NumPy: numpy.array() function
numpy.array() function
The numpy.array() function is used to create an array. This function takes an iterable object as input and returns a new NumPy array with a specified data type (if provided) and shape.
The array() function is useful when working with data that can be converted into an array, such as a list of numbers. It is often used to create a NumPy array from an existing Python list or tuple.
Syntax:
numpy.array(object, dtype=None, copy=True, order=’K’, subok=False, ndmin=0)
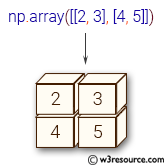
Parameters:
Name | Description | Required / Optional |
|||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
object | An array, any object exposing the array interface, an object whose __array__ method returns an array, or any (nested) sequence. | Required | |||||||||||||||
dtype | The desired data-type for the array. If not given, then the type will be determined as the minimum type required to hold the objects in the sequence. This argument can only be used to 'upcast' the array. For downcasting, use the .astype(t) method. | optional | |||||||||||||||
copy | If true (default), then the object is copied. Otherwise, a copy will only be made if __array__ returns a copy, if obj is a nested sequence, or if a copy is needed to satisfy any of the other requirements (dtype, order, etc.). | optional | |||||||||||||||
order | Specify the memory layout of the array. If object is not
an array, the newly created array will be in C order (row major) unless ‘F’ is specified, in
which case it will be in Fortran order (column major). If object is an array the following holds.
When copy=False and a copy is made for other reasons, the result is the same as if copy=True, with some exceptions for A, see the Notes section. The default order is ‘K’. |
optional | |||||||||||||||
subok | If True, then sub-classes will be passed-through, otherwise the returned array will be forced to be a base-class array (default). | optional | |||||||||||||||
ndmin | Specifies the minimum number of dimensions that the resulting array should have. Ones will be pre-pended to the shape as needed to meet this requirement | optional |
Return value:
An array object satisfying the specified requirements
Example: Creating Numpy Arrays
>>> import numpy as np
>>> np.array([2, 4, 6])
array([2, 4, 6])
>>> np.array([2, 4, 6.0])
array([ 2., 4., 6.])
>>> np.array([[2, 3], [4, 5]])
array([[2, 3],
[4, 5]])
In the above code the first line creates a one-dimensional numpy array of integers with values [2, 4, 6]. The second line creates a one-dimensional numpy array with mixed data types - integers 2 and 4, and float 6.0. When a numpy array has mixed data types, numpy will try to convert them to a single data type, in this case, float. The third line creates a two-dimensional numpy array of shape (2, 2) with values [[2, 3], [4, 5]].
Pictorial Presentation:
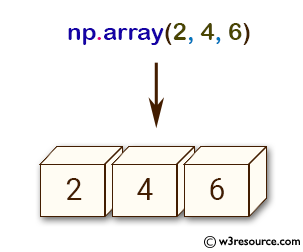
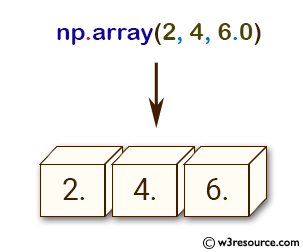
Example: Accessing structured array elements
>>> import numpy as np
>>> a = np.array([(2,4),(5,6)], dtype=[('x', '<i4'), ('y','i4')])
>>> a['y']
array([4, 6], dtype=int32)
>>> a['x']
array([2, 5], dtype=int32)
In the above example the first code, a 2x2 structured array is created with dtype=[('x', '<i4'), ('y','i4')]. The first element of the tuple specifies the column name, and the second element specifies the data type. In this case, the data type is a 32-bit integer (i4). Then, a['y'] is used to access the entire 'y' column of the structured array, and a['x'] is used to access the entire 'x' column. These operations return NumPy arrays with the selected columns.
Example: Creating NumPy Arrays from Matrix Objects
>>> import numpy as np
>>> np.array(np.mat('2, 4; 5, 6'))
array([[2, 4],
[5, 6]])
>>> np.array(np.mat('2 4; 6 8'))
array([[2, 4],
[6, 8]])
In the first code snippet, the np.mat() function creates a matrix object from comma-separated values '2, 4; 5, 6', which represents a 2x2 matrix. The np.array() function is then used to convert this matrix object into a NumPy array.
In the second code snippet, the np.mat() function creates a matrix object from space-separated values '2 4; 6 8', which also represents a 2x2 matrix. The np.array() function is used to convert this matrix object into a NumPy array as well.
Example: Creating a 4-Dimensional Array using ndmin parameter
>>> import numpy as np
>>> np.array([2, 4, 6], ndmin=4)
array([[[[2, 4, 6]]]])
In the above code creates a 4-dimensional numpy array by using the ndmin parameter of the np.array() function. The input array to np.array() is [2, 4, 6], which is a 1-dimensional array. Since we have set ndmin=4, the function will create a new array with at least 4 dimensions, padding the missing dimensions with size 1 if necessary.
Example: Converting matrices to arrays with subok parameter
>>> import numpy as np
>>> np.array(np.mat('2 4; 6 8'), subok=True )
matrix([[2, 4],
[6, 8]])
>>> np.array(np.mat('2 4; 6 8'), subok=False)
array([[2, 4],
[6, 8]])
The subok parameter in the np.array() function determines whether to create a new array object from the input or not. In the above code the first line creates a new array object from the input matrix with subok=True, while the second line creates a new array object without sub-classing the input matrix.
Frequently asked questions (FAQ) - numpy. array()
1. What is numpy.array()?
numpy.array() is a function in the NumPy library used to create arrays, which are central to numerical and scientific computing in Python.
2. What types of data can be converted into a NumPy array?
numpy.array() can convert lists, tuples, and other array-like sequences into NumPy arrays.
3. What are the key attributes of a NumPy array created using numpy.array()?
Key attributes include:
- ndarray.shape: The dimensions of the array.
- ndarray.size: The total number of elements in the array.
- ndarray.dtype: The data type of the elements in the array.
4. Can numpy.array() handle different data types?
Yes, numpy.array() can handle various data types, including integers, floats, and complex numbers. The desired data type can be specified using the dtype parameter.
5. What is the purpose of the dtype parameter in numpy.array()?
The dtype parameter allows you to specify the desired data type for the elements of the array.
6. How does numpy.array() handle multidimensional data?
numpy.array() can create multidimensional arrays by passing nested sequences (like lists of lists) as input.
7. What is the significance of the copy parameter in numpy.array()?
The copy parameter determines whether the function should copy the input data or use the original data directly. By default, it creates a copy of the data.
8. Can you control the memory layout of the array created by numpy.array()?
Yes, you can control the memory layout (row-major, column-major) using the order parameter.
9. How does numpy.array() handle array data that contains mixed types?
numpy.array() attempts to infer a common data type that can represent all elements, potentially upcasting to a more general type.
10. What are some common use cases for numpy.array()?
Common use cases include:
- Converting lists or tuples to arrays for numerical operations.
- Creating arrays for mathematical computations.
- Preparing data for machine learning and data analysis tasks.
11. How does numpy.array() differ from other array creation functions in NumPy?
numpy.array() is versatile for converting array-like sequences to NumPy arrays, whereas other functions like numpy.zeros(), numpy.ones(), or numpy.empty() are used for creating arrays with specific initial values or shapes.
Python - NumPy Code Editor:
Previous: full_like()
Next: asarray()
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/numpy/array-creation/array.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics