting if necessary to ensure the output is an ndarray, depending on the input type.
6. What happens if the input is not an ndarray?
If the input to numpy.asanyarray() is not already an ndarray, it will create a new ndarray object from the input data.
7. Is numpy.asanyarray() useful for handling different types of inputs?
Yes, numpy.asanyarray() is useful for handling a wide range of inputs, including lists, tuples, or other array-like objects that can be converted to ndarrays.
8. Does numpy.asanyarray() provide any performance benefits?
Using numpy.asanyarray() can sometimes provide performance benefits by avoiding unnecessary memory allocation and copying, especially when dealing with large datasets.
Python - NumPy Code Editor:
Previous: asarray()
Next: ascontiguousarray()